PubNub provides an MQTT gateway that allows you to use all the features of our Data Stream Network, like Functions and wildcards, with MQTT. This tutorial will show how to use the PubNub MQTT gateway to send and receive a ‘Hello World' message from a NodeMCU-32S development board with a built-in ESP32 WiFi Module.
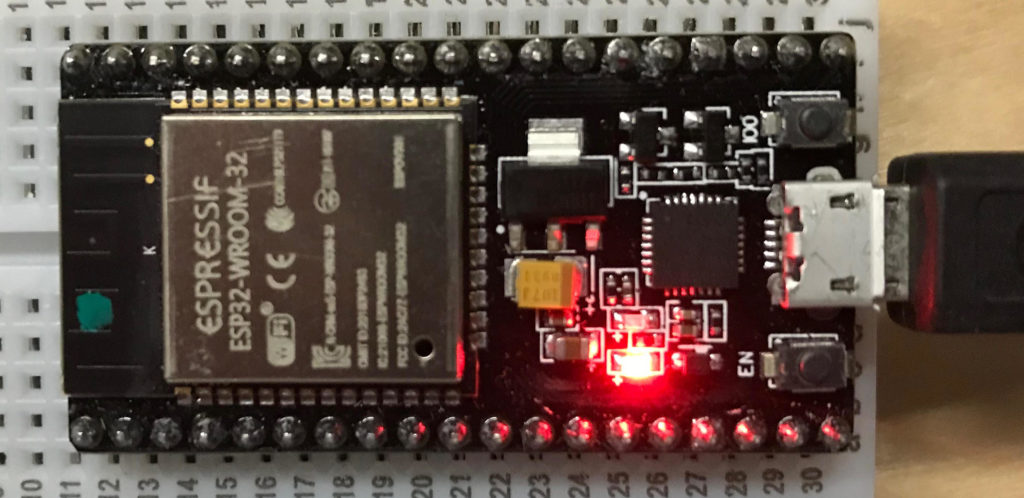
How does PubNub work with MQTT?
You can use PubNub as your broker with MQTT using any MQTT client or library that supports MQTT 3.1 or newer. MQTT protocol uses a publish-subscribe method to send data. Clients can publish messages to topics and subscribe to receive updates as they happen. MQTT requires an intermediary, called a broker (PubNub), to handle queuing and transmitting messages.
- PubNub maintains synchronized global Points of Presence (data centers) that can transfer data anywhere in the world (with an internet connection) in under 0.25 seconds with 99.999% SLA. You can trust that you'll get every message in mission-critical industrial IoT applications.
- PubNub offer features like mobile push notifications and Project EON that you can use for monitoring. If a sensor reading is out of range you can get a notification.
- PubNub offers a serverless environment to execute functions on messages as they route through the PubNub Network. You can incorporate logic like:
- Re-routing
- Forward to other channels.
- Augmenting
- Supplement with more information.
- Filtering
- Remove outliers or strip words.
- Aggregating data
- Combine data before it's received.
- Re-routing
Logitech uses PubNub for IoT applications.
Getting Started with Arduino and NodeMCU ESP-32S
First, download and install the latest Arduino IDE. Arduino 1.8.9 on macOS Mojave was used to make this tutorial.
Before you can use the NodeMCU ESP-32S with the Arduino IDE you'll need to add it with the Boards Manager.
Open the Arduino IDE.
Open the preferences window by going to File > Preferences.
In the “Additional Board Manager URLs” field add the following URL:
https://dl.espressif.com/dl/package_esp32_index.json
Navigate to the Board Manager by going to Tools > Boards > Boards Manager. Search for “esp32”. Install esp32.
Connect your NodeMCU-32S development board to your computer with a USB cable. The driver should install automatically if needed.
Select the port that your board is connected to under Tools > Port.
Select the board for use by going to Tools > Boards.
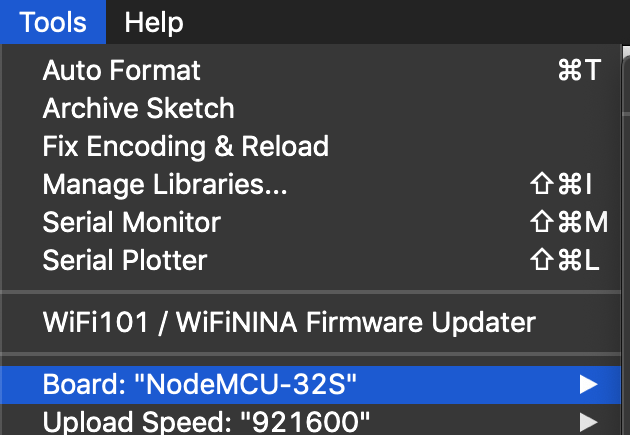
Install Arduino PubSub Client for MQTT
For this tutorial, we'll use PubSubClient as our MQTT library. There are also many other MQTT libraries you can pick from that offer a variety of features.
Install PubSubClient by going to Sketch > Include Library > Manage Libraries. Search for “PubSubClient”. Then select Install. You may need to scroll down to find it.
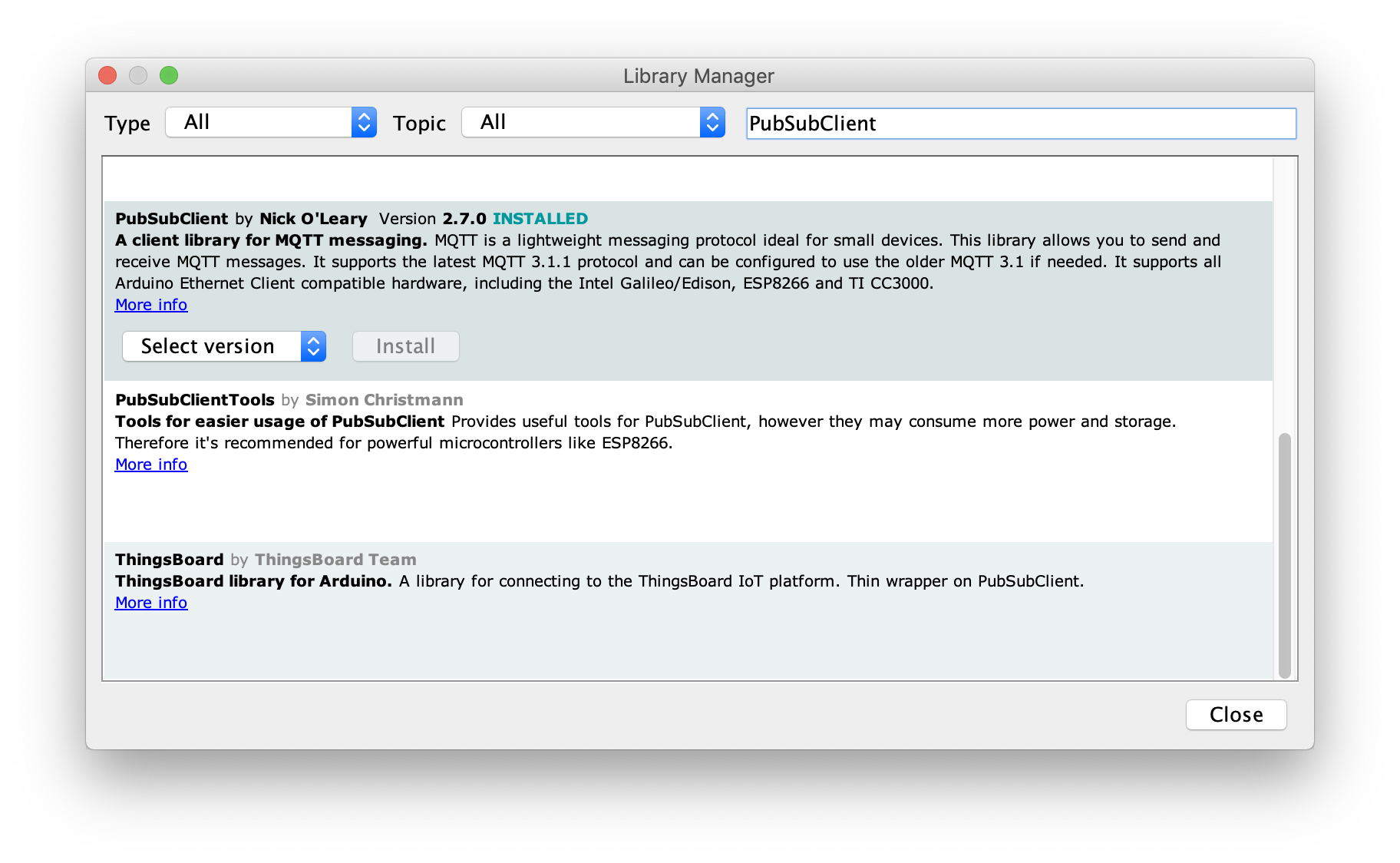
Uploading PubSub Sketch
Now we can flash the board with our sketches.
Next, sign up for a PubNub account. Once you sign up, you can get your unique PubNub keys from the PubNub Admin Dashboard.
Sign up using the form below:
The following code sends and receives “Hello World!” to a “hello_world” channel. Paste this code into the Arduino IDE.
// PubNub MQTT example using ESP32. #include <WiFi.h> #include <PubSubClient.h> // Connection info. const char* ssid = "YOUR_NETWORK_SSID"; const char* password = "YOUR_NETWORK_PASSWORD"; const char* mqttServer = "mqtt.pndsn.com"; const int mqttPort = 1883; const char* clientID = "YOUR_PUB_KEY_HERE/YOUR_SUB_KEY_HERE/CLIENT_ID"; const char* channelName = "hello_world"; WiFiClient MQTTclient; PubSubClient client(MQTTclient); void callback(char* topic, byte* payload, unsigned int length) { String payload_buff; for (int i=0;i<length;i++) { payload_buff = payload_buff+String((char)payload[i]); } Serial.println(payload_buff); // Print out messages. } long lastReconnectAttempt = 0; boolean reconnect() { if (client.connect(clientID)) { client.subscribe(channelName); // Subscribe to channel. } return client.connected(); } void setup() { Serial.begin(9600); Serial.println("Attempting to connect..."); WiFi.begin(ssid, password); // Connect to WiFi. if(WiFi.waitForConnectResult() != WL_CONNECTED) { Serial.println("Couldn't connect to WiFi."); while(1) delay(100); } client.setServer(mqttServer, mqttPort); // Connect to PubNub. client.setCallback(callback); lastReconnectAttempt = 0; } void loop() { if (!client.connected()) { long now = millis(); if (now - lastReconnectAttempt > 5000) { // Try to reconnect. lastReconnectAttempt = now; if (reconnect()) { // Attempt to reconnect. lastReconnectAttempt = 0; } } } else { // Connected. client.loop(); client.publish(channelName,"Hello world!"); // Publish message. delay(1000); } }
Get your unique PubNub keys from the PubNub Developer Portal. If you don’t have a PubNub account, you can sign up for a PubNub account for free. Replace “YOUR_PUB_KEY_HERE” and “YOUR_SUB_KEY_HERE” with your keys.
“CLIENT_ID” is the actual device ID and identifies the connection to PubNub. This should be unique to each client and you can set it to whatever you want.
Replace “YOUR_NETWORK_SSID” and “YOUR_NETWORK_PASSWORD” with your WiFi settings.
Check that you have selected the port that your board is connected to under Tools > Port. Select the board for use by going to Tools > Boards.
The upload speed is selected to ‘921600' by default. Lower it to ‘115200' to avoid a espcomm_sync failed
error when trying to upload the sketch at 921600 speed.
Hold-down the ‘BOOT' button in your ESP32 board.
Make sure that you have selected the proper port, set the upload speed to 115200 or lower, and set the board as ‘NodeMCU-32S'.
Upload the sketch (Sketch > Upload).
Release the ‘Boot' button when you see “Writing at …” and after seeing “Connecting…” in the console. You must press and hold the ‘BOOT' button before uploading and keep it pressed until the IDE starts writing.
After uploading, press the ‘Enable' button to restart the board and run the new sketch.
Open the serial monitor (Tools > Serial Monitor) to see messages being received.
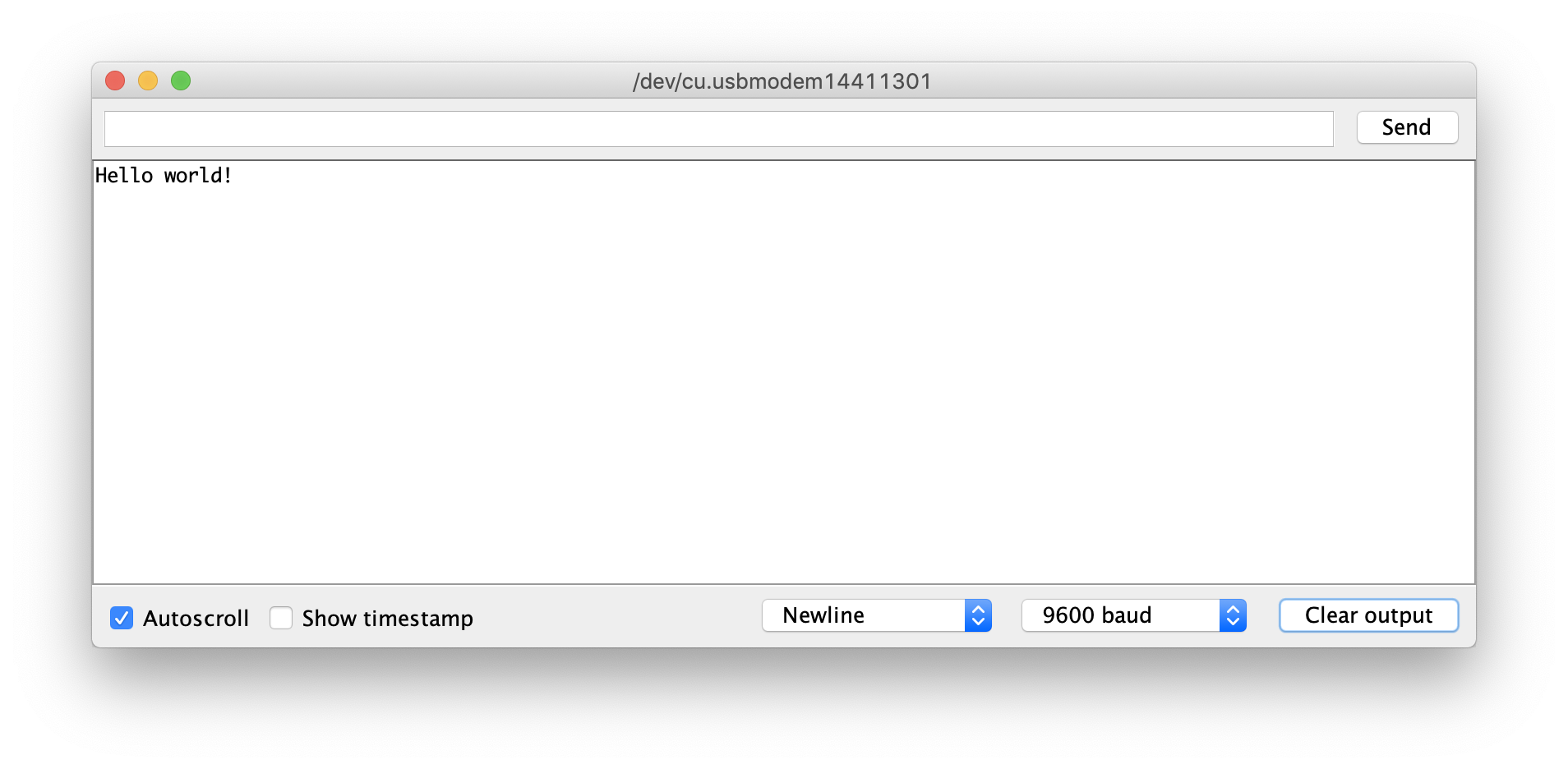
You're using the PubNub MQTT gateway! The messages you're publishing can be any data you want. Try connecting a sensor to an analog input pin and publishing the reading. Set up a second development board and you should be able to see messages transmitting between devices. You can also see messages being transmitted from the debug console found in the PubNub Admin Dashboard.
Next Steps for MQTT and NodeMCU ESP32
Now that you're familiar with using MQTT for publish-subscribe you should learn more about how PubNub can grow your IoT application. Start with our IoT Solution Overview. From there, learn about:
Other ways to expand your IoT projects with PubNub:
- Chat messaging applications.
- Real-time updates for GPS location.
- Dashboards for real-time monitoring.
- Live audience interaction for televised events.
Have suggestions or questions about the content of this post? Reach out at devrel@pubnub.com.