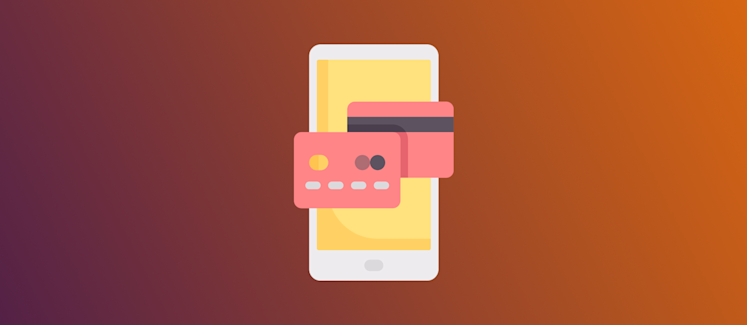
This tutorial shows how to create a Stripe charge using Functions. Use cases for this Function include selling products or services, restricting access to a web page, selling a file, and accepting donations.
What are Functions?
Functions is a serverless environment for executing functions. Functions are JavaScript event handlers that can be executed on in-transit PubNub messages or in the request/response style of a RESTful API over HTTPS. If you are familiar with Node.js and Express, the On Request event handler development will be second nature.
Here are a few things you could use Functions to do:
- Translation – Send a chat app message in English, and the recipient receives it in Spanish.
- Secure API Calls – Trigger an alert when a secure message meets certain criteria – email, text message, tweet, mobile push, etc.
- Network Logic – Tally up millions of votes or question answers during real-time audience interaction.
- Serve Data – Build a globally replicated, low latency, production REST API in 5 minutes.
Advantages of Functions:
- Reduced Cost: Pay for what you use and not for idle servers.
- Decreased Time to Market: Focus on building your product and not managing the infrastructure to support it.
- Reduced Latency: Your users are global. Your product should be global. PubNub operates points of presence across the globe.
Getting Started
Check out the Stripe Charge Block for a faster way to get started.
- You’ll need a Stripe account and API keys. You don’t need to activate your account unless you plan to go beyond testing. The test keys will work fine for this project.
- You’ll need to sign up for a PubNub account. Once you sign up, you can get your unique PubNub keys from the PubNub Admin Dashboard. Sign up using the form below.
Design Pattern
- A user enters their payment details and a preferred username into a frontend. The payment details are sent directly to Stripe using Stripe.js. Stripe.js then tokenizes the sensitive information so that it won’t be exposed when sent to a backend to initiate a charge.
- It is against PubNub Terms and Conditions to send credit card information including credit card numbers, CSV numbers (three-digit codes for Visa and MasterCard, four-digit code for American Express) and magnetic stripe information through the PubNub Network.
- The token is used by our Stripe Charge Function, hosted in Functions, to initiate a charge with Stripe.
- The charge is processed by Stripe and our Stripe Charge Function receives the result.
- The payment status is then forwarded to the frontend.
Setting up the Stripe Charge Function
Go to your PubNub Admin Dashboard and select your app. Then click on the keyset that was created for that app.
Next, click “Functions” in the sidebar and then create a new module named “Stripe”.
Create a function with the name “Stripe”, set the event type to “On Request”, and set the path to “stripe”.
Delete any code in the editor and replace it with a function to create a Stripe charge:
// DO NOT USE THIS CODE CLIENT SIDE. FOR A PUBNUB SERVERLESS ENV ONLY. // Set the module event type to "On Request". // Create a token with Stripe.JS: https://stripe.com/docs/stripe-js // Or use a test token: token=tok_visa export default (request, response) => { const vault = require("vault"); const xhr = require("xhr"); const token = request.params.token; return vault.get("stripe_secret_key").then((apiKey) => { const http_options = { "method": "POST", "headers": { "Authorization": "Bearer "+apiKey, 'Content-Type': 'application/x-www-form-urlencoded', }, "body": "amount=100¤cy=usd&source="+token, }; return xhr.fetch("https://api.stripe.com/v1/charges", http_options).then((resp) => { if (resp.status == 200) { // Record that the user has paid and do something. } response.status = resp.status; return response.send(resp); }); }); };
You’ll need to add your Stripe secret key to the PubNub vault. Click on “My Secrets” in the function editor and add a key named “stripe_secret_key” with your Stripe secret key.
Edit the query string to change the currency and charge amount.
How to Test the Stripe Charge Function
Use a Stripe test token to test the function.
Use Curl from your Terminal to send a test request to the function:
- Set the query parameter to “?token=tok_visa”
- Use POST to make the request.
- Replace “
YOUR_SUB_KEY_HERE
” with your Subscribe Key from your PubNub Admin Dashboard.
curl -X POST https://pubsub.pubnub.com/v1/blocks/sub-key/YOUR_SUB_KEY_HERE/stripe?token=tok_visa
How to Build a Frontend to Accept Stripe Charges
The easiest way to create card input form you can use with this function is by using Stripe Checkout.
What’s Next?
Here are a few ideas:
- Combine with a Slackbot to accept pizza orders.
- Build a smart home where guests have to pay to open your refrigerator.
- Send an email with a file download after a user has paid.
Have suggestions or questions about the content of this post? Reach out at devrel@pubnub.com.