Build a Real-time Voting or Polling App using Highcharts
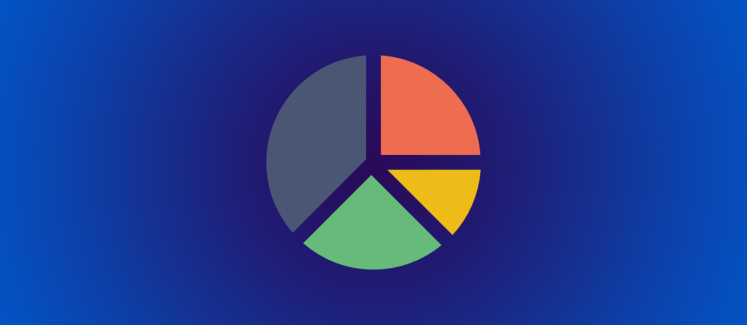
Charts are an effective and simple way to improve user experience in your application. Visualizing data is hard, and charts make it possible to understand complex sets of information at a glance. Even better, what if you could update those charts in real time, changing the visualization as new data came through?
You could create the charts yourself or embed them as images; however, using a library, such as Highcharts, to display data will give your application more visual flair and provide a better user experience without needing to build it yourself.
To showcase real-time, multi-user charts in action, we’ll be building an ice cream voting application using JavaScript, Highcharts, and PubNub. The objective of this tutorial is to demonstrate how you can use Highcharts with PubNub to visualize data.
Open up the demo in a couple of windows, you’ll see votes from one user reflected across the rest of the charts in real time.
What is Highcharts?
Highcharts is a library for charting written in pure JavaScript. It makes it easy to add interactive charts to your web and mobile projects. It’s used by over 80% of the world’s 500 largest companies, including Facebook, Twitter, and Verizon. Highcharts claims that it’s “the simplest yet most flexible charting API on the market.”
Some of the key reasons to use Highcharts include:
- Chart flexibility: Supports line, spline, area, area spline, column, bar, pie, scatter, angular gauges, area range, area spline range, column range, and polar chart.
- Robust documentation: Customize how data is displayed and fit it seamlessly into your app.
- Advanced responsiveness: Charts adapt to how your users view them and animate when new data points are added.
- Industry-leading accessibility support: Your users will still be able to access information when using non-visual methods.
- Extreme compatibility: Includes mobile, tablets and old IE back to IE6.
- Free for non-commercial: Use with a personal website, a school site or a non-profit organization with no barriers.
Why use PubNub with Highcharts?
PubNub’s primary product is a real-time publish/subscribe messaging API built on a global Data Stream Network. Messages sent with PubNub are delivered in under 0.25 seconds, and PubNub supports over 70 SDKs in most programming languages. PubNub provides the secure, scalable, and reliable infrastructure to power any real-time application.
Combined with Highcharts, you can visualize information sent with PubNub in real time. In this tutorial, you’ll see the pie chart update as votes are received. You could build a ton of other useful visualizations like:
- Real-time algorithmic trading and investing data.
- Performance statistics during a multiplayer game.
- Data outliers from your IoT device.
Voting App Tutorial
Start by creating three empty files in the same directory: ‘index.html’, ‘styles.css’, and ‘vote.js’.
First, populate index.html with a container for our chart, add three buttons for users to cast their votes, and include the Highcharts and PubNub JavaScript SDKs:
<!-- Vote Demo using PubNub JavaScript V4 SDK and HighCharts--> <!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <link rel="stylesheet" href="styles.css"> <title>Vote PubNub</title> </head> <body> <div class="page-container"> <div id="chart-container"></div> <div id="buttons"> <button onClick="publishVote('0')">Vote Chocolate</button> <button onClick="publishVote('1')">Vote Vanilla</button> <button onClick="publishVote('2')">Vote Strawberry</button> </div> <p><a href="https://www.pubnub.com/?devrel_pbpn=vote_pubnub"><img src="https://d2c805weuec6z7.cloudfront.net/Powered_By_PubNub.png?devrel_pbpn=vote_pubnub" alt="Powered By PubNub" width="150" class="center"></a><p> <script src="https://code.highcharts.com/highcharts.js"></script> <script src="https://cdn.pubnub.com/sdk/javascript/pubnub.4.23.0.min.js"></script> <script src="vote.js"></script> </div> </body> </html>
Next, style the buttons and the container for our chart. Edit syles.css and add the following:
body { text-align: center; } .page-container{ display: inline-block; } #chart-container { min-width: 200px; height: 250px; margin: 5 auto } #buttons { width: 100%; text-align: center; padding-top: 25px; padding-bottom: 15px; } button { height: 40px; width: 200px; text-align: center; font-size: 20px; color: #fff; line-height: 0; background: #d02129; border: 1px solid #d02127; margin: 5px; border-radius: 5px; }
Last, use the Highcharts API to build and style a pie chart. The pie chart will display a running count of our three vote options (flavors of ice cream).
Initialize PubNub and create a function that publishes a message when a vote button is pressed. Then add a listener to subscribe to updates. The callback will update the pie chart in real time when new votes are received.
Edit vote.js and add the following:
var chart = Highcharts.chart('chart-container', { // Build the chart. colors: ['#660000', '#DDAABB', '#D02129'], chart: { plotBackgroundColor: null, plotBorderWidth: null, plotShadow: false, type: 'pie' }, title: { text: 'What\'s your favorite ice cream?' }, tooltip: { pointFormat: '<b>{point.percentage:.1f}% - {point.y} Vote(s)</b>' }, plotOptions: { pie: { allowPointSelect: true, cursor: 'pointer', dataLabels: { enabled: false }, showInLegend: true } }, subtitle: { text: '* Refreshing page will reset chart.', floating: true, align: 'right', x: -10, verticalAlign: 'bottom', y: -75 }, series: [{ name: 'Flavors', colorByPoint: true, data: [{ name: 'Chocolate', y: 0 }, { name: 'Vanilla', y: 0 }, { name: 'Strawberry', y: 0 }] }] }); var voteChannel = "ice_cream_flavor_votes"; // Channel for counting votes. pubnub = new PubNub({ // Your PubNub keys here. Get them from https://admin.pubnub.com. publishKey : 'YOUR_PUBNUB_PUBLISH_KEY_HERE', subscribeKey : 'YOUR_PUBNUB_SUBSCRIBE_KEY_HERE' }); function publishVote(flavor) { // Publish a vote with PubNub. var publishConfig = { channel: voteChannel, message: flavor // Send the flavor of the vote. }; pubnub.publish(publishConfig, function(status, response) { // Publish message. console.log(status, response); }); }; pubnub.addListener({ message: function(vote) { var chartData = chart.series[0].data[vote.message]; chartData.update(chartData.y + 1); // Add vote. }, }); pubnub.subscribe({ channels: [voteChannel] // Listen for votes. });
Sign up for a free PubNub account. Once you sign up, you can get your unique PubNub keys from the PubNub Admin Dashboard. PubNub is always free, up to 1 million real-time transactions per month.
Sign up using the form below:
Replace “YOUR_PUBNUB_PUBLISH_KEY_HERE” and “YOUR_PUBNUB_SUBSCRIBE_KEY_HERE” with your Publish Key and Subscribe Key from your PubNub Admin Dashboard.
Open index.html in your browser. You’ll be able to see your voting application and cast votes!
Deploy the files to a server or share the files, and you can have other people cast their votes.
What’s Next?
Want to take this project further? Here are a few ideas:
- Use Storage & Playback to keep a history of votes after the page is refreshed.
- Create real-time trading dashboards.
- Expand this project by using it for live audience interaction for classrooms and televised events.
- Win America’s Got Talent
Have suggestions or questions about the content of this post? Reach out at devrel@pubnub.com.