Build a Java Chat App For Android: Getting Started (1/4)
Welcome to our 4-part tutorial on building a feature-rich mobile chat application for Android with PubNub and Java, providing APIs and infrastructure for building real-time applications.
Android Java Chat Tutorial Overview
By the end of this tutorial series, you’ll have a fully-functional Android chat application powered by PubNub, written in Java. You can navigate between parts with the links below.
- Part One: Getting Started and Setup
- Part Two: Sending and Receiving Messages, Message Persistence
- Part Three: Real-time User List With Presence
- Part Four: Scaling and Channel Groups
The purpose of this tutorial series is to show how easy it is to build real-time Android applications using PubNub and our Java SDK. Why? We think the Android platform is awesome because it gives developers the power to release applications across a huge range of devices.
Why PubNub and Android?
PubNub is a global real-time data stream network that allows you to create applications that scale from one user to hundreds of millions of users, seamlessly. You may have previously heard of technologies like WebSocket and Socket.io. PubNub takes the essence of these technologies, adds dozens of value-added features, and deploys it across a global network that enables message delivery within just a tenth of a second. That’s 100ms, much of which is taken up by the last hop from the mobile data carrier to the device itself.
Can developers build real-time applications without PubNub? Yes, but they also then have to manage a secure and highly reliable global network that scales to massive audience sizes, not to mention creating dozens of SDKs to provide a unified API and connectivity to the full range of devices on the network. Might as well leave it to PubNub.
There are a few reasons why PubNub and Android go so well together. The rise of enterprise communication services like Slack, ride-hailing services like Uber and Lyft, as well as the growth of IoT (Internet of Things) applications means that user expectations are moving rapidly towards a continuously-updated, “real-time” model of applications.
This means that users don’t want to click a “refresh button” or “pull down to refresh”, they want to see changes in the application as soon as they happen. Similarly, daily data updates just don’t cut it anymore – stakeholders now want to see information with low latency as soon as it becomes available. A static GIF of a map, graph or pie chart is a huge fail – it needs to be a dynamically updated SVG showing a timestamp of “less than 5 seconds ago.”
What We're Building and Tools Used
Here's an example of what we'll build:
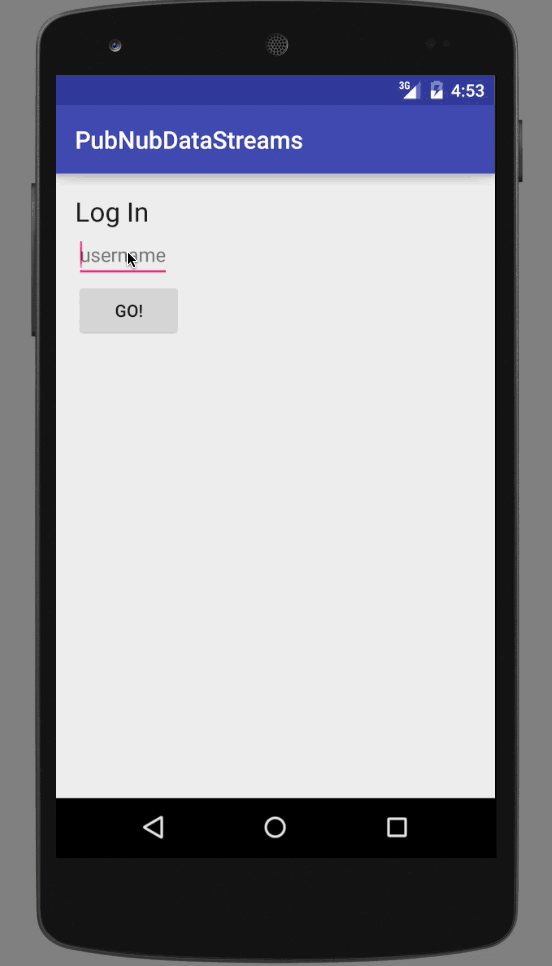
The app uses Publish/Subscribe messaging for a real-time chat feature, Presence tracking for a buddy list, and Multiplexing to show the last message across a group of channels. In this case, the Login activity is just a dummy one to collect the username.
Real-time Messaging
Real-time Messaging is the core of any real-time application. It allows devices to subscribe to named “channels.” When messages are published, they are delivered instantly to all connected devices. Publish/Subscribe data streams can transfer device positions, chat messages, or just about any kind of dynamic structured data you can think of; think JSON.
Presence
Presence is online/offline detection. If you’re familiar with chat applications, you’ve seen the friendly “buddy list” before. PubNub generalizes this concept across all the applications of Publish/Subscribe messaging, making it easy to see which devices are online and connected to channels using its Presence API. As devices come online, leave or become disconnected from the network, PubNub sends the corresponding Presence events of join, leave, and timeout to your application, allowing it to respond instantly to dynamic channel membership.
Multiplexing and Channel Groups
Multiplexing and Channel Groups allows developers the ability to create an unlimited number of channels. Using one connection for each channel subscription would quickly become unwieldy. Similarly, it’s often useful to give a group of channels a “nickname” to allow for easier subscription. PubNub provides Multiplexing to allow one device connection to support dozens of channel subscriptions. For cases where it’s necessary to subscribe to hundreds or thousands of channels, PubNub provides a feature called Channel Groups to create a compound subscription.
PubNub Android API
PubNub plays well with Android because the PubNub Android SDK (part of the PubNub Java SDK family) is extremely robust and has been battle-tested over the years across a huge number of mobile and backend installations. The SDK is currently on its 4th major release, which features a number of improvements such as fluent API, increased performance, sync/async operation, server-side filtering, streamlined logging & debugging, and more.
The PubNub Java SDK is distributed via the Maven Central repository, so it’s easy to integrate with your application whether you’re using Maven or Gradle.
Getting Started with PubNub
You’ll need unique publish/subscribe keys. First, go to the signup form on the PubNub website.
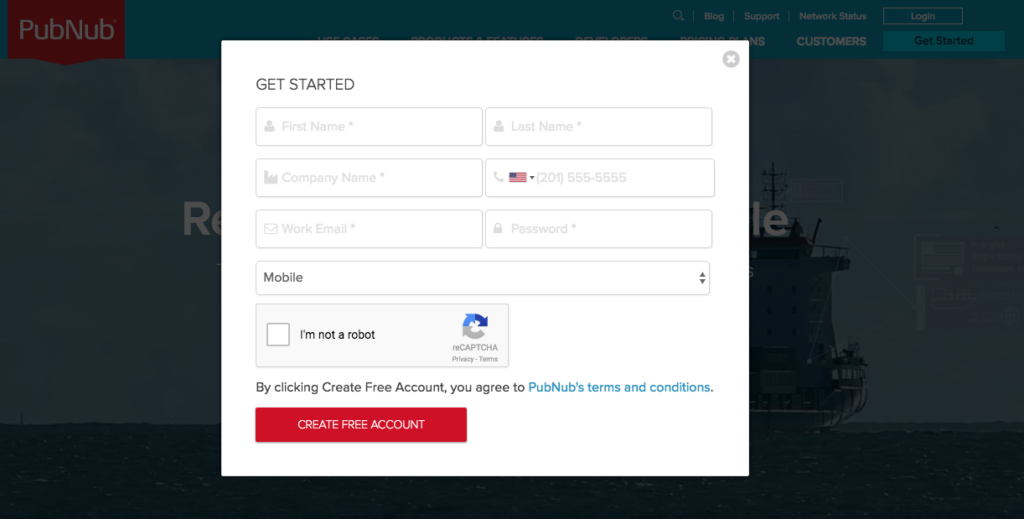
Create a new application, including publish and subscribe keys.
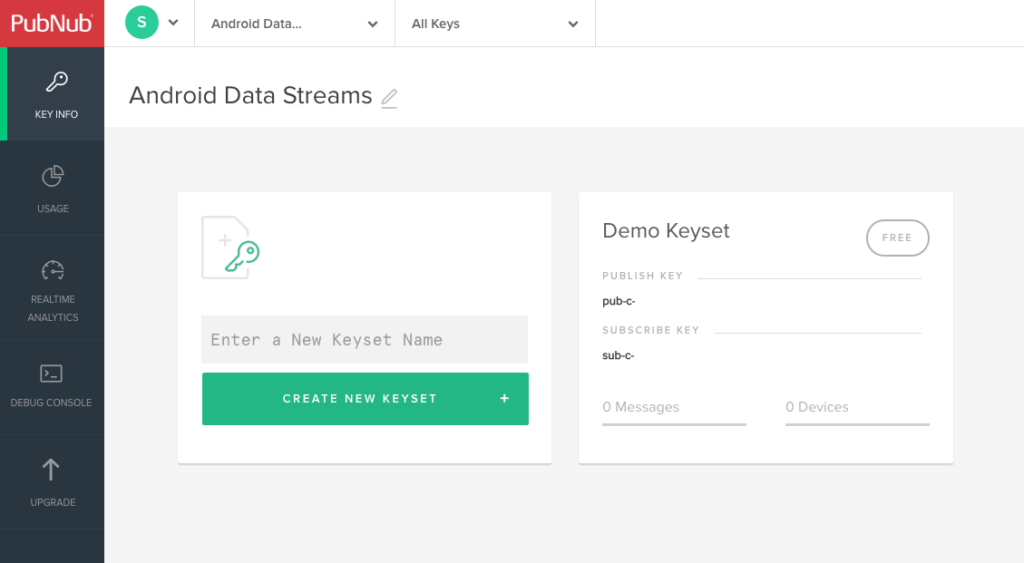
The publish and subscribe keys look like UUIDs and start with “pub-c-” and “sub-c-” prefixes respectively. Keep these handy – you’ll need to plug them in when initializing the PubNub object in your Android application.
Getting Started with Android Studio
If you’re building an Android app, chances are you’re using Android Studio. Don’t worry – it’s ok if you use Eclipse, EMACS or Vim, for that matter! We just include the initial steps here so you will get the rough idea.
Starting a new project is the same as you always would:
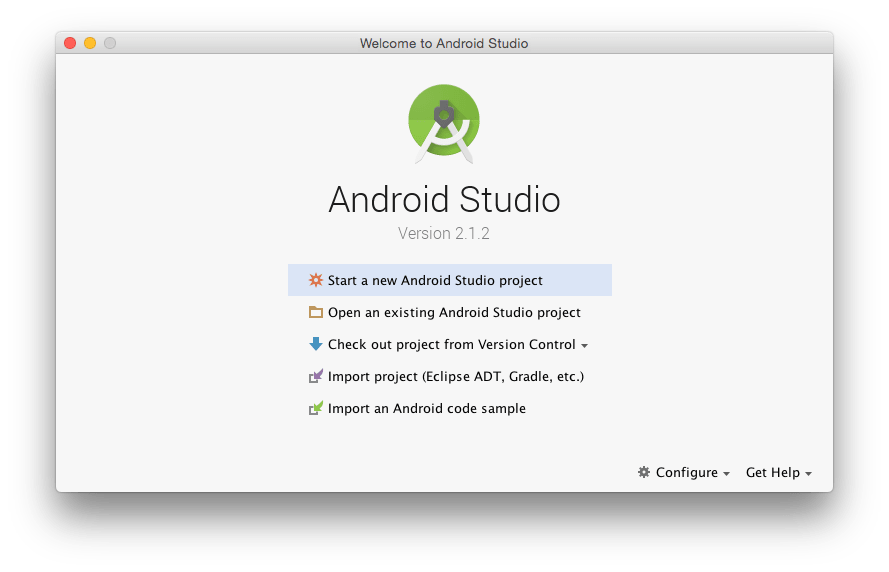
Then, you’ll want to add a PubNub dependency line to gradle:
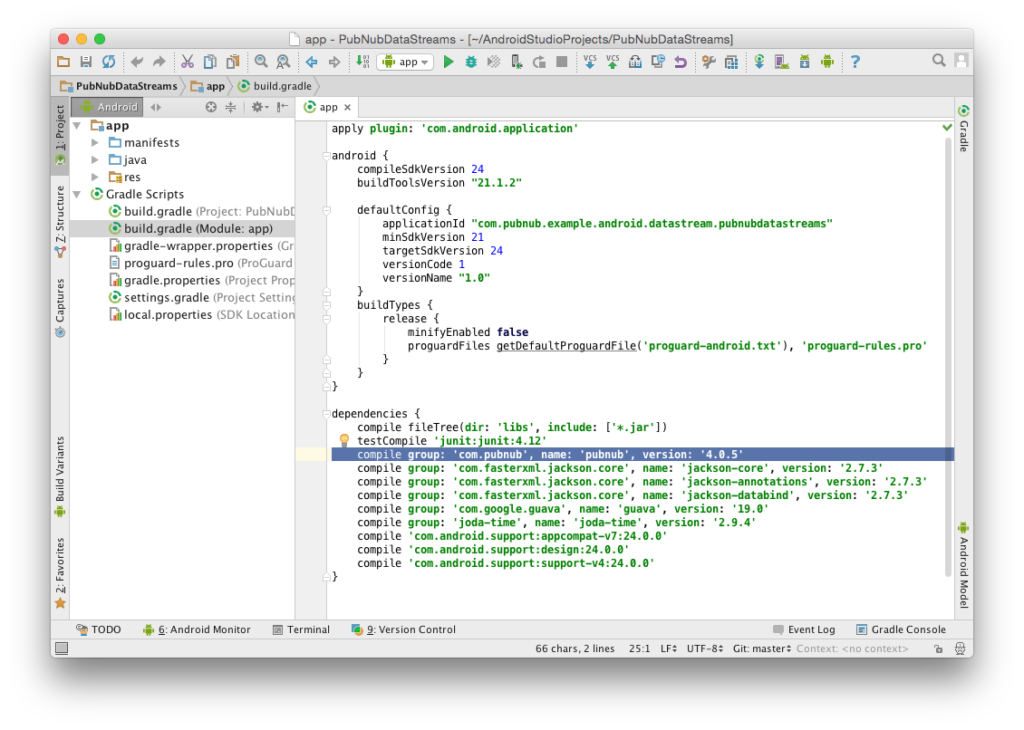
Here’s the dependency line up close:
compile group: 'com.pubnub', name: 'pubnub', version: '4.0.5'
Be sure to check the PubNub developer site frequently so you don’t miss a new version update!
Or, if you’d like to jump into the sample app right away, you can download it from GitHub.
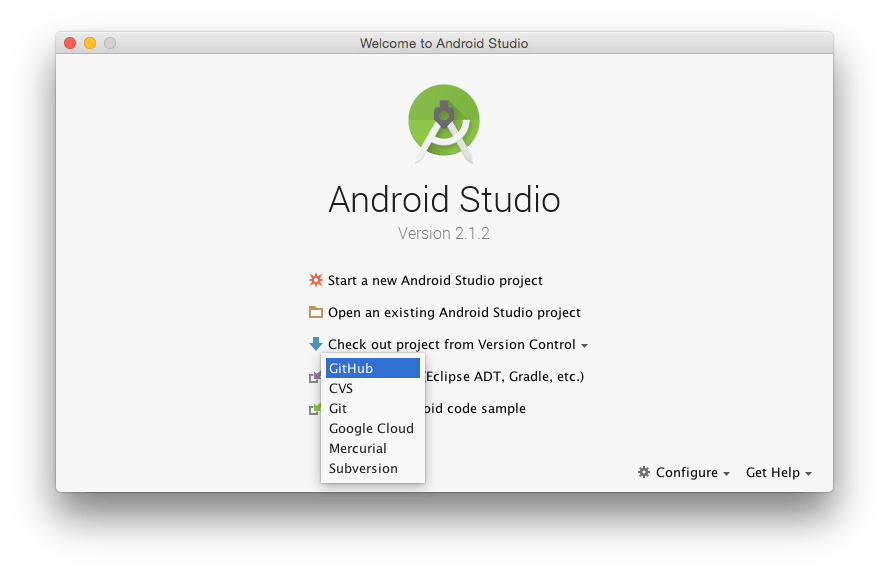
If you use the “clone project from GitHub” feature in Android Studio, the Git url for the sample app is:
https://github.com/sunnygleason/pubnub-android-data-streams-tutorial.git
Navigating the Sample Application
Once you’ve set up the sample application, you’ll want to update the publish and subscribe keys in the Constants class. These are the keys you created when you made a new account and PubNub application in previous steps. Make sure to update these keys, or the app won’t work!
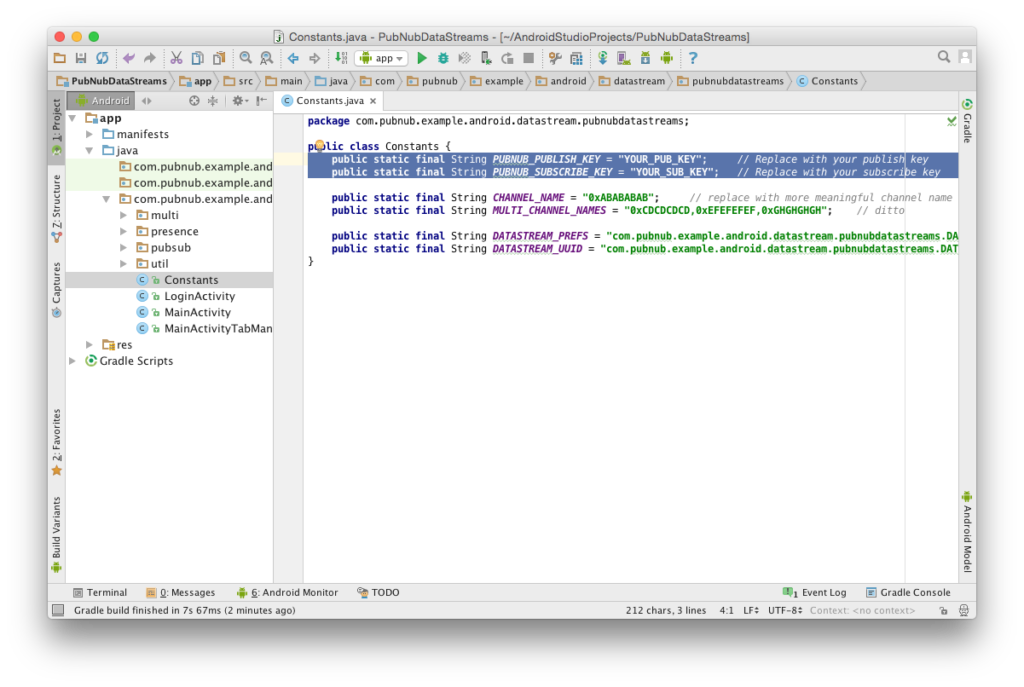
As with any Android app, there are 2 main portions of the project – the Android code (written in Java), and the resource files (written in XML).
The Java code contains 2 Activities, plus packages for each major feature: Real-time Messaging, Presence, and Multiplexing.
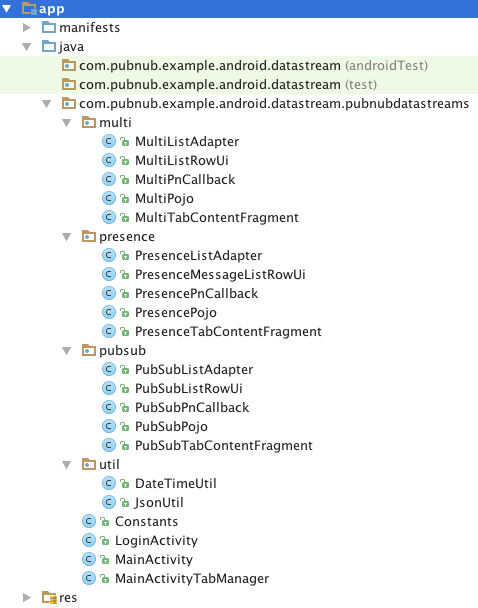
The resource XML files include layouts for each activity, fragments for the 3 tabs, list row layouts for each data type, and a menu definition with a single option for “logout.”
Whatever you need to do to modify this app, chances are you’ll just need to tweak some Java code or resources. In rare cases, you might add some additional dependencies in the build.gradle
file, or modify permissions or behavior in the AndroidManifest.xml
.
Running the Code
Running the code should be as easy as clicking the play button in the Android Studio toolbar. You can use a connected device or an AVD in the emulator to run the application.
As you sign into the application from multiple devices, you can exchange chat messages in the Pub/Sub tab, the Presence tab will show an updated member list, and the Multi tab will show the latest message sent by each device to a random channel.
Here’s what you’ll find:
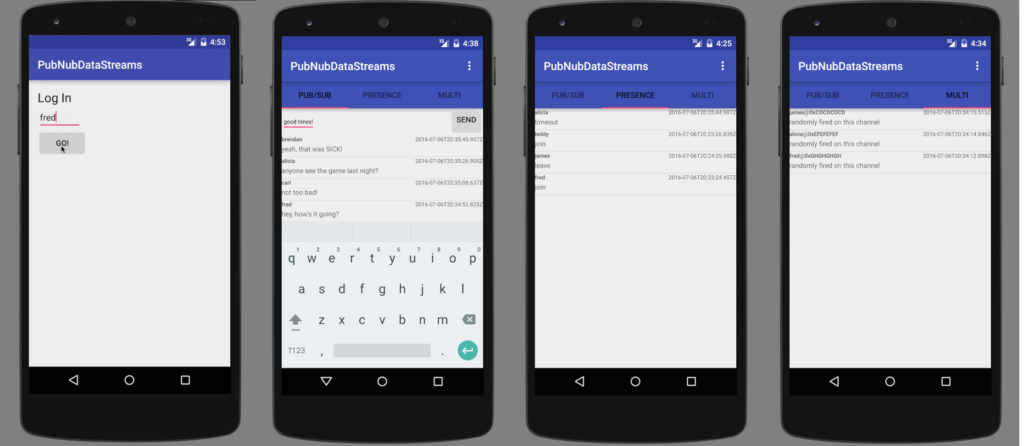
Next Steps
Now that we're all set up, it's time to start building chat. In our next part, we'll start sending and receiving messages, and a system for storing historic messages.