How to Add a Friend List to Your Unity Game
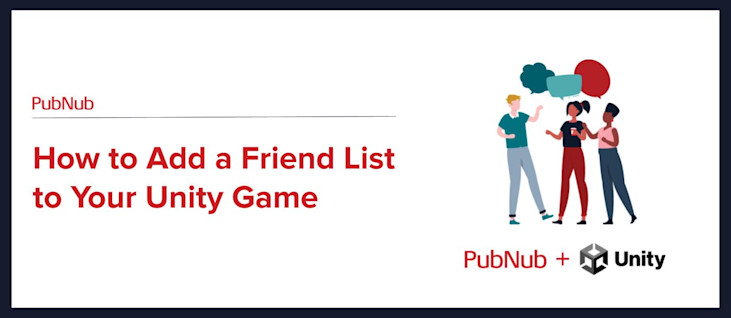
Incorporating a friend list in your Unity game is a key aspect of promoting player engagement. It allows your players to create a closer connection with other players in-game, whether they are friends outside of the game or if they formed a close bond during an intense match.
While friend lists are a vital feature in creating an engaging community, incorporating these features in your Unity project is easier said than done. To start from scratch, it takes a lot of resources to build, maintain, and scale when your players increase. Luckily, PubNub has made it easier than ever to inject real-time functionalities into Unity games with our real-time, low-latency API platform. We take care of the infrastructure layer of your apps so that you can focus on your application. Whether you're developing for Windows, Mac, iOS, Android, Virtual Reality systems such as Oculus and Meta Quest, or going cross-platform, our Unity SDK has you covered.
If you would like to see an example of how to implement a friend list in a Unity game to use as a reference when following along with this guide, be sure to check out our Unity Showcase Game.
Continue reading to learn step by step how to add a friend list to your Unity game to add, remove, and see friends’ online statuses in real time. While this guide discusses adding a friend list, the same architecture can easily apply to guild, clan, and alliance lists. You’ll start by understanding how to configure the PubNub GameObject, how Channel Groups architect friend lists, and finally learn about implementing a friending system.
Getting Started with PubNub
Before you begin to understand how to set up a scoreboard and leaderboard for your Unity Game, you’ll need to understand PubNub and how to configure your application to take advantage of the platform’s features.
Overview
PubNub is based on the Pub/Sub (Publish/Subscribe) model. A user will publish a message, which is essentially a payload that contains all relevant information, to the PubNub network. Users who want to receive or listen to the message and other generated events will subscribe to the PubNub network and parse the message. Event listeners are used to catch messages and events generated in the PubNub Network and trigger based on an action taken place.
To ensure the message gets to the right recipients, channels are used as the mechanism through which the data is transmitted from one device to another. Channels are required each time a device wants to publish and subscribe to the PubNub network. While a user can only publish one message at a time, a user can subscribe to many different channels at a time.
Install and Configure the PubNub Unity SDK
To start, you'll need to install any PubNub dependencies and configure the PubNub Unity SDK to connect your application to the PubNub network. This guide already assumes you have the Unity Game Engine, Unity Assets, and code editors such as Visual Studio installed. Please refer to the Unity SDK documentation for full details, but as an overview, you will need to:
- Add the Unity package through the Package Manager.
- Create a free PubNub account and obtain your PubNub Keys. You’ll need to enable the features on your keyset that your application needs. For this guide, you’ll need to enable Presence and Stream Controller with the default settings. Learn more details about the different features for your keyset by following our guides.
- Create a new Unity Project or open your existing game and provide Unity with the publish and subscribe keys you obtained in the previous step to configure the PubNub GameObject in the Unity Editor. You should also provide a UserId, as every PubNub object requires a unique identifier to establish a connection to PubNub. Once you have done so, initialize the PubNub object:
1 2 3 4
using PubnubApi; using PubnubApi.Unity; … PubNub pubnub = new Pubnub(pnConfiguration);
- Add an event listener for your game to react to messages, where your back end will essentially receive notifications about when you receive new messages. There are different event listeners to be able to implement custom logic to respond to each type of message or event, but for this guide, you’ll need the Presence and Message Event Listeners:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
var listener = new SubscribeCallbackListener(); pubnub.AddListener(listener); listener.onPresence += OnPnPresence; listener.onMessage += OnPnMessage; … // Handle Presence Events generated by the PubNub Network private void OnPnPresence(Pubnub pn, PNPresenceEventResult result) { Debug.Log(result.Event); } // Handle Message Events generated by the PubNub Network. private void OnPnMessage(Pubnub pn, PNMessageResult<object> result) { Debug.Log($"Message received: {result.Message}"); }
Presence is used to determine the online and custom status of your players in your game, where PubNub generates events to deliver information for their status in real time. You can learn more about implementing presence to your Unity game with our how-to guide.
Architecting Friend Lists
You’ll begin by subscribing to channels in order to receive Presence Events, which are essentially notifications for your game to know whenever a player’s friend comes online. But how do players know which players are their friends? Channel Groups are a perfect solution for this problem.
Channel Groups
Channel Groups allows you to manage a large, persistent number of channels at once. Channel Groups are essentially a pointer to a list of channels that allow you to send a single subscribe call for up to 2000 channels per Channel Group. Channel Groups are unique to the User ID that is connected to the network, meaning you can name a channel group uniquely and be tied to that player.
This means that instead of needing to subscribe to unique channels per user, you can subscribe to a Channel Group and receive Presence and other events anytime a player’s online or custom status changes. You can then think of Channel Groups and channels being the lists (Channel Group) of friends and members (channels) that you can manage and receive updates.
Setting up Channel Groups
Depending on the needs of your game, you’ll need at least two channel groups to implement a friend list for each player, each concatenated with the player’s User ID to be uniquely identified. Please note that the names can be different, so be sure to follow our channel naming conventions documentation:
1 2 3 4 5
//receive presence updates, so you know when a player’s friends online or custom status changes. string chanGroupFriendsStatus = $“friends_status_” + {UserID} ; // receive chat messages from friends string chanGroupFriendsChat = $”friends_chat_” + {UserID};
You need two channel groups because you want to isolate Presence Events from other events, such as chat messages. Having the same Channel Group represent both messages and channels can cause a lot of unnecessary traffic. You want to isolate events as much as possible to allow for more flexibility for your game to be expanded with more features in the future.
If you do not care about implementing friend chat, then you simply need the status channel group. If you do want to implement friend chat, please keep in mind that this guide is going to follow the architecture of friend lists, not setting up the chat system for your game. Please take a look at our real-time chat guide for more information on implementing a chat system in Unity.
Every Channel Group needs at least one channel associated with it. You can solve this by creating a channel pattern for all players and concatenating the player’s User ID to the channel names, such as:
1 2
string chanFriendStatus = $”friends.presence.” + {UserID}; string chanFriendChat = $”friends.chat.” + {UserID};
And then adding these two channels to the appropriate Channel Group using the Channel Groups API. You would do this for each player logging in for the first time:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
PNResult<PNChannelGroupsAddChannelResult> statusFriendsResponse= await pubnub.AddChannelsToChannelGroup() .ChannelGroup(chanGroupFriendsStatus ) .Channels(new string[] { chanFriendStatus }) .ExecuteAsync(); //Handle response … PNResult<PNChannelGroupsAddChannelResult> chatFriendsResponse= await pubnub.AddChannelsToChannelGroup() .ChannelGroup(chanGroupFriendsChat) .Channels(new string[] { chanFriendChat }) .ExecuteAsync(); //Handle response
This way of adding these named channels to the respective channel group is exactly how you would add and remove friends from a player’s friend list, but more on that later.
Listen for Presence and Message Events: Subscribe
With your channels and Channel Group system in place, you can now subscribe to the PubNub Network, which generates Presence Events whenever a player’s status changes and effectively comes online.
Similar to subscribing to channels, you can subscribe to Channel Groups directly and receive both Presence and Message Events from any of the channels associated with that Channel Group. Typically when subscribing, we can include the withPresence parameter to have the SDK handle managing both the Presence and non-Presence channels (more details on what this means in our Presence How-To Guide for Unity). However, we do not want to do that for our chanGroupFriendsStatus Channel Group, as we only want the Presence Events to be caught and not any other events, such as messages, metadata updates, etc, for that channel.
To remedy this situation, you can append -pnpres to the chanGroupFriendsStatus. This means that any events for this Channel Group, specifically Presence Events, will be caught by the Presence Event Listener we set up earlier. Furthermore, the chanGroupFriendsChat will not be listening for any Presence Events and only focus on Messages:
1 2 3 4 5 6
pubnub.Subscribe<string>() .ChannelGroups(new string[] { chanGroupFriendsStatus + “-pnpres”, chanGroupFriendsChat }) .Execute();
Send a Message: Publish
While your Channel Group chanGroupFriendsStatus ready to go, you need to set up a publish call for a friend to be able to send a message and have every friend associated with their friend chat Channel Group receive the message.
While you can subscribe to Channel Groups, you cannot publish to them. Instead, you will need to publish the message to a channel that is associated with the Channel Group you set up earlier:
1 2 3 4 5 6
PNResult<PNPublishResult> publishResponse = await pubnub.Publish() .Message(“Hey friends, let’s game?”) .Channel(chanFriendChat) .ExecuteAsync(); PNPublishResult publishResult = publishResponse.Result; //Handle result
Although you cannot publish to Channel Groups directly, when messages are published to any of the channels in this channel group, they will be received by the message handler of the client's listener. This means that any friends associated with the Channel Group will receive the message.
Initial Game Load
When players initially login, you need to call the List Channels service to list all the channels, or friends, of the Channel Group:
1 2 3
PNResult<PNChannelGroupsAllChannelsResult> friendsListResult= await pubnub.ListChannelsForChannelGroup() .ChannelGroup(chanGroupFriendsStatus) .ExecuteAsync();
This list of friends can then be used to construct the player’s Friend List UI since you can extract the User IDs from these channels (since you created the pattern yourself). If there is metadata related to these players, you can then use the User IDs with the App Context API to obtain metadata for their profile information, such as nickname, profile picture, notes about them, and forth.
To determine their current online status, make a HereNow call to retrieve every user that is currently online, and cross-reference with the player’s friend list:
1 2 3 4 5
pubnub.HereNow() .ChannelGroups(new String[]{ chanGroupFriendsStatus }) .IncludeState(true) .IncludeUUIDs(true) .ExecuteAsync()
You can then update your Friend List UI’s online status indicator to show that these friends are online. The Presence Event Listener that we set up earlier will detect any online and custom status changes, and you can update the player’s Friend List UI accordingly. As mentioned earlier, messages are also caught in the Message Event Listener and you can display them in a unique color that represents they were sent from the friend group.
You’ve got nearly everything implemented for adding a Friend List into your system, but how do players manage their friends list?
Managing Friends: Friending System
As we showed earlier, to add friends to a Channel Group, you can call the Add Channels function to add channels to a Channel Group. This can be initiated when a player clicks on a button to add another friend or accepts an invitation from another player.
Players can also remove friends from the friend group by calling the Remove Channels function and specifying the Channel Group and Channels. You’ll need to repeat this for both the status and chat Channel Groups you created earlier. You can include a button that initiates this call, passing the User ID and including that in the channel pattern you created earlier to remove them from the Channel Group.
While you can perform both adding and removing friends in your game itself, we strongly recommend that your players should never be able to add/remove channels to/from a Channel Group within the game itself. When a player wants to add/remove friends, there should be a request made to your back-end server (it can be the same one when a user registers for an account) to handle these requests.
This is to increase the security of your game: you don’t want players who are not friends with each other to access a player’s friend group. Implementing Access Manager, which is a cryptographic token-based permission administrator allows you to regulate clients’ access to PubNub resources.
With this security control on the back-end server, you can implement a system that receives friend request invitations for a specific player and forwards the request to the intended target. The player chooses to accept or deny the request, sending the invitation back through your back-end server (protected via Access Manager) and forwarding it to the original sender. This ensures that your players’ friend lists are secure and well-managed.
What’s Next
In this how-to guide, you’ve learned how to add a friend list to your Unity game. Making use of Channel Groups and Presence, you’ve implemented a system where you can see friends’ online/offline and custom status changes using PubNub, as well as the foundation for adding and removing friends by securing permissions with Access Manager.
Game devs can learn more with the following resources:
- Read our documentation.
- Learn how to add real-time chat in Unity.
- Implement Presence into your Unity Engine project with our how-to guide.
- Understand how our Unity Showcase Game implements a scoreboard and leaderboard in real time.
- Dive into the Unity SDK source code.
Feel free to reach out to the Developer Relations Team at devrel@pubnub.com for any questions or concerns.