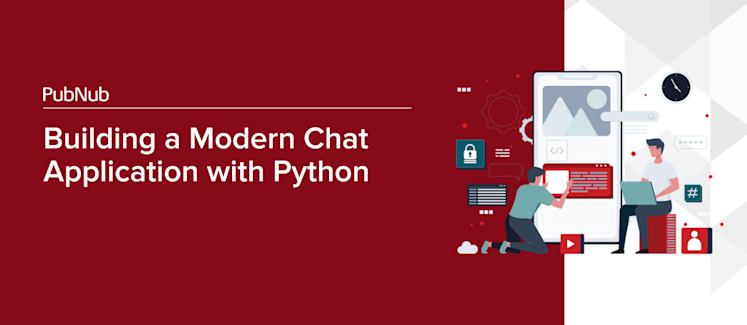
As a powerful and versatile programming language, Python allows developers to create chat applications that scale and adapt to different requirements. This blog will give you an overview of how to build a chat application and integrate PubNub for enhanced functionality.
This blog is aimed at beginners, so we'll cover all the essential concepts and keywords related to chat applications, such as server and client side, frontend and backend, as well as threading and sockets. With this foundational knowledge, you'll be well-prepared to build your Python chat application.
Why Build Chat Applications Using Python
Python has long been a popular choice for building chat applications thanks to its simplicity, readability, and a wide array of libraries and frameworks available. In this section, we'll discuss the reasons behind Python's popularity in the chat app development sphere and how it can help you create a seamless chat experience.
Benefits of Using Python for Chat Applications
Ease of use
Python's syntax is easy to learn and read, making it an ideal choice for beginners starting their chat app journey. The language is designed to be straightforward and concise, which helps developers write clean and maintainable code.
Versatility
Python offers numerous libraries and frameworks like Flask, Django, and socket.io that can simplify the chat app development process. These tools provide pre-built components and functions, enabling developers to focus on the core features of their chat application.
Community support
Python boasts a large and active community, offering valuable resources such as tutorials, forums, and GitHub repositories. This support makes it easier for developers to find solutions to problems and learn from others' experiences.
Integration with PubNub
PubNub's real-time chat API can be easily integrated with Python, enabling developers to add real-time messaging and presence capabilities to their chat applications. This powerful API provides the building blocks for a robust and scalable chat experience.
Limitations of Using Python for Building Chat Applications
Although Python is an excellent choice for chat app development, it does come with some limitations:
Performance
Python may not be the fastest language, which can impact the performance of real-time chat applications. However, this limitation can be mitigated by using efficient libraries and optimizing your code.
Concurrency
Python's Global Interpreter Lock (GIL) can be challenging when dealing with multi-threaded applications. Despite this, the language offers multiple ways to handle concurrency, such as using the threading library or alternative implementations like Jython or PyPy.
Python's benefits and extensive resources make it an excellent choice for building chat applications, even for those just starting. In the next section, we'll explore how to build Python chat applications, incorporating PubNub to enhance your chat app's capabilities.
How to Build Python Chat Applications
Building a chat application in Python can be a rewarding experience, especially with the right tools and libraries at your disposal. In this section, we'll guide you through building a Python chat app and show you how PubNub fits into the picture, enhancing your app's functionality.
Set up your environment: Start by setting up your Python development environment. Ensure you have Python 3 installed and consider using a virtual environment to manage your project dependencies.
Choose a framework or library: Select a suitable framework or library for your chat app, such as Flask or Django. These tools will provide you with essential building blocks and help streamline the development process.
Design your frontend: Create the frontend of your chat app using HTML, CSS, and JavaScript. You'll need to design an intuitive user input for users to send new messages and receive messages, display chat rooms, and manage connected clients.
Develop the backend: Build the backend (server side) of your chat application in Python, handling client connections, message sending, and message receiving. You'll need to use the socket library for server and client-side communication. Implement server and client sockets and methods for encoding and decoding messages.
Integrate PubNub: Enhance your chat app by integrating PubNub's real-time chat API. This will provide advanced features like real-time messaging, presence, and scalable infrastructure. To get started, follow our PubNub tour which will guide you through the top-level concepts.
Test and deploy: Thoroughly test your chat application, ensuring all features work as expected. Once satisfied with its performance, deploy your app to a server, making it accessible to users.
Optimize and scale: Monitor and optimize your chat application for performance and user experience. As your chat app gains popularity, consider leveraging PubNub's scalable infrastructure to handle increased user traffic and maintain a seamless chat experience.
By following these steps, you'll be well on your way to creating a successful Python chat application. In the next section, we'll explore some examples of Python chat apps that range from simple to more complex, providing you with inspiration and guidance for your chat app project.
Python Chat Examples
Let’s look at a few examples of Python chat applications, ranging from simple to more complex. These examples will provide you with inspiration and a better understanding of how to build your chat application.
Example 1: Simple Python Chat Server and Client
A simple chat server and client can be built using Python's socket library. The server will listen for client connections, manage connected clients, and handle message sending and receiving. The client will connect to the server, send messages, and display received messages. To learn more, check out this socket programming tutorial.
Example 2: Flask-based Chat Application
Flask is a lightweight web framework for Python that can be used to build a chat application. You can create a chat app with a user-friendly interface and real-time client communication by combining Flask with JavaScript and WebSockets.
Example 3: Django-based Chat Application with Channels
Django is a popular web framework for Python that can be used to build more complex chat applications. Using Django Channels, you can implement real-time messaging and handle WebSocket connections.
Getting Started with PubNub for Your Python Chat App
Integrating PubNub into your Python chat application offers numerous benefits, including enhanced functionality, real-time communication, and improved scalability. This section will guide you through the steps to start with PubNub for your chat app.
Step 1: Sign up for a PubNub account
To begin using PubNub, sign up for an account. You'll receive API keys that you'll need to incorporate into your Python chat application. Keep these keys secure, as they are required for accessing the PubNub API.
Step 2: Install the PubNub Python SDK
To use PubNub in your Python chat application, you'll need to install the PubNub Python SDK. You can do this using the package manager pip. Once installed, you can import the PubNub library into your chat application.
Step 3: Initialize the PubNub object
After importing the PubNub library, you must initialize a PubNub object using your API keys. This object will enable you to make connection requests to the PubNub API and utilize its features in your chat application.
Step 4: Publish and Subscribe to Channels
With the PubNub object initialized, you can now publish messages to channels and subscribe to receive messages from those channels. To do this, you'll create functions for publishing messages and handling incoming messages. Make sure to add a listener to your PubNub object to receive real-time updates.
These steps provide a basic overview of integrating PubNub into your Python chat application. For more advanced features like presence, history, message reactions, typing indicators, and access management, all of which are essential for modern chat applications, consult the official PubNub documentation.