What are Server-Sent Events?
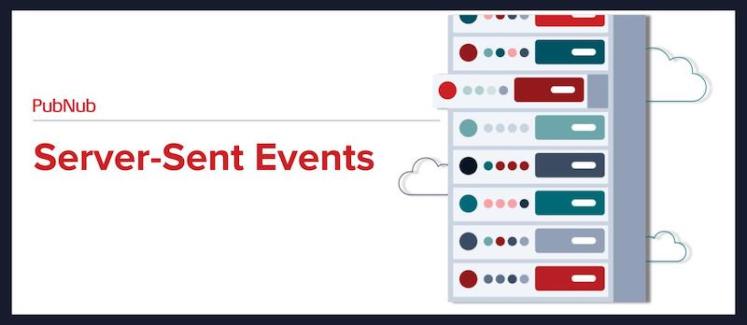
What are server-sent events?
Server-Sent Events (SSE) is one technology approach that allows servers to push real-time client updates over a single HTTP connection. It’s part of the HTML5 specification and provides a simple and efficient way for server applications to send data to clients. It is supported by about 96% of all browsers in the wild.
Like many other “data streaming approaches” for the web, SSEs establish a long-lived HTTP connection between the server and the client. Once the connection is established, the server can send data to the client anytime without requiring the client to make additional requests. This contrasts traditional HTTP connections, where the client must continuously poll the server for updates. SSEs provide servers with one of many approaches to real-time data pushing to clients.
How does SSE work?
The server-sent event protocol is based on the HTTP protocol, and it uses the "text/event-stream" content type to send a stream of events from the server to the client. The server sends a series of events, each consisting of a JSON-formatted message, to the client. The client can then process these events as they arrive and update the user interface accordingly.
To establish an SSE connection, the client sends an HTTP GET request to the server, requesting the SSE endpoint. The server responds with a "200 OK" HTTP status code and sets the "Content-Type" header to "text/event-stream." From then on, the server can send events to the client anytime.
The server sends the events as a continuous stream, separated by newlines. Each event is preceded by an "event" field, which specifies the type of the event. It can also include fields like "data" to provide additional information. The events are sent in the following format:
```
event: eventType
data: eventData
```
The client receives these events and processes them as they arrive. It can use JavaScript to listen for the "message" event on the SSE connection object and handle the received data accordingly. For example, the client can update the user interface with the new data or perform other necessary actions.
The SSE connection remains open until the client or the server closes it. The server can no longer send events if the client closes the connection. However, if the server closes the connection, the client must reconnect and continue receiving events.
What are the advantages of using server-sent events?
Server-sent events (SSEs) are a useful approach to streaming data unidirectionally (i.e., “one direction”) from server to client for streaming data like stock quotes, bitcoin prices, etc. Some benefits are:
Simplicity and ease of use: SSEs provide a straightforward way to establish a unidirectional connection between the server and the client. The client subscribes to an SSE endpoint, and the server can then push data to the client over this connection without needing the client to send requests constantly.
Reduced network overhead: SSEs significantly reduce network overhead compared to constantly polling techniques (i.e., having each client request data from servers every few seconds). With SSEs, the server only sends data to the client when new information is available, minimizing unnecessary data transfers and reducing bandwidth usage.
Standardized protocol: SSEs are based on the HTTP protocol, making them easily deployable and compatible with existing web infrastructure. SSEs use a single long-lived HTTP connection, eliminating the need for additional protocols or libraries.
Automatic reconnection: SSEs automatically handle reconnection in case of network disruptions or server failures. The client will automatically try reconnecting to the server when the connection is lost without additional code implementation.
Cross-domain support: SSEs support cross-domain communication, making them suitable for scenarios where the server and client are hosted on different domains. This allows for greater flexibility in designing and deploying applications.
Accessibility and compatibility: SSEs are built on HTML5 technology and are supported by modern web browsers. This ensures broad compatibility and accessibility for application developers without additional plugins or software installations.
SSEs vs. WebSockets
Server-sent events (SSEs) and WebSockets are both technologies that enable real-time communication between servers and clients. However, there are several key differences between the two.
Protocol: SSEs use the HTTP protocol, while WebSockets use a WebSocket protocol. SSEs are a part of the HTML5 specification, making them a natural fit for web-based applications. On the other hand, WebSockets are a separate protocol specifically designed for real-time communication.
Connection: SSEs use a unidirectional connection, where the server pushes data to the client. The client cannot send data back to the server using SSEs. Alternatively, WebSockets provide full-duplex communication, allowing the server and client to send data to each other.
Data Transmission: SSEs transmit data in a simple text format using a stream of events. An event type precedes each event and can contain arbitrary data. WebSockets transmit data in binary format, allowing for more efficient and versatile transmission of complex data structures.
Client Connection: SSEs maintain a long-lived connection between the client and server, with the server pushing data as soon as it becomes available. This makes SSEs well-suited for scenarios where the server must continuously send client updates, such as real-time notifications. WebSockets also maintain a long-lived connection but allow for bidirectional communication, making them suitable for more interactive applications, such as chat or online multiplayer games.
Browser Support: SSEs have broader browser support than WebSockets. SSEs are supported by all major browsers, including Internet Explorer 10 and above, while WebSockets have slightly more limited support, with some older browsers not fully supporting them.
Scalability: SSEs are generally more scalable than WebSockets because they use a single HTTP connection that servers can efficiently handle. On the other hand, WebSockets require a separate WebSocket connection, which may require additional server resources to handle multiple connections.
Security: SSEs use the same security mechanisms as the HTTP protocol, such as SSL/TLS encryption and authentication, with WebSockets requiring additional security measures, such as enforcing origin policies and handling cross-site scripting attacks.
Server-Sent Events Example Use Cases
SSEs can benefit a wide range of applications, especially those that require real-time data updates or need to push information from the server to the client. Here are some types of applications that can benefit from SSEs:
Real-time collaboration tools: Applications like project management tools, team chat applications, or document collaboration platforms can benefit from SSEs. SSEs allow these applications to instantly notify users of changes or updates, ensuring real-time collaboration and synchronization across team members.
Social media platforms: Social media applications heavily rely on real-time updates to deliver notifications, comments, likes, or messages to users. SSEs enable these platforms to instantly push notifications to users' devices, providing a seamless and engaging user experience.
Stock market and financial applications: SSEs are valuable for applications requiring real-time stock price updates, financial news, or market fluctuations. SSEs allow these applications to deliver up-to-date information to users in realtime, enabling them to make informed decisions quickly.
Real-time monitoring and dashboards: Applications that monitor system metrics, server health, or IoT devices can benefit from SSEs. SSEs enable these applications to push real-time updates to the dashboard, allowing users to monitor and respond to critical events as they happen.
Live streaming platforms: SSEs play a crucial role in live streaming applications, such as video conferencing platforms, gaming platforms, or live event streaming services. SSEs enable these applications to deliver real-time updates to users, ensuring a smooth and uninterrupted streaming experience.
What libraries exist to help implement server-sent events?
There are several libraries available that can help application developers implement server-sent events (SSEs) in their applications. These libraries provide the necessary tools and functionalities to connect the server and the client and enable real-time communication.
EventSource:
EventSource is a built-in browser API that provides a simple and straightforward way to consume SSEs. It allows developers to listen for updates from the server by creating an EventSource object and attaching event listeners to handle incoming messages. EventSource handles automatic reconnection, error handling, and other important SSE features. Most modern browsers support it.
SSE.js:
SSE.js is a popular JavaScript library that offers a simple and lightweight solution for implementing SSEs. It provides an abstraction layer on top of the EventSource API, making it easier to work with SSEs. SSE.js simplifies event handling, error management, and reconnection logic, allowing developers to focus on the core functionality of their applications.
Spring WebFlux:
Spring WebFlux is a reactive web framework for building scalable, high-performance applications. It includes built-in support for SSEs using the Server-Sent Events (SseEmitter) class. Spring WebFlux allows developers to create SSE endpoints and stream data to non-blocking clients. It also provides features like error handling, backpressure, and flow control.
Express.js:
Express.js is a popular Node.js web application framework. It offers middleware and plugins that can be used to implement SSEs in Express.js applications. The express-sse library is an intuitive way to create SSE endpoints. It manages connections, event broadcasting, and reconnection logic, allowing developers to integrate SSEs into their Express.js applications easily.
Django Channels and server-sent events:
Django Channels is a library for handling asynchronous communication in Django applications. It includes support for SSEs through the use of the EventStream consumer. Django Channels allows developers to create SSE endpoints and send events to clients in a scalable and efficient manner. It also provides features like authentication, authorization, and message filtering.
SSEs vs. HTTP Long Polling
Server-sent events (SSEs) are a technology that allows servers to push real-time updates to clients over HTTP without the need for the clients to poll the server for new data constantly. This is achieved through a persistent connection between the server and the client, where the server can send events to the client as soon as they occur.
In contrast, traditional client-server requests follow a request-response model, where the client initiates a request to the server and waits for a response. This requires the client to repeatedly send requests to the server to check for updates (i.e., polling), resulting in unnecessary network traffic and increased server load.
There are several advantages of using SSEs over traditional client-server requests:
Real-time updates: SSEs allow for real-time updates to be sent from the server to the client as soon as they occur. This enables applications to provide users with live, up-to-date information without manual refreshing or constant polling.
Reduced network traffic: SSEs eliminate the need for repeated client requests by establishing a persistent connection between the server and the client. This reduces network traffic and improves scalability, as the server only needs to send updates when they are available.
Lower server load: By reducing the number of client requests, SSEs help to lower the server load. This is especially beneficial for applications with many clients, as it allows the server to handle more concurrent connections without being overwhelmed.
Simplified client code: Compared to other real-time web technologies such as WebSockets, SSEs have a simpler client-side implementation. SSEs use a simple text-based protocol, where the server sends events as plain text over an HTTP connection. This makes it easier for developers to integrate SSEs into their applications, as they don't need to deal with complex protocols or libraries.
Cross-platform compatibility: SSEs are supported by all modern web browsers, making them a reliable and widely compatible solution for real-time updates. This ensures that applications using SSEs can reach a wide range of users without compatibility issues.
Message filtering: SSEs support message filtering, allowing clients to specify the types of events they are interested in. This reduces unnecessary data transfer and allows clients to receive only the needed updates, improving performance and efficiency.
Server-sent events (SSEs) play a crucial role in the architecture of real-time applications. They provide a standardized and efficient way for the server to push real-time updates to the client, enabling the client to receive and process data as it happens.
SSE vs. PubNub
Server-Sent Events (SSE) and PubNub are popular for developers building real-time chat and messaging applications. However, there are several key differences between these two approaches.
Protocol: SSE is one of many protocols that supports real-time data streaming. Running SSE requires the developer to host servers and manage the operations of the SSE environment. On the other hand, PubNub provides a globally deployed service for streaming real-time data bi-directionally, using open-source SDKs that manage protocols directly so developers don’t have to.
Bi-Directional Communication: While SSE enables server-to-client communication, it is primarily a one-way communication channel where the server can push messages to the client. In contrast, PubNub allows bi-directional communication, allowing the server and client to send and receive messages.
Scalability: SSE relies on a single HTTP connection for communication, which can limit its scalability, especially when dealing with many concurrent connections. PubNub is built for massive scale and can handle millions of concurrent connections, making it more suitable for high-traffic applications.
Reliability: SSE is prone to connection drops and may require manual reconnection handling in case of interruptions. PubNub offers built-in reliability features such as automatic reconnection, message retry, and fallback mechanisms, ensuring message delivery even in challenging network conditions.
Security: SSE does not provide built-in security measures, requiring developers to implement authentication and encryption mechanisms. PubNub provides security features such as end-to-end encryption, access control, and authentication options, making it a more secure choice for handling sensitive data in real-time applications.
While both Server-Sent Events (SSE) and PubNub are viable options for building real-time applications, they have distinct differences that developers should consider. If you require bi-directional communication, scalability, reliability, and built-in security measures, PubNub may be the better choice. Ultimately, the decision depends on your application's specific requirements and priorities.
Conclusion
SSEs are a valuable tool as they provide a standardized and efficient way to implement real-time updates for unidirectional use cases. They offer benefits such as instant updates, reduced network traffic and server load, simplified client-side implementation, and compatibility with existing web infrastructure. By leveraging SSEs, developers can enhance the user experience of their realtime apps and provide users with live and up-to-date information more efficiently and scalable.
With over 15 points of presence worldwide supporting 800 million monthly active users and 99.999% reliability, you’ll never have to worry about outages, concurrency limits, or any latency issues caused by traffic spikes. PubNub is perfect for any application that requires real-time data.
Sign up for a free trial and get up to 200 MAUs or 1M total transactions per month included.