What are RESTful APIs?
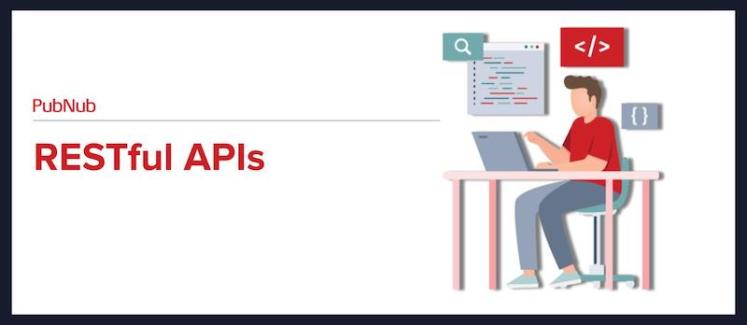
What is a RESTful API?
A RESTful API, or a Representational State Transfer API, is an architectural style for designing networked applications. It is a set of rules and constraints that govern how web services communicate with each other over the internet.
In a RESTful API, resources are represented as URLs (Uniform Resource Locators) and are accessed using the standard HTTP methods such as GET, POST, PUT, and DELETE. These methods correspond to the CRUD (Create, Read, Update, and Delete) operations on resources.
The key principles of a RESTful API include:
Stateless: Each request from the client to the server should contain all the information necessary to understand and process the request. The server should not store any client state between requests.
Uniform interface: The API should have a consistent and standardized set of methods and protocols for communication. This includes using HTTP requests for specific operations (e.g., GET for retrieving a resource) and standard status codes for indicating the outcome of a request.
Client-Server architecture: The client and server are separate entities that communicate over a network. The client is responsible for the user interface and user experience, while the server handles the business logic and data storage.
Cacheable: Responses from the server can be cached by the client to improve performance and reduce the load on the server. The server can include caching instructions in the response
Layered system: The architecture can comprise multiple layers, each with a specific role and responsibility. This allows for flexibility and scalability in the system design.
What are the benefits of using RESTful APIs?
RESTful APIs have several benefits in developing realtime applications.
Scalability
RESTful APIs are designed to be stateless, meaning that each API request carries all the necessary information to fulfill the request. This design allows for easy scalability as the server does not need to maintain any client-specific state. It enables developers to horizontally scale their application by adding more servers as the demand increases, without worrying about managing session state or affinity.
Interoperability
RESTful APIs follow a uniform and standardized architecture, making them highly interoperable. They can be integrated into different platforms and programming languages, allowing developers to build applications that can communicate with each other seamlessly. This interoperability allows for easy integration with other services and systems, enabling developers to leverage existing infrastructure and services.
Simplicity and Ease of Use
RESTful APIs are designed to be simple and easy to understand. They use standard HTTP methods (GET, POST, PUT, DELETE) and utilize well-known data formats such as JSON or XML. This simplicity makes it easier for developers to build, test, and maintain APIs. Additionally, RESTful APIs are self-descriptive, providing metadata about themselves, making it easier for developers to understand and consume the API.
Security
RESTful APIs can be secured using standard security protocols such as HTTPS, providing encryption and authentication. They also support token-based authentication mechanisms, such as OAuth, which adds an extra layer of security. By following best practices for API security, developers can ensure that their applications are protected against unauthorized access and data breaches.
RESTful APIs vs. Regular APIs
RESTful APIs follow architectural principles and constraints. While both RESTful and regular APIs serve as intermediaries between different software applications, there are some key differences between the two.
Design Style
RESTful APIs adhere to the principles of REST, which emphasize a stateless client-server communication model. They utilize standard HTTP methods (GET, POST, PUT, DELETE) to perform operations on resources. On the other hand, regular APIs do not necessarily follow the REST principles and can have their design styles.
Statelessness
RESTful APIs are stateless, meaning that each request from a client to a server contains all the information necessary to understand and process the request. The server does not store any information about the client's previous requests. In contrast, regular APIs can maintain state, keeping track of client sessions and storing data between requests.
Uniform Interface
RESTful APIs have a uniform interface, which includes standard HTTP methods, resource-based URLs, and data formats like JSON or XML for representing resources. This uniformity makes it easier for developers to understand and interact with the API. Regular APIs may have varying interfaces depending on their design.
Scalability
RESTful APIs are designed to be scalable and can handle many concurrent requests. They achieve this by leveraging the statelessness and caching capabilities of HTTP. Regular APIs may or may not be designed with scalability in mind, depending on their specific implementation.
So, why is scalability important for chat and messaging applications?
Scalability is crucial for chat and messaging applications because they often handle many concurrent users and messages. As the number of users and messages increases, the application should be able to handle the load without experiencing performance issues or downtime.
By using a scalable API, developers can ensure that their realtime apps can handle the increasing demands of their users. A scalable API can distribute the load across multiple servers or resources, allowing horizontal scaling. As the user base grows, additional servers can be added to handle the increased traffic.
Scalability is especially important for real-time applications like chat and messaging, where responsiveness and low latency are key. Users expect instant message delivery and real-time updates, regardless of the number of concurrent users. A scalable API can help meet these expectations by efficiently handling the high volume of messages and delivering them in a timely manner.
In addition to handling increasing user loads, a scalable API allows for future growth and expansion. As the application evolves and new features are added, the API should be able to accommodate these changes without major disruptions or performance degradation. This scalability ensures that the application can continue to meet the needs of its users as it grows and evolves.
Scalability is essential for real time applications to provide a seamless and reliable user experience. By choosing a scalable API, developers can ensure that their applications can handle the demands of a growing user base and the evolving needs of the application. This allows for smooth performance and responsiveness and future-proofs the application for potential growth and expansion.
Security
Regarding chat and messaging applications, security is paramount. Users exchange sensitive information and expect their conversations to remain private and secure. RESTful APIs can offer high security by utilizing HTTPS encryption and implementing authentication and authorization mechanisms.
HTTPS encryption ensures that the data transmitted between the client and the server is encrypted, making it difficult for unauthorized users to intercept and access the information. This helps protect the privacy and confidentiality of the messages exchanged within the application.
Authentication mechanisms, such as OAuth or token-based authentication, verify the identity of the users and prevent unauthorized access. Authorization mechanisms, such as role-based access control, determine the level of access each user has within the application. These security measures ensure only authorized users can access and interact with the chat and messaging application.
Additionally, RESTful APIs can provide features like rate limiting and request validation to prevent abuse and protect against common security vulnerabilities, such as denial-of-service attacks or injection attacks. These measures help maintain the integrity and availability of the application.
By choosing a secure API, developers can build chat and messaging applications that prioritize the privacy and security of their users' conversations. This builds trust with users and ensures compliance with data protection regulations and industry best practices.
Best practices for designing a RESTful API
Designing a RESTful API involves following certain best practices to ensure scalability, maintainability, and ease of use for developers. Here are some recommended practices:
Use clear and meaningful endpoints: API endpoints should be intuitive and descriptive, representing the resources they manipulate. For example, instead of using vague endpoints like "/data" or "/get", use endpoints like "/users" or "/products".
Use HTTP verbs correctly: Utilize the appropriate HTTP verbs (GET, POST, PUT, DELETE) to perform specific actions on resources. GET for retrieving data, POST for creating new resources, PUT for updating existing resources, and DELETE for removing resources.
Use HTTP status codes correctly: Return the appropriate HTTP status codes to indicate the response status. For example, 200 for success, 404 for not found, 201 for resource created, etc. This helps clients understand the outcome of their requests.
Versioning: Consider versioning your API to allow future updates without breaking existing clients. This can be done by including the version number in the API URL (e.g., "/v1/users").
Authentication and authorization: Implement secure authentication mechanisms, such as OAuth or JWT, to protect your API from unauthorized access. Use authorization mechanisms like role-based access control (RBAC) to limit user actions based on their roles or permissions.
Use sensible pagination: Implement pagination to retrieve data in smaller chunks when dealing with large data collections. This improves performance and reduces the amount of data transferred over the network.
Error handling: Implement proper error handling by returning informative error messages and appropriate HTTP status codes. This helps developers understand and troubleshoot issues more effectively.
Consistency in response format: Maintain consistency in the response format of your API. This includes using a consistent structure for error responses, following naming conventions for fields, and providing helpful documentation for developers.
Rate limiting: Implement rate limiting to prevent abuse and protect your API from excessive requests. This can help ensure fair usage and maintain the performance and availability of your API.
Documentation: Provide comprehensive documentation for your API, including endpoint descriptions, request/response examples, and any necessary authentication details. This will make it easier for developers to integrate and use your API effectively.
What is a typical response format for a RESTful API?
A typical response format for a RESTful API is JSON (JavaScript Object Notation). JSON is a lightweight data-interchange format that is easy for humans to read and write and for machines to parse and generate. Programming languages widely support it and are the most common choice for data exchange in RESTful APIs.
JSON response format consists of key-value pairs enclosed in curly braces ({}) and separated by commas. The keys are strings, and the values can be of different data types, including strings, numbers, booleans, arrays, and nested objects. Here is an example of a JSON response header:
```json
{
"id": 123,
"name": "John Doe",
"email": "john.doe@example.com",
"age": 30,
"active": true,
"roles": ["admin", "user"],
"address": {
"street": "123 Main Street",
"city": "New York",
"country": "USA"
}
}
```
In this example, the response represents a user object with properties such as id, name, email, age, active, roles, and address. The roles property is an array, and the address property is a nested object.
However, it's worth noting that other response formats like XML (eXtensible Markup Language) can also be used in RESTful APIs, although JSON has become the de facto standard due to its simplicity, wide support, and better readability for developers. It is recommended to use JSON as the response format for a RESTful API to ensure consistency and interoperability with clients. Implementing rate limiting is also crucial to protect the API from abuse and ensure fair usage. Rate limiting limits the number of requests a client can make within a certain period. Enforcing rate limits can prevent excessive requests that can overload the API and affect its performance and availability.
What is the difference between JSON and XML when used with a RESTful API?
JSON and XML are popular choices for representing data using a RESTful API. However, there are some key differences between the two.
Syntax: JSON uses a lightweight and human-readable syntax derived from JavaScript object syntax. It primarily consists of key-value pairs, making it easier to read and write for developers. On the other hand, XML uses a more verbose and hierarchical structure with tags, making it more suitable for complex and structured data.
Data Types: JSON supports a limited set of data types, including strings, numbers, booleans, arrays, and objects. It does not directly support more complex data types like dates or binary data. In contrast, XML allows developers to define custom data types using Document Type Definitions (DTD) or XML Schema Definitions (XSD), making it more flexible in handling different data types.
Readability: JSON is generally more readable than XML due to its simpler syntax and compact structure. This makes it easier for developers to parse and understand the data. On the other hand, XML can become verbose and repetitive, especially for large data sets, which may decrease readability.
Parsing: JSON can be parsed directly by JavaScript, making it a natural choice for web-based applications. On the other hand, XML requires an XML parser, which adds an extra layer of complexity and overhead to the parsing process.
Performance: JSON is typically faster to parse and process than XML. JSON has a simpler structure and fewer characters, resulting in faster data transmission and parsing. With its verbose structure and additional parsing requirements, XML can be slower and more resource-intensive.
Support: JSON has recently gained widespread support and popularity, especially in web development and APIs. Most programming languages have built-in support for JSON, making it easy to work with. While still widely used in certain industries and legacy systems, XML has lost some popularity in favor of JSON.
Compatibility: JSON is compatible with modern web technologies and is often used with JavaScript-based front-end frameworks and libraries. While still compatible with web technologies, XML may require additional processing and transformation to work seamlessly with JavaScript-based applications.
What are the common HTTP methods used in a RESTful API?
In a RESTful API, several common HTTP methods perform different actions on resources. The HTTP protocol defines these methods and is widely used in building web services. The most commonly used HTTP methods in a RESTful API are:
GET: The GET method retrieves data from a server. It is used to fetch a specific resource or a collection of resources from the server. When a GET request is made, the server returns the requested data in the response body.
POST: The POST method sends data to the server to create a new resource. It is commonly used to submit form data or upload files to the server. The data sent in a POST request is typically included in the request body.
PUT: The PUT method updates an existing resource on the server. It replaces the entire resource with the updated data in the request body. A new resource can be created using PUT if the resource does not exist.
DELETE: The DELETE method removes a specific resource from the server. It instructs the server to delete the specified resource. Once the resource is deleted, subsequent requests to retrieve or modify the resource will return an error.
PATCH: The PATCH method performs a partial update on a resource. It is similar to the PUT method, but instead of replacing the entire resource, it only updates the specified fields or properties of the resource.
It is important to note that these HTTP methods have specific semantics and should be used according to their intended purpose. For example, GET should be used for retrieving data and should not have side effects, while POST should be used for creating new resources.
By understanding and using these common HTTP methods effectively, developers can design RESTful APIs that are intuitive, easy to use, and adhere to best practices in web service development.
What is the difference between a resource and an endpoint in a RESTful API?
In a RESTful API, a resource and an endpoint are two fundamental concepts that serve different purposes.
A resource is a logical entity representing a piece of data or an object in the system. It can be anything from a user, a product, or a blog post to more abstract concepts like a shopping cart or a reservation. Resources are typically identified by unique URLs, known as Uniform Resource Locators (URLs) or Uniform Resource Identifiers (URIs).
On the other hand, an endpoint is a specific URL or URI that serves as a point of interaction with a resource. It represents a specific operation or action that can be performed on or with a resource. Endpoints are how clients can access and manipulate the resources in a RESTful API. They define how a client can interact with a resource, such as retrieving, creating, updating, or deleting it.
Simply put, a resource is the entity or object itself, while an endpoint is the URL that allows you to perform actions on that resource.
Let's take a practical example to illustrate this distinction. Suppose we have a RESTful API for managing a collection of books. The books are the resources, and each can have multiple endpoints. These endpoints could include:
- GET /books/{id}: Retrieve the details of a specific book.
- POST /books: Create a new book.
- PUT /books/{id}: Update the details of a specific book.
- DELETE /books/{id}: Delete a specific book.
In this example, the books are the resources, and the endpoints represent the different actions that can be performed on those resources. Clients can retrieve, create, update, or delete specific books in the collection by the appropriate endpoint. The endpoints provide a clear and structured way for clients to interact with the resources in the API.
Understanding the difference between resources and endpoints is crucial for designing and building RESTful APIs. By properly defining and organizing resources and endpoints, developers can create a well-structured and intuitive API that allows clients to interact effectively with the available data and functionalities.
Conclusion
Designing a RESTful API for realtime applications requires careful consideration of resource and endpoint organization, scalability, security, performance, and error handling. By following best practices and implementing a well-structured API, developers can create a scalable and secure platform that provides a seamless user experience.
Implementing proper authentication and authorization mechanisms ensures the security of the API and protects sensitive user data. Minimizing the number of API calls and efficiently handling errors further enhances the performance and usability of the application. By adhering to these guidelines, developers can build real time apps using REST APIs.
With over 15 points of presence worldwide supporting 800 million monthly active users and 99.999% reliability, you’ll never have to worry about outages, concurrency limits, or any latency issues caused by traffic spikes. PubNub is perfect for any application that requires real-time data.
Sign up for a free trial and get up to 200 MAUs or 1M total transactions per month included.