YouTube with Friends using WebRTC
Since we wrote this post, we've made some changes to our what we do, and what we don't do with WebRTC. Learn more here.
WebRTC is becoming widely adopted by top companies. If you don't know it already, WebRTC is a free, open-source project that provides simple APIs for creating Real-Time Communications (RTC) for browsers and mobile devices. It powers many modern video chatting services, even Chromecast uses it to broadcast your chrome tab to the TV screen. WebRTC makes streaming any content such as video, audio, even arbitrary data simple and fast. Today we will be building a video chat application that allows you to watch YouTube with a friend!
PubNub for Signaling
WebRTC is not a standalone API, it requires a signaling service to coordinate communication. Metadata needs to be sent between callers before a connection can be established.
This metadata includes:
- Session control messages to open and close connections
- Error messages
- Codecs/Codec settings, bandwidth and media types
- Keys to establish a secure connection
- Network data such as host IP and port
Once signaling has taken place, video/audio/data is streamed directly between clients using WebRTC's PeerConnection API. This peer-to-peer direct connection allows you to stream high-bandwidth robust data, like video. In addition, we will be using DataChannels to stream messages through our RTC connection.
PubNub makes this signaling incredibly simple, and then gives you the power to do so much more with your WebRTC applications.
Browser Compatibility
WebRTC is supported by popular browsers such as Chrome and Firefox, but there are many browsers on which certain features will not work. This is a list of WebRTC supported browsers.
Part 1: The Video Setup
Time to begin! First we will make the bare minimum WebRTC video chat. Then, in Part 2 we will be using WebRTC DataChannels, and the YouTube API to create our application. The live demo for this tutorial can be found here.
A Note on Testing and Debugging
If you try to open file://<your-webrtc-project> in your browser, you will likely run into Cross-Origin Resource Sharing (CORS) errors since the browser will block your requests to use video and microphone features. To test your code you have a few options. You can upload your files to a web server, like Github Pages if you prefer. In production, WebRTC requires HTTPS, so if you choose this method, visit your pages as https://yourusername.github.io/project. However, to keep development local, I recommend you setup a simple server using Python.
To do this, open your terminal and change directories into your current project and depending on your version of Python, run one of the following modules.
cd <project-dir>
# Python 2
python -m SimpleHTTPServer <portNo>
# Python 3
python -m http.server <portNo>
For example, I run Python 2.7 and the command I use is python -m SimpleHTTPServer 8001. Now I can go to http://localhost:8001/index.html to debug my app! Try making an index.html with anything in it and serve it on localhost before you continue.
The HTML5 Backbone
This should leave you with a very basic HTML backbone that looks something like this:
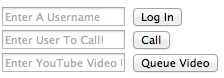
The player div will house our YouTube video, while vid-box will hold the video chat. We will use the forms to log-in, place calls, and enqueue videos.
The JavaScript Imports
There are three libraries that you will need to include to make WebRTC operations much easier. The first thing you should include is jQuery to make modifying DOM elements a breeze. Then, you will need the PubNub JavaScript SDK to facilitate the WebRTC signaling. Finally, include the PubNub WebRTC SDK which makes placing phone calls as simple as calling the dial(number) function.
We will include the YouTube API later. Now we are ready to write our calling functions for login, makeCall, and end!
Making and Receiving Calls
In order to start facilitating video calls, you will need a publish and subscribe key. To get your pub/sub keys, you’ll first need to sign up for a PubNub account. Once you sign up, you can find your unique PubNub keys in the PubNub Developer Dashboard. The free Sandbox tier should give you all the bandwidth you need to build and test your WebRTC Application.
You can see we use the username as the phone's number, and instantiate PubNub using your own publish and subscribe keys. The next function phone.ready allows you to define a callback for when the phone is ready to place a call. I simply change the username input's background to green, but you can tailor this to your needs.
The phone.receive function allows you to define a callback that takes a session for when a session (call) event occurs, whether that be a new call, a call hangup, or for losing service, you attach those event handlers to the sessions in phone.receive.
I defined session.connected which is called after receiving a call when you are ready to begin talking. I simple appended the session's video element to our video div.
Then, I define session.ended
which is called after invoking phone.hangup. This is where you place end-call logic. I simply clear the video holder's innerHTML field.
We now have a phone ready to receive a call, so it is time to create a makeCall function.
If window.phone is undefined, we cannot place a call. This will happen if the user did not log in first. If it is, we use the phone.dial function which takes a number and an optional list of servers to place a call.
Finally, to end a call or hangup, simply call the phone.hangup function and hide the video div.
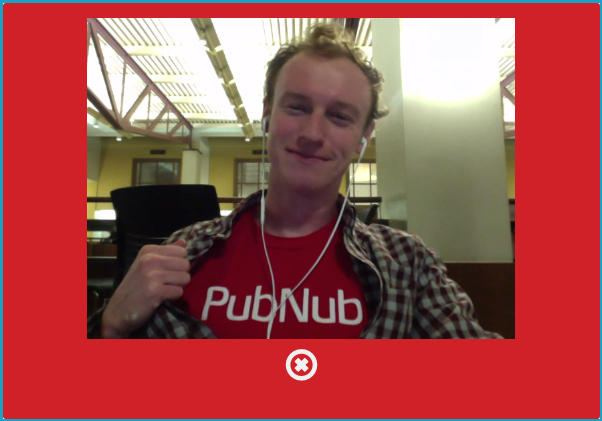
You should now have a working video chatting application! When you are ready we can move on and implement the DataChannels.
DataChannels and YouTube
All that's left to do is set up the YouTube API, and then use WebRTC DataChannels to synchronize playback, so lets get going!
The YouTube IFrame API
We will start by filling the player div we created in the previous section with a YouTube IFRAME. The following code is all based off the YouTube API documentation.
This will look for a div with id player and place an iframe inside of it. You can change vidId to be the id of any YouTube video. onPlayerStateChange is a callback that we will have to implement shortly.
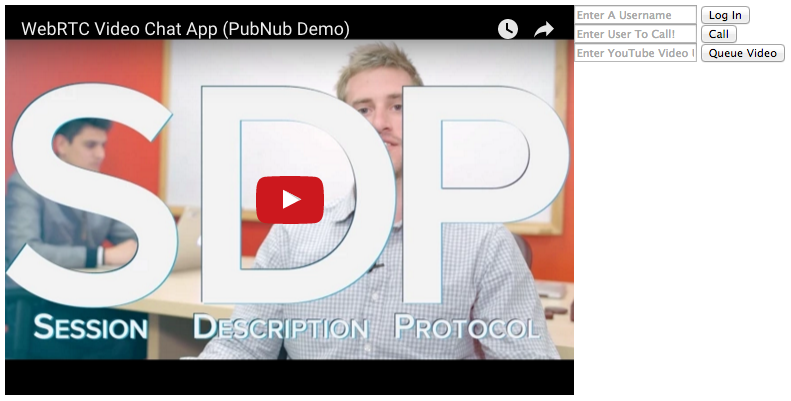
DataChannels to Synchronize Playback
Currently, we can video chat, and we can watch YouTube. Now we have to synchronize video playback. This includes play/pause, seeks, and what video is currently playing. We will accomplish all of this using the WebRTC DataChannel API. First, we need to define a few variables that will be used in the synchronization.
We will use these variables to implement the onPlayerStateChange function from the previous step. The YouTube API defines PlayerStates which are attached to the onPlayerStateChange event. Here is a quick overview of some events and when they are triggered:
– `YT.PlayerState.PLAYING` when the play button is clicked
– `YT.PlayerState.PAUSED` when the pause button is clicked
– `YT.PlayerState.BUFFERING` when the video is buffering (seeks)
The PubNub WebRTC SDK makes the DataChannel API extremely easy to use. To send data, use the phone.sendData. Makes sense to me! Now, lets implement that callback, onPlayerStateChange.
The YouTube API calls this function when the player's state changes. We attach a username to the event and then handle the state change as needed. The event comes through as JSON (e.g. {data:PLAYING}). Play/pause are handled by simply sending the event through the WebRTC DataChannel to the other user. When a user seeks, the BUFFERING callback will be triggered. If you seek, the event gets sent through the data channel. Otherwise, the other user sent you the buffering change, so we ignore it.
If you have followed any of my other WebRTC tutorials, you will notice that we use phone.sendData instead of phone.send. sendData will send 1:1 messages through the WebRTC DataChannel API for immediate interactions, while send uses the 1:many PubNub streaming network. The PubNub WebRTC SDK allows us to seamlessly transition between both.
Note: The PubNub streaming network is ideal for things like chat, while DataChannels are quicker for user interactions such as synchronization.
Handling DataChannel Events
In the previous step we sent state changes through the data channel. Now we have to handle the changes by creating an onDataReceived function, and then registering it as a callback using phone.datachannel in our login function.
If the incoming data message is from yourself, return and ignore it. We handle the received data using several YouTube player functions. If the user we are chatting with sent a PLAYING change, we simply call player.playVideo. If we receive a BUFFERING change, the other user likely seeked. We handle this by calling player.seekTo. We need to set seek=true so that our onStateChange function does not send a BUFFERING message back, causing an infinite seek loop. We also check for VID_CUE events, which change the currently playing video.
We will implement a cue function in the next step. First, we need to register this data channel callback. At the end of your login function, before you return false, register the callback with phone.datachannel.
Adding Videos to the Queue
Take a deep breath, the hard part is over. In this final step we will implement a function to change the currently playing video. Luckily, the YouTube API gives us a nice function to do it, player.cueVideoById.
player.cueVideoById(videoId:String,
startSeconds:Number, suggestedQuality:String):Void
When looking at a YouTube URL, for example https://www.youtube.com/watch?v=dQw4w9WgXcQ, the video ID is the final value dQw4w9WgXcQ. Our queue function will parse this off the url.
If the field is empty, we ignore the click by returning false. We parse the ID by splitting at v= and the following &, or the end of the string if no ampersand is present. When we have the ID, we cue the video and send a message through the data channel to notify users of the new video. We create the JSON payload in the msg variable and send it with phone.sendData.
You made it! Time to relax by dialing a friend and watching a funny cat video. Now its up to you to dream up some new features for this app. Hope you enjoyed this WebRTC tutorial, see you next time!
Now What?
Why not make your WebRTC app something great?
Use PubNub to Enhance Your WebRTC App
PubNub will take your WebRTC app to the next level. For example, let's build a community. Say you wanted a live chat for all users watching a current video. DataChannels are only good at 1:1 interactions. With PubNub, you can subscribe users to a channel of the YouTube VideoID, and use Storage & Playback to display what people watching this video have been talking about. You can also subscribe all users on the app to a global channel and send real-time video recommendations of currently viral videos to your community. Simply create a callback much like onPlayerStateChange and register it using phone.send instead of sendData!
Production Quality WebRTC with XirSys
While PubNub handles all the signaling for you WebRTC application, there are many other server side features that you will likely need to handle the quirks of real-world connectivity. In reality, most devices live behind layers of NAT, proxies, and corporate firewalls. XirSys is a WebRTC hosting company that provides production quality STUN and TURN servers to solve these problems. Sign up on their website to receive your free API key so you can start using using their solutions!
To use a XirSys server in your application, follow their Quick Start Guide to make a domain. You will need to navigate to this page and create a new domain. This domain will automatically be populated with an application “default” and a room “default” which we will use to get ICE servers.
ICE Servers can be gathered by placing a request to the XirSys API. Note: Requires jQuery.
function get_xirsys_servers() {
var servers;
$.ajax({
type: 'POST',
url: 'https://service.xirsys.com/ice',
data: {
room: 'default',
application: 'default',
domain: '',
ident: '',
secret: 'Your API key, on dashboard',
secure: 1,
},
success: function(res) {
console.log(res);
res = JSON.parse(res);
if (!res.e) servers = res.d.iceServers;
},
async: false
});
return servers;
}
This will return the servers you can use to start a video chat with production quality dependency. The PubNub phone.dial has an optional argument of servers to use to place a call, so to use your servers, simply call it as follows: phone.dial(number, get_xirsys_servers()); You're all ready to go now! Happy chatting!
Want to learn more?
If you made it this far, you must. Here are some other resources PubNub offers on WebRTC: