Real-time Location Tracking on a Map in JavaScript
In this tutorial, we'll show you how to get started with real-time location tracking on a live-updating map using the EON framework and the Mapbox API, all in JavaScript. In a nutshell, our application will stream data from a connected user in real time with their location, and publish that data to a map. As the user moves, their marker on the map reflects their change of location.
Check out our live demos below:
For an overview of how EON works, including a getting started guide for the overall framework, check out our Getting Started with Real-time JavaScript Dashboards.
Click here for the full EON GitHub repository.
Now, let's get started!
Quickstart
Init
Parameter | Value | Default |
---|---|---|
id | The ID of the element where the map will be rendered. | undefined |
mb_token | Mapbox API Token. | undefined |
mb_id | Mapbox Map ID. | undefined |
transform | Method for changing the payload format of your stream. | function(m){} |
history | Use PubNub history call to retrieve last message. This will display points at their last known location. Requires PubNub Storage & Playback to be enabled. | false |
pubnub | An instance of the PUBNUB javascript global. This is required when using your own keys. See the subscribe_key example. | false |
connect | A function to call when PubNub makes a connection. See PubNub subscribe. | function(){} |
marker | A custom Mapbox marker object. Use this to change the marker icon, tooltip, etc. | L.maker |
rotate | Add bearing to markers in options.angle . This won't have any effect unless you're using a rotated marker type. | false |
Simple Example
Call eon.map({})
. Check out the table of options above for more information.
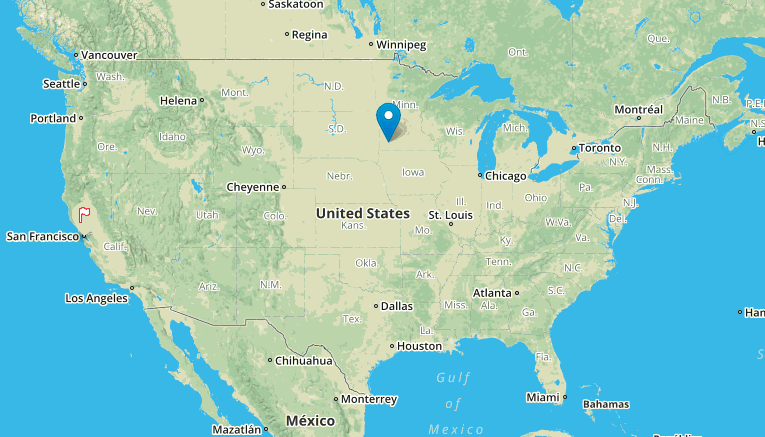
Lat/Long Values
eon.map
expects an array of objects to be published on the same channel it's subscribed to. More on publishing in the next section.
For example, below you can find a list of all the Torchy's Tacos in Austin, TX.
Publishing Messages
The function below is called connect
and fires when the pubnub_mapbox
library is ready.
This function uses the included PubNub library to pubnub.publish() packets to the pubnub.subscribe() call waiting inside the Mapbox framework.
Notice how the subscribe_key
and channel
matches.
You may want to publish data from the back-end instead, and in that case, check out our docs for our back-end SDKs (we have over 70 total!).
Following a Point
You can tell the map to follow a point to its new location whenever data is received by supplying a message
callback.
Marker Customization
You can supply a custom Mapbox marker object with custom tooltips by extending the L.marker
object provided by the Mapbox API. Learn more about custom Mapbox API markers here.
Configure Using Your Own PubNub API Keys
Using your own API Key with EON Maps
To get your unique publish/subscribe keys, you’ll first need to sign up for a PubNub account. Once you sign up, you can get your unique PubNub keys in the PubNub Developer Dashboard. Our free Sandbox tier should give you all the bandwidth you need to build and test your app with the PubNub API.
You can set the pubnub
init parameter when using EON for real-time maps. This allows you to configure PubNub client connections with extra security options such a auth_key
and your cipher_key
.
You should also set secure: true
and ssl: true
as well.
Customizing with Mapbox
The MapBox map object is returned by eon.mapbox
and can be customized using the Mapbox API. See the Mapbox examples page.
You can also customize your map using the Mapbox map editor. You can change the map background style, add static markers, etc. Visit Mapbox for your own API key.
Distributed Systems
The EON library compiles all messages at designated intervals. This means you can publish from multiple sources into one map.
For example, you can map the individual locations of three phones by supplying the same channel to your PubNub publish requests. The flight example works like this; not every flight is updated on every subscribe call.
That's all for this real-time location tracking maps getting started. Stay tuned for future tutorials on building real-time dashboards with EON!