PubSub for MKR1000 using ATWINC1500: MQTT and Arduino SDK
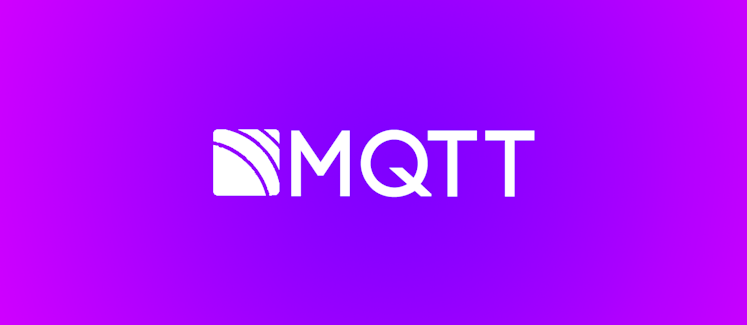
This tutorial will explain how to use both the PubNub Arduino SDK and PubNub MQTT gateway to send and receive data from a MKR1000 development board using a built-in ATWINC1500 WiFi module.
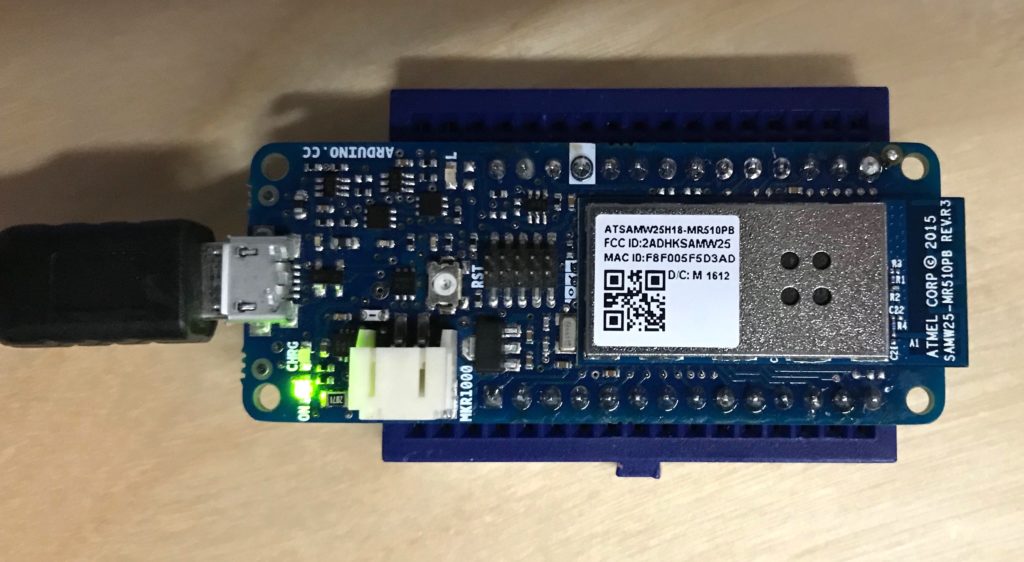
Check out the first part of this series, Publish and Subscribe for Internet of Things with MQTT and PubNub Arduino SDK, if you’re not sure why to use PubNub with IoT or when you should use the PubNub Arduino SDK or PubNub MQTT Gateway.
Getting Started
First, download and install the latest Arduino IDE. Arduino 1.8.9 on macOS Mojave was used to make this tutorial.
Next, sign up for a PubNub account. Once you sign up, you can get your unique PubNub keys from the PubNub Admin Dashboard. PubNub provides a 99.999% SLA on all of its services making it a great choice for transmitting IoT data reliably.
Sign up using the form below:
Setup the Arduino IDE
Before you can use the MKR1000 with the Arduino IDE you’ll need to add it with the Boards Manager.
Open the Arduino IDE.
Navigate to the Board Manager by going to Tools > Boards > Boards Manager. Search for “Arduino SAMD Boards”. Install Arduino SAMD Boards (32bits ARM Cortex-M0+).
Connect your MKR1000 development board to your computer with a USB cable. The driver should install automatically if needed.
Select the port that your board is connected to under Tools > Port.
Select the board for use by going to Tools > Boards.
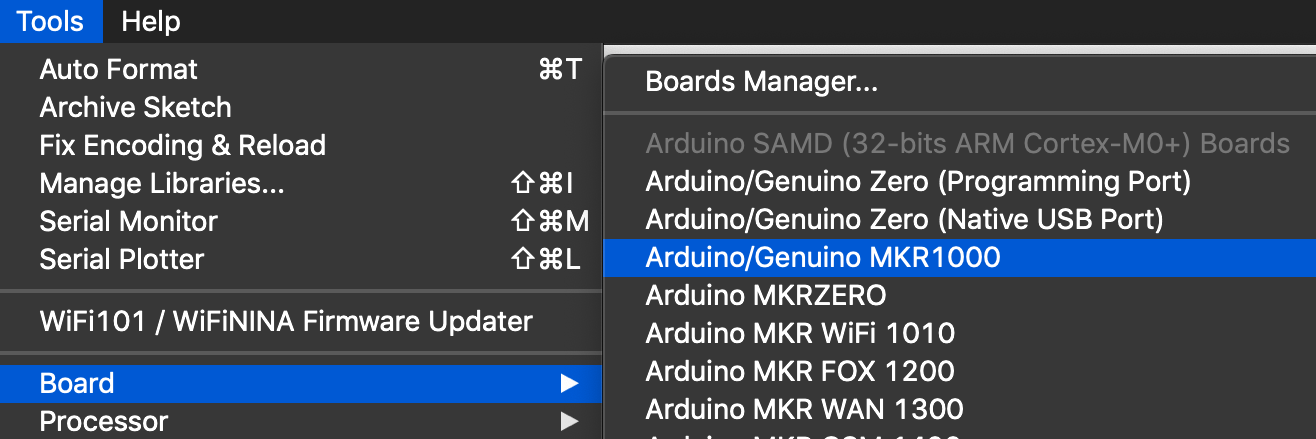
Install PubNub Arduino SDK
Install the PubNub Arduino SDK by going to Sketch > Include Library > Manage Libraries. Search for “PubNub”. Then select Install.
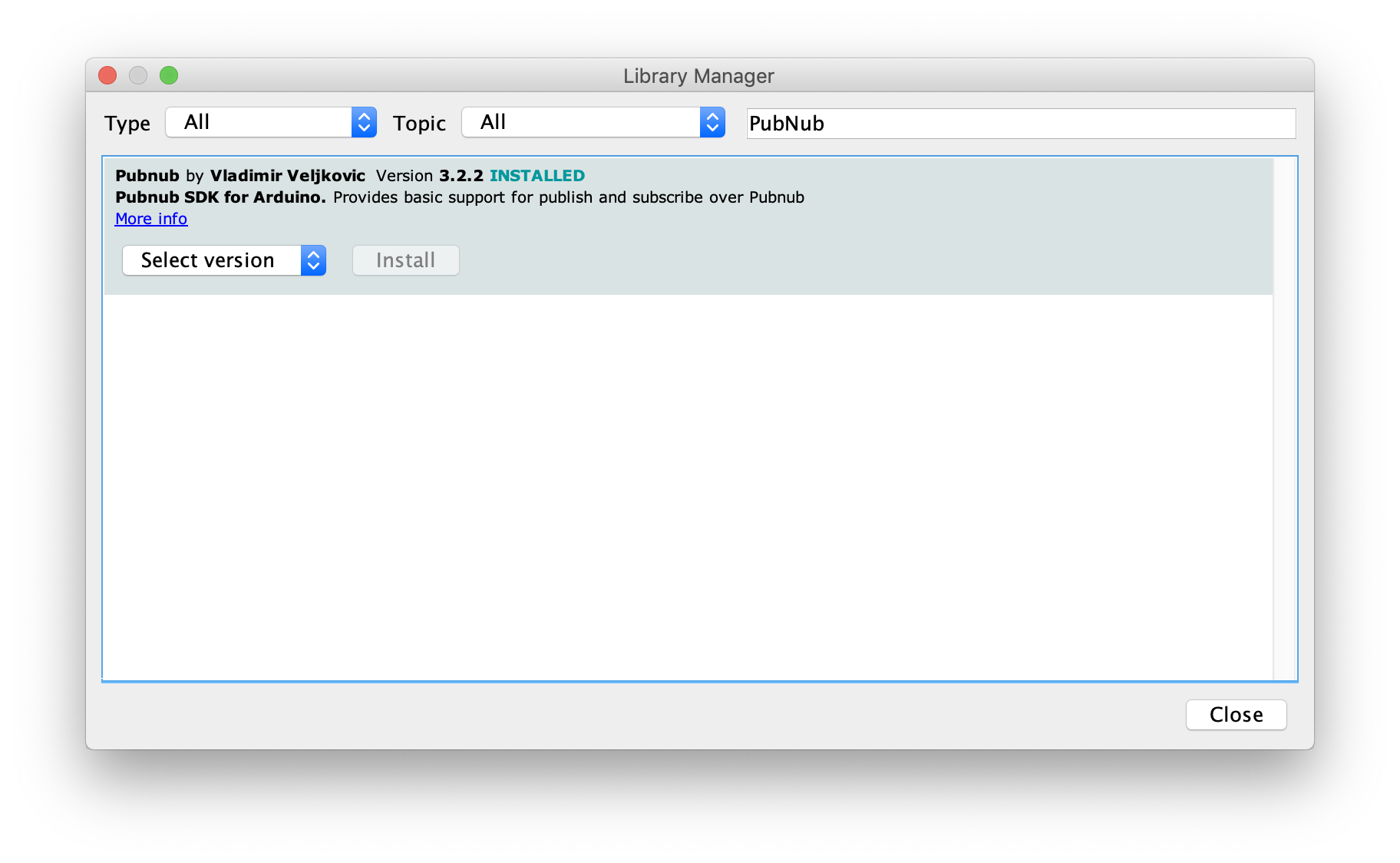
Install Arduino PubSub Client for MQTT
There are many MQTT libraries you can pick from. For this tutorial, we’ll use PubSubClient.
Install PubSubClient by going to Sketch > Include Library > Manage Libraries. Search for “PubSubClient”. Then select Install. You may need to scroll down to find it.
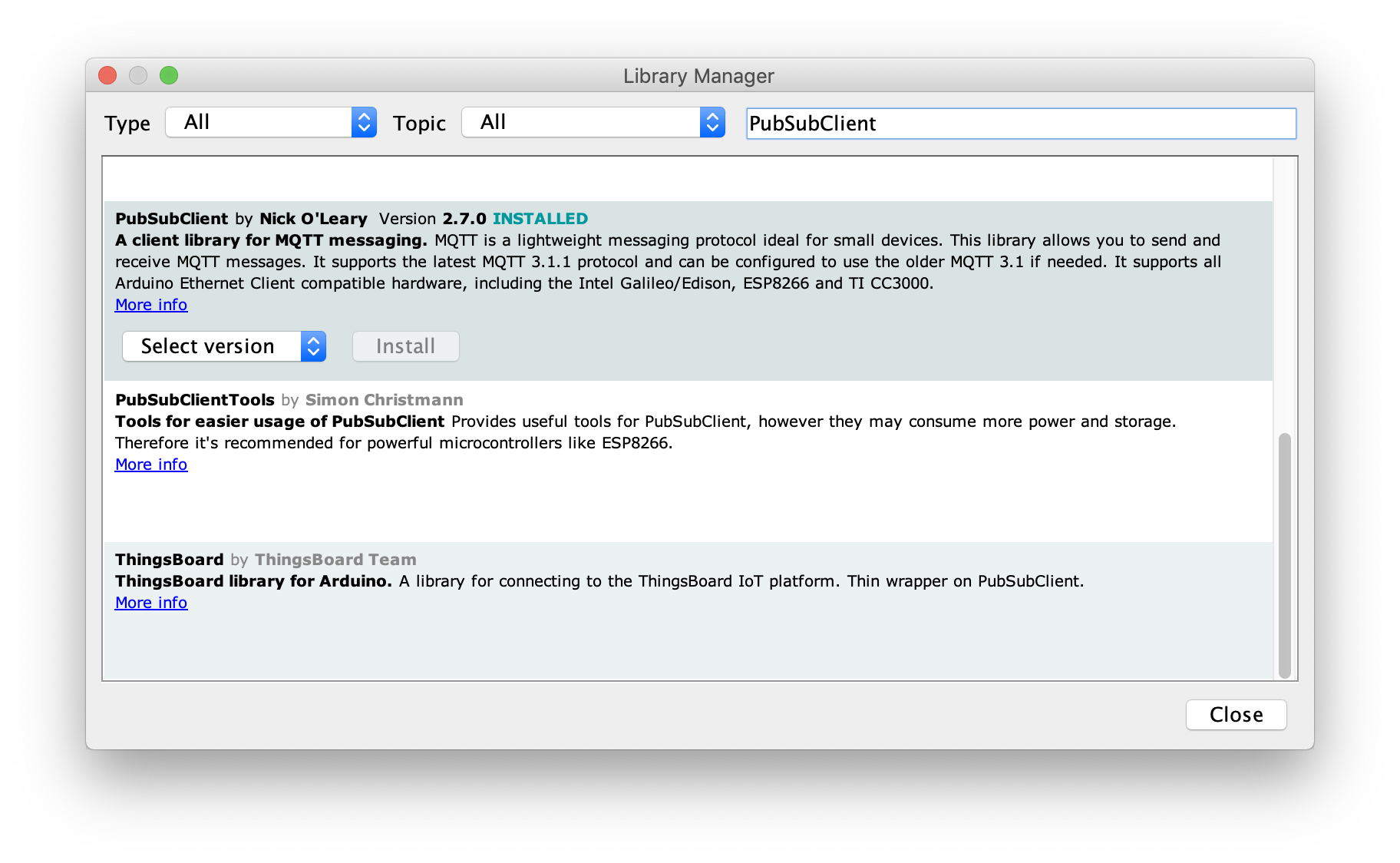
Uploading PubSub Sketch
Now that the Arduino IDE is set up for our development board and we have the required libraries we can flash the board with our sketches.
PubSub with PubNub Arduino SDK
This code uses the PubNub Arduino SDK to send and receive “Hello World!” to a “hello_world” channel. Paste this code into the Arduino IDE.
Be sure that you select the port that your board is connected to under Tools > Port and select the board for use by going to Tools > Boards.
Get your unique, free PubNub keys from the PubNub Developer Portal. If you don’t have a PubNub account, you can sign up for a PubNub account for free. Replace “YOUR_PUB_KEY_HERE” and “YOUR_SUB_KEY_HERE” with your keys.
Replace “YOUR_NETWORK_SSID” and “YOUR_NETWORK_PASSWORD” with your WiFi settings.
Upload the sketch (Sketch > Upload).
// PubNub example using ATWINC1500. #include <WiFi101.h> #define PubNub_BASE_CLIENT WiFiClient #include <PubNub.h> static char ssid[] = "YOUR_NETWORK_SSID"; static char pass[] = "YOUR_NETWORK_PASSWORD"; const static char pubkey[] = "YOUR_PUB_KEY_HERE"; const static char subkey[] = "YOUR_SUB_KEY_HERE"; const static char channel[] = "hello_world"; String message; void setup() { Serial.begin(9600); Serial.println("Attempting to connect..."); if(WiFi.begin(ssid, pass) != WL_CONNECTED) { // Connect to WiFi. Serial.println("Couldn't connect to WiFi."); while(1) delay(100); } else { Serial.print("Connected to SSID: "); Serial.println(ssid); PubNub.begin(pubkey, subkey); // Start PubNub. Serial.println("PubNub is set up."); } } void loop() { { // Subscribe PubSubClient* sclient = PubNub.subscribe(channel); // Subscribe. if (0 == sclient) { Serial.println("Error subscribing to channel."); delay(1000); return; } while (sclient->wait_for_data()) { // Print messages. Serial.write(sclient->read()); } sclient->stop(); } { // Publish char msg[] = "\"Hello world\""; WiFiClient* client = PubNub.publish(channel, msg); // Publish message. if (0 == client) { Serial.println("Error publishing message."); delay(1000); return; } client->stop(); } delay(1000); }
Open the serial monitor (Tools > Serial Monitor) to see messages being received.
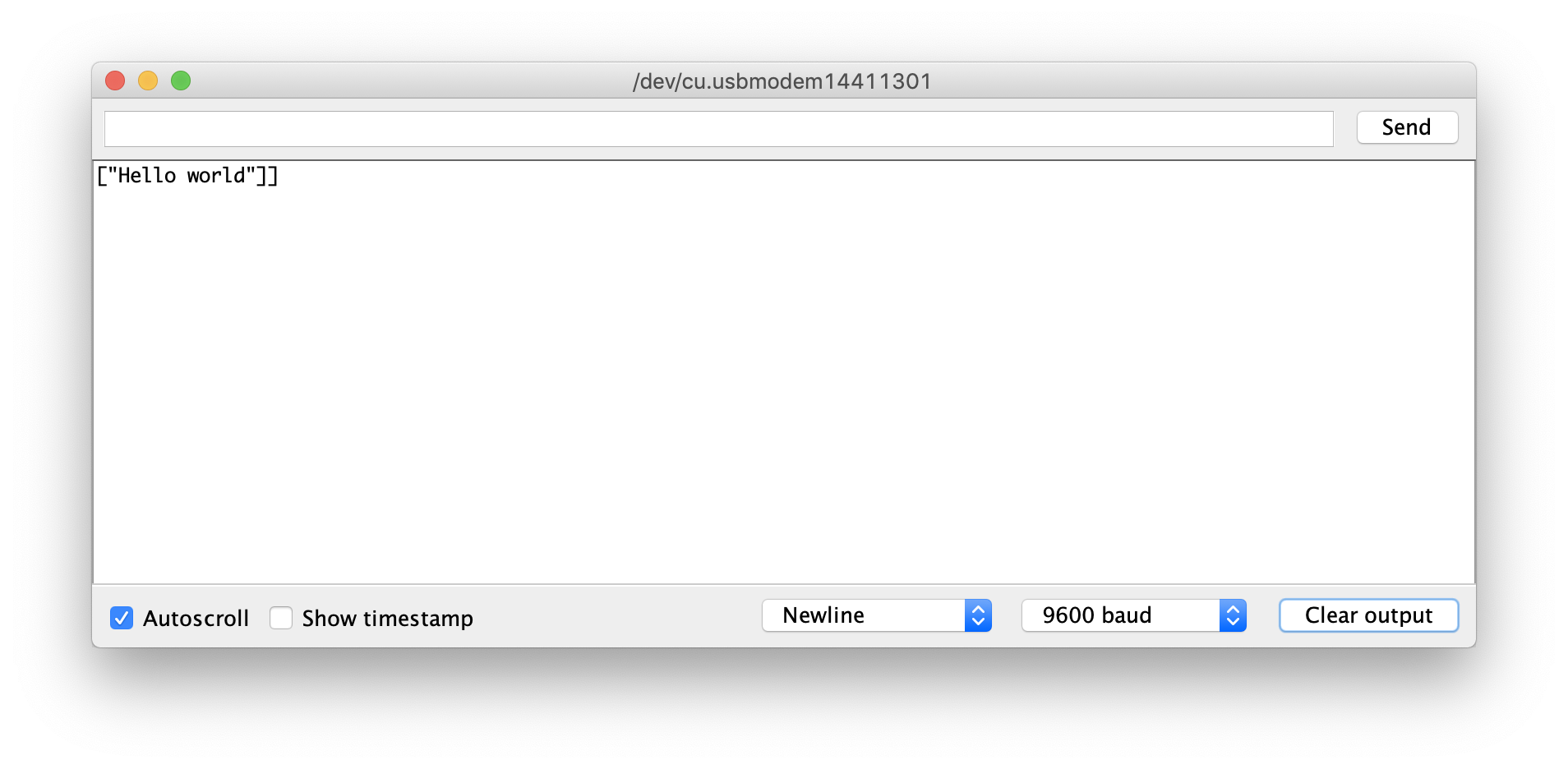
PubSub with PubNub MQTT Gateway
This code uses the PubNub MQTT gateway to send and receive “Hello World!” to a “hello_world” channel. Paste this code into the Arduino IDE.
Be sure that you select the port that your board is connected to under Tools > Port and select the board for use by going to Tools > Boards.
Get your unique PubNub keys from the PubNub Developer Portal. If you don’t have a PubNub account, you can sign up for a PubNub account for free. Replace “YOUR_PUB_KEY_HERE” and “YOUR_SUB_KEY_HERE” with your keys.
“CLIENT_ID” is the actual device ID and identifies the connection to PubNub. This should be unique to each client and you can set it to whatever you want.
Replace “YOUR_NETWORK_SSID” and “YOUR_NETWORK_PASSWORD” with your WiFi settings.
Upload the sketch (Sketch > Upload).
// PubNub MQTT example using ATWINC1500. #include <WiFi101.h> #include <PubSubClient.h> // Connection info. const char* ssid = "YOUR_NETWORK_SSID"; const char* password = "YOUR_NETWORK_PASSWORD"; const char* mqttServer = "mqtt.pndsn.com"; const int mqttPort = 1883; const char* clientID = "YOUR_PUB_KEY_HERE/YOUR_SUB_KEY_HERE/CLIENT_ID"; const char* channelName = "hello_world"; WiFiClient MQTTclient; PubSubClient client(MQTTclient); void callback(char* topic, byte* payload, unsigned int length) { String payload_buff; for (int i=0;i<length;i++) { payload_buff = payload_buff+String((char)payload[i]); } Serial.println(payload_buff); // Print out messages. } long lastReconnectAttempt = 0; boolean reconnect() { if (client.connect(clientID)) { client.subscribe(channelName); // Subscribe. } return client.connected(); } void setup() { Serial.begin(9600); Serial.println("Attempting to connect..."); if(WiFi.begin(ssid, password) != WL_CONNECTED) { // Connect to WiFi. Serial.println("Couldn't connect to WiFi."); while(1) delay(100); } client.setServer(mqttServer, mqttPort); // Connect to PubNub. client.setCallback(callback); lastReconnectAttempt = 0; } void loop() { if (!client.connected()) { long now = millis(); if (now - lastReconnectAttempt > 5000) { // Try to reconnect. lastReconnectAttempt = now; if (reconnect()) { // Attempt to reconnect. lastReconnectAttempt = 0; } } } else { // Connected. client.loop(); client.publish(channelName,"Hello world!"); // Publish message. delay(1000); } }
Open the serial monitor (Tools > Serial Monitor) to see messages being received.
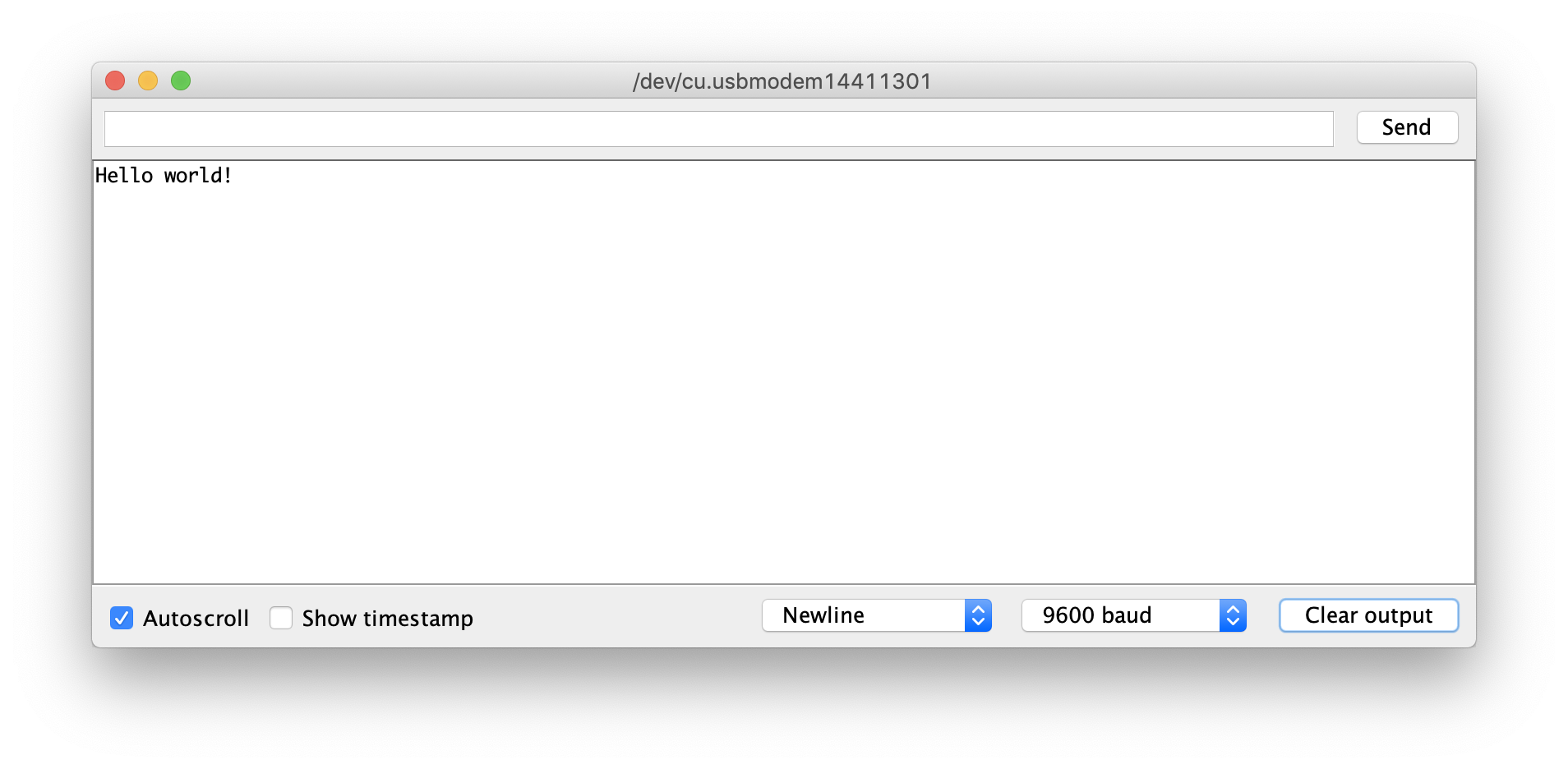
More IoT Resources
- How Industrial IoT and PubNub Can Be the Future of Predictive Maintenance
- The Future of IoT
- IoT Solution Overview
- IoT Machine Learning and Predictive Maintenance Webinar
- ExecTALK: IoT in an Event-Driven Software Development World
- Cloud State Machines: The Future of IoT and Edge Computing
Have suggestions or questions about the content of this post? Reach out at devrel@pubnub.com.