Multiplayer Gaming Basics Using Phaser – Occupancy Counter
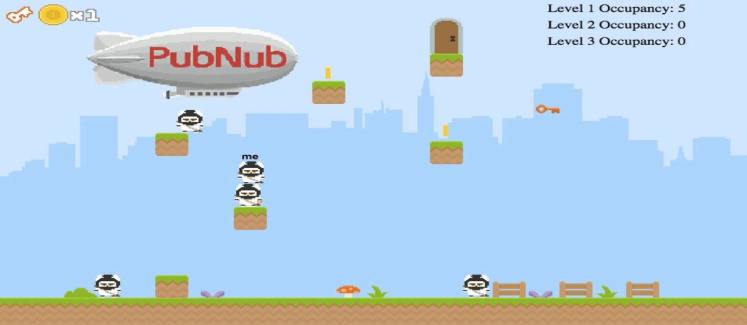
This is a detailed look at the concepts and code for an occupancy counter for multiplayer games, part of our comprehensive series on building a JavaScript multiplayer game.
When creating your own multiplayer game, keeping track of how many concurrent users are in a channel (connected to a game) is important for both end users, as well as your own analytics. For example, when the player can see in real time how many players are in the lobby, it gives them the incentive to invite their friends to join. Or for apps like HQ, you can show how truly successful your game is when 700k+ players are connected in a single game.
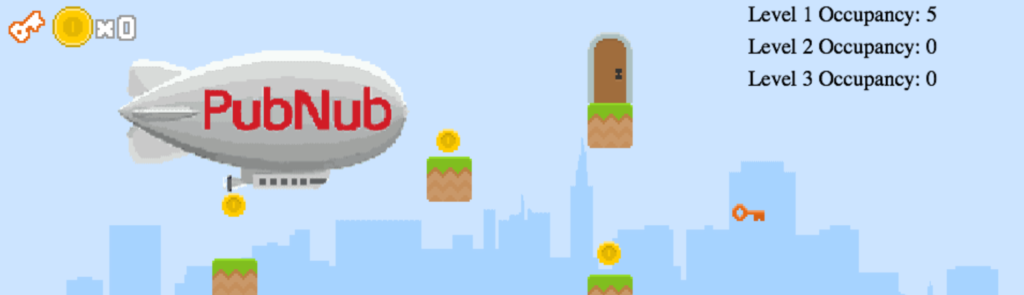
For this quick tutorial, we will be using the Phaser API and the PubNub Javascript SDK. We’ll utilize PubNub Presence, which allows you to get a count, in real time, of how many users are online, and updates when new users connect or disconnect. We’ve got a deep dive multiplayer game tutorial here, but in this part, we’ll flesh out occupancy counter concepts in greater detail.
The first step is to create your text variables which will display to the end user that 0 users are subscribed to each channel. In this example, we have three levels. We want to show the player occupancy for each stage so that players will be incentivized to advance in the game if they see that their friends are on the next level.
window.text1 = 'Level 1 Occupancy: 0'; window.text2 = 'Level 2 Occupancy: 0'; window.text3 = 'Level 3 Occupancy: 0';
Using Presence
The below function checks to see if the hero has joined the stage successfully in (window.globalOtherHeros && checkIfJoined === true)
. The checkFlag()
function is run every time someone joins, leaves or times out of a channel. Once we determine that someone has successfully joined, we run a PubNub Here Now function. Here Now checks who, and how many users are subscribed to a particular channel.
You can learn more about Here Now method here.
presence(presenceEvent) { // PubNub on presence message / event let occupancyCounter; function checkFlag() { // Function that reruns until response if (window.globalOtherHeros && checkIfJoined === true) { window.pubnub.hereNow( { includeUUIDs: true, includeState: true }, (status, response) => { // If I get a valid response from the channel change the text objects to the correct occupancy count if (typeof (response.channels.realtimephaser0) !== 'undefined') { textResponse1 = response.channels.realtimephaser0.occupancy.toString(); } else { textResponse1 = '0'; } if (typeof (response.channels.realtimephaser1) !== 'undefined') { textResponse2 = response.channels.realtimephaser1.occupancy.toString(); } else { textResponse2 = '0'; } if (typeof (response.channels.realtimephaser2) !== 'undefined') { textResponse3 = response.channels.realtimephaser2.occupancy.toString(); } else { textResponse3 = '0'; } window.text1 = `Level 1 Occupancy: ${textResponse1}`; window.text2 = `Level 2 Occupancy: ${textResponse2}`; window.text3 = `Level 3 Occupancy: ${textResponse3}`; window.textObject1.setText(window.text1); window.textObject2.setText(window.text2); window.textObject3.setText(window.text3); } ); } } }
When we get a response from the HereNow function, we update each text object to the correct score count.
And that’s it folks! It’s pretty simple to add a real-time updating occupancy counter using PubNub Here Now and Presence. Contact me at schuetz@pubnub.com if you have any further questions or, and check out our full multiplayer game tutorial here.