How to Use Slack Incoming Webhooks from a Function
This tutorial explains how to post messages to Slack from a Function using Slack Incoming Webhooks. Using Incoming Webhooks, you can create notifications or alerts and even make them interactive. You can use Incoming Webhooks to send an alert when a user has paid, an error occurred, or if a service isn’t responding. You can also use Incoming Webhooks to ask if a user has permission for a resource with the ability to respond from Slack.
What are Functions?
Functions is a serverless environment for executing functions. Functions are JavaScript event handlers that can be executed on in-transit PubNub messages or in the request/response style of a RESTful API over HTTPS.
Advantages of Functions:
- Reduced Cost: Pay for what you use and not for idle servers.
- Decreased Time to Market: Focus on building your product and not managing the infrastructure to support it.
- Reduced Latency: Your users are global. Your product should be global. PubNub operates points of presence across the globe.
Getting Started
Check out the Slack Incoming Webhook Block for a faster way to get started.
- You’ll need a Slack account and a workspace.
- Follow Slack’s documentation for creating an Incoming Webhook. Configure the Incoming Webhook settings to your preferences.
- You’ll need to sign up for a PubNub account. Once you sign up, you can get your unique PubNub keys from the PubNub Admin Dashboard. Sign up using the embedded form below.
Setting up a Slack Incoming Webhook Function
Go to your PubNub Admin Dashboard and select your app. Then click on the keyset that was created for that app.
Next, click “Functions” in the sidebar and then create a new module named “Slack Incoming Webhook.”
Create a Function with the name “Slack Incoming Webhook.”
Set the event type to “On Request”, and set the path to “slack-post”. You can use the other event types if you want to post to Slack before or after messages are sent on PubNub.
Delete any code in the editor and replace it with a function to post to Slack:
// FOR A PUBNUB SERVERLESS ENV. // Learn More: https://api.slack.com/incoming-webhooks export default (request, response) => { const xhr = require('xhr'); // Message let message = `Hello World!`; // Slack Webhook URL const url = "SLACK-WEBHOOK-URL-HERE"; // Basic Slack Post const http_options = { "headers": { "Content-Type": "application/json" }, "method": "POST", "body": JSON.stringify({ "text": message, }) }; return xhr.fetch(url, http_options).then((x) => { //console.log(body); return response.send(); }); };
Replace ‘SLACK-WEBHOOK-URL-HERE’ with your Incoming Webhook URL and edit the message text to be whatever you want.
How to Test the Slack Incoming Webhooks Function
If you set the event type to “On Request”, then you can test the function by making an empty request to the function.
You can use Curl to send a request to the function. Replace “YOUR_SUB_KEY_HERE
” with your Subscribe Key from your PubNub Admin Dashboard.
curl https://pubsub.pubnub.com/v1/blocks/sub-key/YOUR_SUB_KEY_HERE/slack-post
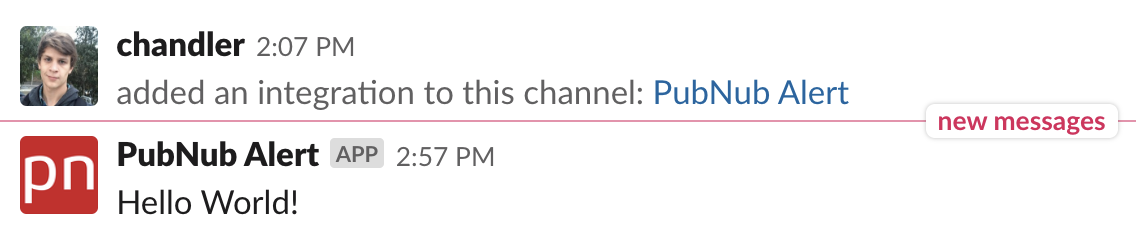
What’s Next?
Here are a few ideas:
Have suggestions or questions about the content of this post? Reach out at devrel@pubnub.com.