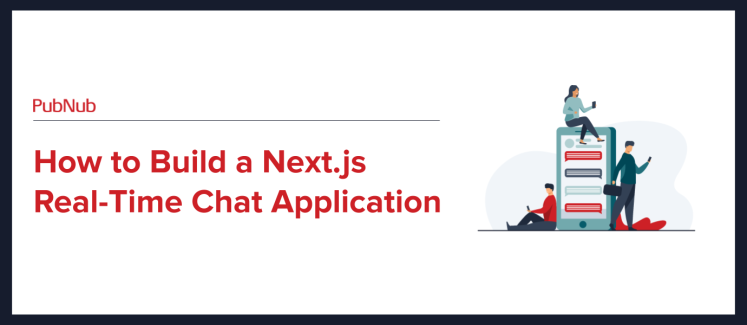
DIY Build a full-stack chat app with ease
Welcome to our tutorial on building a real-time chat application using Next.js and PubNub. This guide will demonstrate how to create a highly interactive chat app with a seamless user experience. By leveraging the power of Next.js, a popular framework built on top of Node.js, alongside the real-time infrastructure capabilities of PubNub, you'll be able to easily develop a full-stack instant messaging app. Let's begin!
Why Use Next.js to Build Chat Apps
Next.js is an open-source, robust JavaScript framework based on React that simplifies the development of server-rendered React applications. It is ideal for building chats software, offering several benefits that streamline the development process.
Benefits of Using Next.js for Real-Time Chat
Server-side rendering: Next.js supports server-side rendering (SSR) out of the box, improving your chat app's performance and SEO. SSR allows your app to load quickly and efficiently, even on slow connections.
Automatic code-splitting: Next.js automatically optimizes your chat app by splitting the code into smaller chunks (code blocks), so users only load the necessary parts of the application, enabling lazy load (defer loading). This ensures a faster, more responsive experience.
Integrated development environment: With Next.js, you can use your preferred tools, such as CSS-in-JS, TypeScript, or GraphQL. This flexibility allows you to create a chat app that best suits your needs.
Real-time updates: When paired with PubNub, Next.js enables real-time chat functionality with low latency. PubNub integration allows chat users to communicate instantly. PubNub's chat API facilitates the implementation of features like user management, push notifications, message history, and presence detection.
Easy deployment: With minimal configuration, Next.js apps can be deployed to various cloud hosting platforms, such as AWS, Vercel, or Netlify. This ease of deployment allows you to focus on building your chat app rather than worrying about infrastructure.
By using Next.js for your chat app, you'll benefit from its powerful features and seamless integration with PubNub for building a real-time chat application that is both performant and scalable.
How to Build a Next.js Real-Time Chat Application
Set up the Next.js project: First, you must create a new Next.js application using create-next-app
.
Install PubNub SDK: To integrate PubNub's real-time functionality into your chat app, you'll need to install the PubNub JavaScript SDK using npm. Run the following command to add it to your project:
Configure PubNub: Next, set up your PubNub account to obtain your API keys. You can sign up for a free account here. Once you have your API keys, store them in your .env file:
Initialize the PubNub Object: it will allow us to send and receive messages across channels.
Create chat components: Now, it's time to build the frontend components for your chat app. Using React Hooks and CSS, you'll create components for the chat input, and message list. The useState and useEffect hooks will handle the state management and side effects of your components.
Chat Input Component: For users to type and send messages.
Chat Messages Component: To display incoming messages.
Implement real-time chat functionality: With these components in place, you have already integrated PubNub's real-time chat functionality using the PubNub SDK to send messages and subscribe to channels (chat groups & threads).
Implement authentication (auth): To secure your chat app, you must implement user authentication. You can use various authentication methods, such as JWT tokens or OAuth providers like Google or Facebook. Remember to secure your server-side endpoints and client-side components with proper authentication checks.
Style the chat app: Finally, you can customize the appearance of your chat app using CSS or any preferred styling approach. You may also use component libraries like Material-UI or Bootstrap to speed up the process.
With these steps completed, you'll have a fully functional real-time chat application built on Next.js and powered by PubNub. You can find additional resources, source codes, and examples in our documentation to help you add more features and polish your chat app.
Next.js Chat App Examples
To inspire your chat app project, here are a few examples of real-time chat apps built using Next.js and PubNub. These examples showcase the versatility and scalability of Next.js when combined with PubNub's real-time messaging capabilities.
Group Chat App: A group chat application where users can join different chat rooms, send messages, and see who's online. You can find a tutorial on how to create a similar app using Node.js and PubNub here.
Web-Based Chat App: A web-based chat application that allows users to send messages and share files with others. This app demonstrates integrating real-time chat functionality into a web app using PubNub. Check out the tutorial here.
Chatbot App: A chat app that integrates an AI-powered chatbot, such as OpenAI's GPT-3, to answer user queries and provide assistance. You can find a tutorial on building a chatbot app with GPT-3 and PubNub here.
Customer Support Chat App: A customer support chat application that connects users with support agents in real-time, allowing them to resolve issues quickly and efficiently. This app showcases PubNub's real-time messaging and presence features in a customer support context.
Next.js and PubNub can create chat apps tailored to different use cases, ranging from simple messaging platforms to sophisticated AI-driven chatbots. These examples serve as a starting point for your own Next.js chat application. Happy coding!
Develop Your Chat App with PubNub
To get started with PubNub for your real-time chat application, follow these steps:
Sign up for a PubNub account: First, sign up for a free PubNub account to obtain your API keys. Your API keys are essential for integrating PubNub's real-time messaging services into your chat app.
Explore the PubNub documentation: Familiarize yourself with PubNub’s documentation to better understand the different features, services, and SDKs available to you. The documentation provides comprehensive guides and tutorials to help you successfully integrate PubNub into your chat app.
Choose a PubNub SDK: PubNub offers SDKs for various languages and platforms, including JavaScript, Node.js, React, Angular, and many more. For your Next.js chat app, you'll want to use the PubNub JavaScript SDK.
Integrate PubNub into your chat app: Once you've chosen the appropriate SDK, follow the quick start guide to integrate PubNub into your chat app. You'll need to initialize the PubNub instance with your API keys and then implement the necessary functions, such as sending messages, subscribing to channels, and managing user presence.
Test your chat app: As you build your chat app, test it thoroughly to ensure that the real-time messaging functionality works as expected.
Deploy your chat app: Once you've built and tested your chat app, deploy it to your preferred cloud hosting platform. Next.js apps can be deployed to various platforms like Vercel, Netlify, or AWS.
By following these steps, you'll be on your way to creating a robust, real-time chat application powered by Next.js and PubNub. Refer to the PubNub docs or github for additional guidance and support throughout your development journey.