Building a Collaborative Real-Time Android Todo App
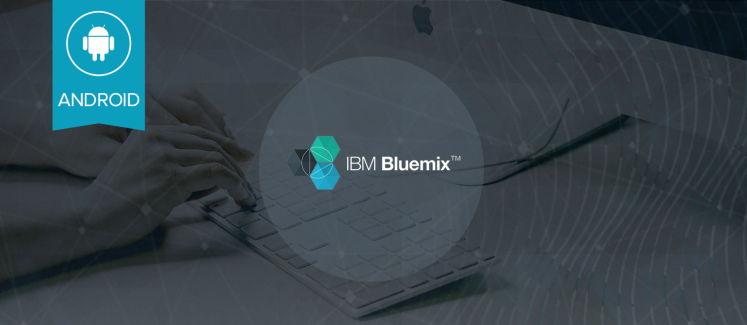
Let’s take a simple todo list and make it multi-user, allowing any number of connected users to collaborate with it in real time. In this tutorial, we show you how to build and deploy a real-time Android todo app using the IBM Bluemix and PubNub.
Building an app is one small piece of the puzzle, but like all apps these days, if your app also relies on back-end content and logic, then hosting it and ensuring that it serves the users well might not always be a cakewalk. We show you some innovative ways in which you can reliably host your app under the Bluemix umbrella of services and reduce the overhead on the part of the developer.
Android Todo App Overview
The real-time todo Android app that we are going to build here is a project collaboration app where multiple users, all belonging to the same project team, will log in and add or update todo items or tasks. They will be able to create tasks and also post comments on other’s tasks. Tasks can also be marked with status to indicate whether they are open or complete.
Source code:
The complete source code for the application, including the app and server, is available in the Real-time Android Todo App Github Repo.
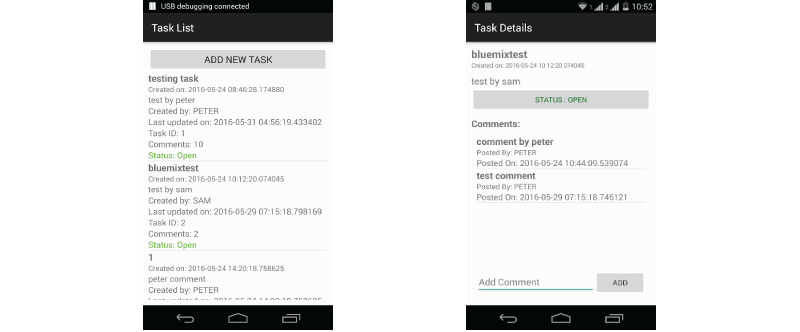
Ditching REST for Bidirectional
A large number of mobile apps built today rely on server side business logic and data persistence for achieving their functionality. Representational State Transfer (REST) has emerged as the most popular way of enabling interaction between a client application and servers. REST offers a more flexible URL scheme based on objects, their behavior, and their states, compared to the earlier interpretation based on static file paths (during the Web 1.0 days).
While it is indeed a great leap, REST is still based on the HTTP protocol. The one fundamental flaw of HTTP is that it is a request-response protocol. HTTP was designed to be that way so that the client could request resources from the server and server return that as a response. It was never designed for bidirectional, duplex, and conversational communications, which we have come to rely on so much for all modern applications these days.
For powering this Android todo app, we are going to use an alternative approach based on PubNub and the IBM Bluemix umbrella of services, which can eliminate some of the limitations of HTTP and make the applications more aware and responsive.
For this todo app, this can be in the form of real-time task/comment updates or instant task notifications between the apps. All of this can be achieved without any workload on the application developer. In fact, building and deploying a real-time mobile app under Bluemix is manyfold simpler compared to the traditional approach. So let’s get started!
Powered by Bluemix, Driven by PubNub
If you have ever deployed a RESTful service on your own, then you would know that this requires setting up a web server as an HTTP API gateway to handle all HTTP/REST requests from the client. Behind the scenes, we also need to set up a few application servers to handle different micro services and their business logic for each REST endpoint.
This leads to a two-tiered architecture that looks something like this:
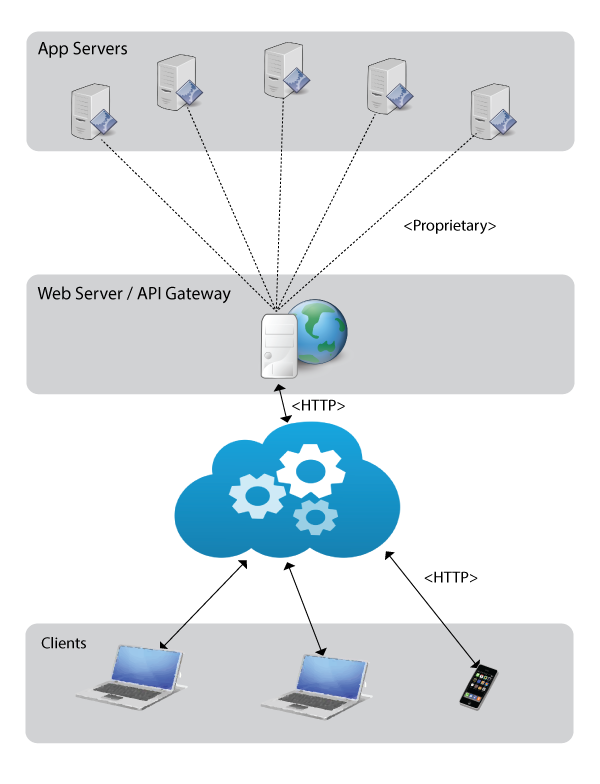
For deploying the server-side architecture of this todo application, we can simplify it. The heavy lifting of connecting the client Android app with the server is done with PubNub.
PubNub is a third-party service available under the Bluemix catalog, which provides a messaging layer over the Internet for enabling bidirectional communication between multiple entities in real time and in a secured and reliable fashion. This way, the API gateway and app servers can be collapsed in a single tier like this:
This way, the API gateway and app servers can be collapsed in a single tier like this:

In this way, the server-side application for this Android app can be hosted on a Bluemix container, either the Cloud Foundry runtime or a docker container. We have chosen the Cloud Foundry runtime with Python Buildpack as our server environment, which means that the server application is built on Python.
Behind the scenes, all messages exchanged between the client app and server are routed through the PubNub Data Stream Network, via a channel, which acts like a secured virtual tunnel, akin to a VPN connection. We can create multiple channels designated for each type of operation, which is the same as defining different REST API endpoints for invoking a different set of business logic.

By using this approach, we can offload all our platform and messaging requirements to Bluemix and PubNub and deploy real-time applications within a single tier. We only need an extra component, which is a database for storing the task data. We have used IBM dashDB™ for this purpose, which is also available as Bluemix service.
Hooking an application to PubNub is easy. You do not have to make any HTTP REST API calls to the PubNub service. Instead, you can use one of the 70+ SDKs available for embedding PubNub messaging in different languages and frameworks. For this application, we used the following PubNub SDKs:
- PubNub Python SDK: For the app server
- PubNub Android SDK: For the mobile app
Project Setup
- Source code for the app server: Located under the server folder in the GitHub repository.
- Source code for the ToDo app: Located under Android-Client folder in the GitHub repository. (You can build the ToDo App using the standard Android build procedure.)
- README file: Contains steps for hosting the app server on Bluemix and using the mobile app.
Before deploying your application, make sure to sign up for a Bluemix and PubNub account. Visit the Bluemix registration page and PubNub add-on page to create your respective accounts. Both of the services offer a free tier account to play around with their offerings.
App Operation
There are seven operations defined for this app:
- Login: For allowing the user to log in to the app
- Logout: For allowing the user to log out the app
- Get Address Book: For getting all the users in the project
- Get Task List: For getting the list of tasks
- Create Task: For creating a new task
- Update Task: For updating the status of task
- Add Comment: For adding a comment to a task
As explained earlier, the architecture of this application is defined in such a way that every app operation is an endpoint that is assigned a separate PubNub channel. This helps in segregating the messages and their business logic based on the functionality.

Client-Server Interaction
All operations are performed as a request-response transaction between the server and app. When the app is launched, it follows a sequence of operations to perform a common set of procedures based on the user inputs as depicted in the sequence diagram below.

The app also provides basic security features to ensure that a logged in user cannot log in again from another app instance, and the user session is tracked for dormancy, forcing the user to log in again if it is detected.
Application Security
Security is a major concern for any application involving client-server interaction. For this ToDo app, because all message exchanges happen through the PubNub channels, that is the place where security can be compromised. PubNub has put in place a robust set of measures for ensuring security, which can be leveraged by application developers for making their apps secure. This can be in the form of:
- Access Control: PubNub allows fine-grained control over the access to channels. This means that the developer can decide which device can have access to the channel, can read and/or write to the channel, and for how long.
- Message encryption: PubNub supports a built-in AES encryption and optional TLS/SSL support for all its APIs. The encryption keys are owned by the application developer, which ensures end-to-end fullproof encryption under the control of the application developer.
Advantages Over Standard REST-based Implementation
Developers always have a choice to build their app using REST and develop and deploy all the supporting components on their own. But the effort of undertaking such an exercise gets exponentially high as the application scales up. Compared to that, here are some of the advantages of using Bluemix and PubNub for deploying your apps:
- Bidirectional: PubNub APIs are bidirectional, meaning that both endpoints of the channel can communicate in either direction. This also means that the server can push a message to the client without the client sending a request or initiating a
REST Will Be History
One of the common performance issue with REST call is that it always opens a new connection for every request and then closes the connection after getting back the response. Doing this frequently is an obvious performance hit for modern web and mobile apps that rely on frequent, bidirectional communication.
PubNub has engineered it in a way to allow massive scalability with a robust back-end infrastructure to support real-time and always on messaging applications.
PubNub provides SDKs for integrating with all the programming language build packs supported under Bluemix. Just like we have seen for Python in the case of this app, there are PubNub SDKs available for some of the most popular web and mobile app platforms such as Java™, Ruby, Go NodeJS, and ASP.NET.
This opens up a huge opportunity for developing state of the art, real-time, and super alert applications that can not only be deployed under Bluemix with ease, but can also scale with ease.
Stay tuned for more demos and application use cases using Bluemix and the PubNub Data Stream Network!