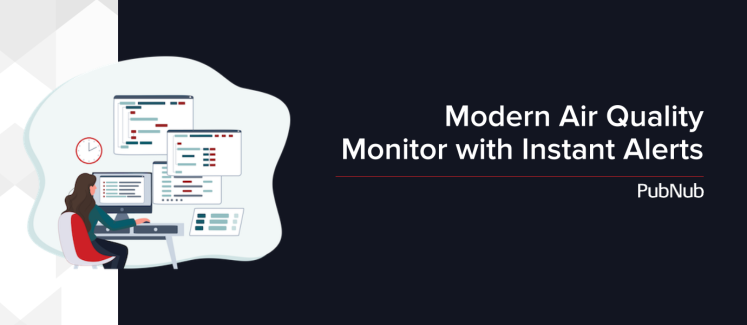
The World Health Organization estimates that 4.2 million people die each year from causes directly attributed to air pollution. With that in mind, even being aware of air particulates and toxins wage a difficult battle could help reduce the 7 million premature deaths every year from exposure to air pollution. Many people often don’t even realize that their own home’s indoor air quality is polluted.
However, in this day and age of powerful IoT technologies, any person can combat bad air quality. There are devices specifically made to detect particulate matter (PM) using PM sensors and CO2 sensors, send the information to a central hub using Bluetooth or WiFi, and display this data to users through devices such as home assistants like Amazon Alexa. Air purifiers are also used to help combat poor air quality by filtering the polluted air.
If you want to create your own DIY air quality sensor and monitoring system, sensors and microcontrollers have gotten so cheap, small, and simple to implement, that practically anyone can install any type of monitor or sensor in their very own home. Among other possibilities, these sensors can trigger things like alerts and notifications to spur user action.
In this tutorial, you'll learn how easy it is to create an air quality sensor and monitoring system that obtains air quality data using a few low-cost electronics and Functions. The sensor will extract air quality information with multiple sensors, graph that data in real time, and even set off a speaker alarm if the air quality reaches a harmful critical point, effectively creating your own DIY air quality monitor.
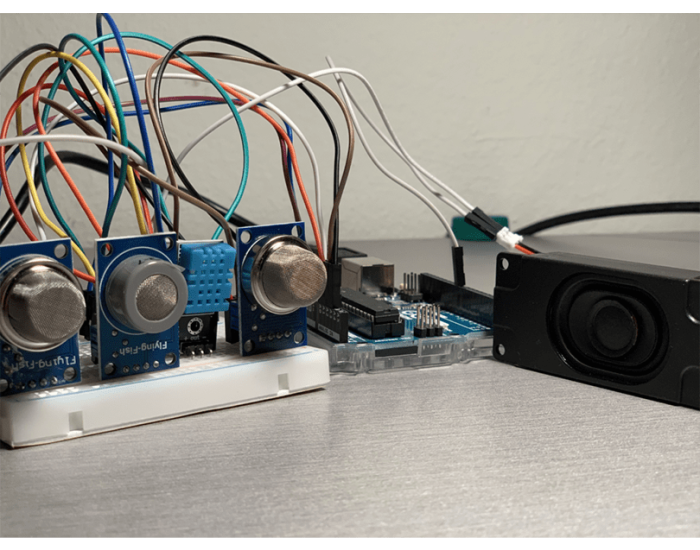
Required hardware for DIY air quality testing
Listed below are the required materials needed to complete this tutorial. You can purchase this hardware from the links provided below or from online retailers such as Amazon and AliExpress.
Arduino Uno
Arduino Uno is one of the cheapest open-source microcontrollers available today. Since the Uno has an onboard analog-to-digital converter adapter, the analog signals produced from the sensors used in this tutorial can be properly read.
Breadboard
A breadboard is a tool used to prototype and test circuits. Breadboards have two long rails (red rail for power and blue rail for ground) on each of the sides that are each connected vertically down the board. This is where engineers typically plug in a power supply and ground so that the whole rail can be easily used as the power and ground connector. At the center of the board, there are groups of 5-holed horizontal lines. Each of these lines is horizontally connected (5-hole connection) which is used for the circuit itself. You can even connect one grouping to another to make a 10-hole connection and so on and so forth.
You can purchase a breadboard with some wires typically in a bundled kit.
Basic Speaker
A speaker is essentially a piece of vibrating metal that moves up by a magnet when fed power and falls down when unpowered. When the magnet is turned on and off at a specific frequency, a tone can be played. Purchase a cheap speaker from an online retailor mentioned before.
Sensors
Each of the sensors you're going to use throughout this tutorial operates on the same fundamental principle. A specific type of material is placed inside a sensor and an electric current is fed through it. When certain environmental conditions occur that react with the sensor’s specific material, the material’s resistance to the current increases or decreases. The current then will be affected and the sensor can translate that change into proper sensor units.
You'll need to pick up the following sensors needed for this tutorial:
Wiring for air quality sensor system
The wiring of each of these sensors follows the same pattern:
Connect the ground pin of the sensor to the ground of the Arduino.
Connect the power pin of the sensor to the 5.5V pin of the Arduino.
Connect the data pin of the sensor to a GPIO pin on the Arduino.
The sensors were connected in the following schematic.
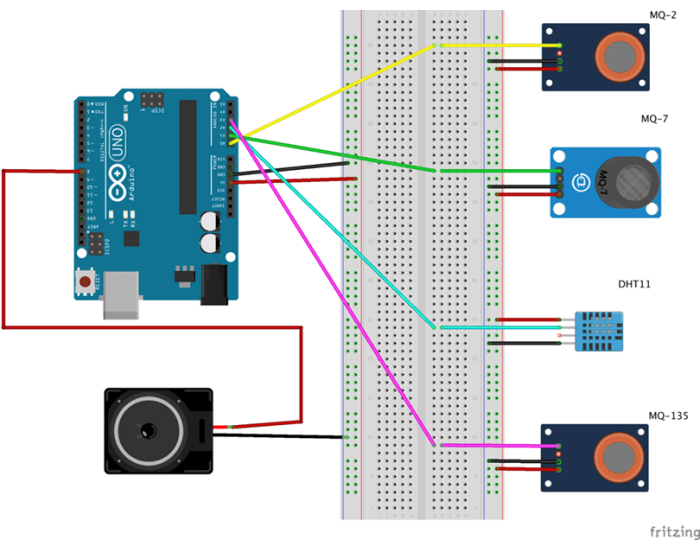
Designing the logic of air quality measuring at home
The code for this logic is set up in three parts: Arduino sketch, publishing code, and graphing HTML code.
The Arduino sketch will handle the direct communication between the sensors and the Arduino.
The publishing code will relay the data received by the Arduino to the PubNub Arduino SDK for publishing, which is used to communicate with PubNub’s APIs.
The HTML code will then visualize the data into a graph in real time on an HTML webpage.
Note: Please note that the PubNub Arduino SDK is no longer officially supported.
Before you begin implementing the code behind, make sure you sign up for a free PubNub account, as you'll need your publish/subscribe keys to send information across the PubNub Network.
Arduino Sketch
For the Arduino code, you will be developing in the Arduino IDE in order to utilize its serial bus capabilities and simple method call structure.
Begin by importing all of the necessary libraries for each of our sensors so you can communicate with them in the Arduino IDE.
To do this, download the .zip file from each library’s GitHub and add them to your Sketch.
GitHub links: DHT11, MQ135, MQ7, and MQ2.
Then you will need to create variables and instances for each of your sensors.
Next, you will need to create a setup function to initialize your Arduino’s baud rate as well as initiate any sensor’s necessary setup modules (in this case the MQ2).
To keep the program continuously running, create a loop method with a delay of one second.
Inside the loop is where you will implement the code to communicate with the sensor’s data. For each sensor, you use the methods defined in their libraries to extract the data from the sensor and format the raw voltage reading to a recognizable unit of measure.
The code for each sensor is as follows:
Note: You need to comment out the serial.print
lines except for temperature and humidity. This makes future steps simpler. But if you’d like to test your devices, uncomment them. You can view the serial prints in the Arduino serial monitor. If you do this, make sure each sensor is publishing to a new line each time.
Next, you'll connect the speaker to the program. As discussed before, you need to implement the speaker to operate at a certain frequency to play a specific tone.
Create a new file named pitches.h
and include the pitches.h file via #include "pitches.h"
.
Next, copy and paste these methods that are responsible for playing a sound using the tone referenced in pitches.h.
Now you can have the sound trigger if any of the sensor values reach above a certain threshold. You will need to create your own critical variables through trial and error as each sensor will come with a noticeable margin of error.
Once you have finished, select your Arduino’s USB port, hit the green checkmark to compile, and the green right arrow to upload it to your connected Arduino.
Tip: You can find the name of the port your Arduino is connected to by going into the top menu: Tools->Port.
Publishing Code
You might be wondering, what is the need to separate the code just to publish messages from the Arduino? Isn't it easier to do it straight from the sketch?
To simplify a complicated answer, the PubNub Arduino SDK requires the use of a WiFi shield to enable Internet capabilities. Since that is out of the scope of this project, we will revert to an alternative method: pySerial.
pySerial is a library that tunes in on the Arduino’s serial monitor. Anything printed over the serial monitor can be read by pySerial. Thus, we can extract the data from the serial bus and publish that data through PubNub.
To install pySerial, type the following command into your terminal.
Then create a python script by giving it a name and include the necessary PubNub libraries to be able to use the PubNub Arduino SDK.
Then you can set up your pySerial port connection.
Next, create the infinite loop of the program that continuously extracts and publishes data. Be careful, as pySerial’s serial bus monitor can only see data coming in serially. It cannot distinguish names or variables, only numbers separated by the new line character.
Since you are going to work with two different sensor readings (temperature and humidity), we can expect the first line of data to be from sensor A, the second by Sensor B, the third by Sensor A, the fourth from Sensor B, and so on.
Going back to the Arduino sketch covered earlier, the temperature sensor is printing first in the code, so you can expect that the pattern will go temperature, then humidity, then repeat. You should capture the data in that pattern.
Next, print the values to the terminal before publishing using PubNub in order to see if you’re getting valid data.
If everything is running smoothly, your terminal should look as follows. Please note that the alternation of data – 29 corresponds to temperature and 34 corresponds to humidity).
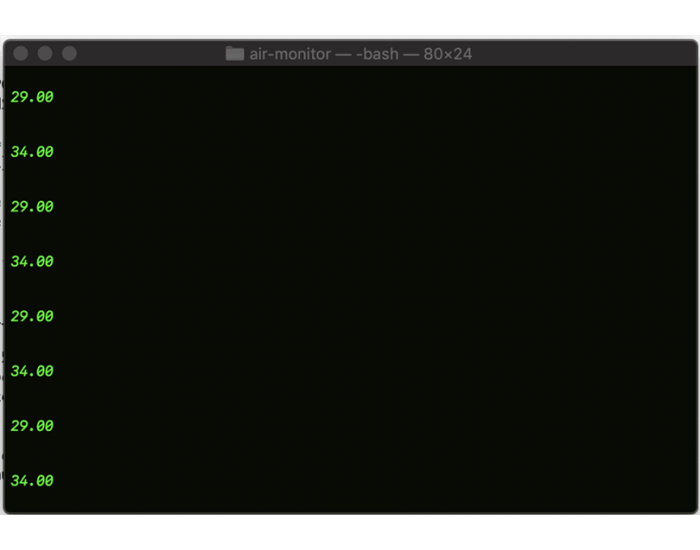
Note: Please note that the rest of the tutorial discusses publishing this data to PubNub's real-time data visualization framework, PubNub EON. PubNub EON and the PubNub EON Chart SDK which is used to communicate with Project EON, are no longer officially supported.
Finally, you're going to publish this data to our real-time data visualization framework: PubNub EON. In order to send the data that EON can parse and graph, you need to format the data first into JSON format.
Then you can publish to the same channel that the chart is subscribed.
HTML Graphical Representation Code
You will now use PubNub EON to display the readings in real time on a live chart.
Then subscribe to the eon-chart channel where you will be posting data.
You now have a connected device collecting and streaming air quality readings and publishing these results to a live dashboard, all in real time. You'll be alerted with a sound to indicate that these thresholds you set up earlier have been reached.
If you would like to learn more about how to power your IoT applications and smart home systems, take a look at our IoT resources.
If you have any other questions or concerns, please feel free to reach out to devrel@pubnub.com.