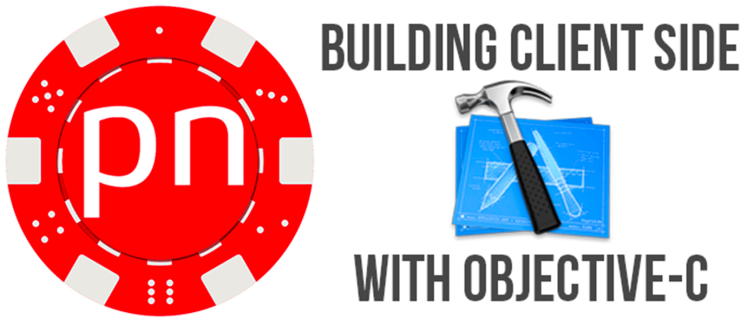
We’ve updated our SDKs, and this code is now deprecated.
Good news is we’ve written a comprehensive guide to building a multiplayer game. Check it out!
This is part one of our two part series on developing an app with a client-server model. You can read the series overview here, or hop on over to Part Two: Developing a C# Game Server here. This two part series on building an iOS app with a client-server model was developed by our two summer interns, Taylor Kimmett and Ajan Jayant.
Developers frequently choose to develop an app entirely in one language, usually because many fear that information can be lost in translation. Will a dictionary in C# be converted into a mutable dictionary in Objective-C? Will Python callbacks behave similarly to ones written using the Android SDK? And if I’m used to writing code for the Android SDK using a specific framework, can I depend on the same functionality if I rewrite the app for the iPhone?
In this tutorial, we’ll walk through how to build the iOS client side for iOS games using Objective-C. In this specific example, we’ll build a iOS poker game.
**NOTE: Since we wrote this tutorial, we’ve released a new, completely-redesigned version of our iOS SDK (4.0). We rebuilt the entire codebase and took advantage of new features from Apple, simplifying and streamlining the SDK.
The code from this tutorial still works great, but we recommend checking out our new SDK. We’ve included a migration guide to help you move from 3.x to 4.0, and a getting started guide to help you create a simple Hello World application in minutes.**
Need for a Language Agnostic Framework
To solve this problem, we need a language agnostic framework, The PubNub Data Stream Network, that has similar behavior across multiple platforms. To examine how well it supports multiple languages, we decided to write an app using the client server model, using different machines, I.D.E.s and people to write each. The server was written in C# to support multiple clients for the iPhone or iPad, which was written using Objective-C.
Server and Client Design
In terms of design, the server and client are very similar. They both listen to PubNub channels (although very different ones), both publish to it and both listen to messages. The client makes heavy use of the didReceiveMessage function, and uses an if-else structure to check the type of message being received. Based on the type of message being received, it loads a view controller, processing the data that the, which gives the user a set of options. After the user selects an option by pressing a button, a message is sent to the server. The client then waits for a response from the server. Pretty much all communication takes this form.
As an example, we’ll look at a small segment of the game where the user calls another user’s bet. The user waits for an update message from the server, which tells him what the previous bet is among other useful message. He also waits for a take-turn message, which then enables his phone buttons which were disabled after his previous turn. He can choose to fold, raise or call; he chooses the latter. A message is then sent to the server. The user then waits for his next turn.
Writing the iOS Client Side
Writing the client was fairly simple. The fact that most of the game processing was taken care off by PubNub, I could focus on the UI design and solving little issues, such as the keyboard freezing if a text field delegated itself. Callbacks in C# behaved exactly as they would in Objective-C. Despite not being fluent in Objective-C, my co-developer on the project Taylor (who developed the C# Server side), with was able to spot bugs in the app simply by looking at the PubNub code. Also, a few functions made our job a whole lot easier: the requestParticipantsListForChannel function allowed me to check server status very easily. This allowed me to halt the game if the server crashed, and made error handling much easier.
Benefits of Using the Client-Server Model
We found that using the client-server model provided significant benefits in terms of usability, design time and performance. It reduced the amount of code the client designer has to write and enables him to focus more on UI design as opposed to data processing. It also allows for added security, enabling only certain data to be visible to the client, making it harder to cheat. Lastly, it also allows for better storage and processing of data, especially if the data is generated from hundreds of users.
Though we only tested C# and Objective-C, the principle of language interoperability with the PubNub framework can be extended to any of the most popular languages (ie. JavaScript, Python and Ruby).
All the code you need to get started right away is in the GitHub repository below.
Get Started
Sign up for free and use PubNub for multiplayer gaming