Create a Chatbot in Under 10 Minutes Using Functions
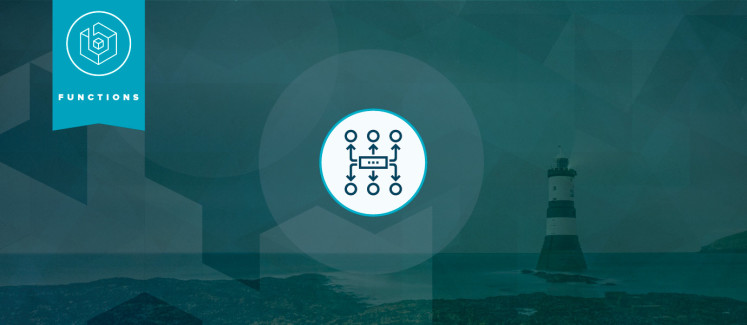
In this tutorial, we will use Functions to create a chatbot for Facebook Messenger in under 10 minutes.
Functions is Functions-as-a-Service backed by a real-time network, for building microservices and webhooks that speed up and simplify the development of modern apps.
We’ll be following Facebook’s quick start guide, but modifying their example code which is written in Express so that it is compatible with ECMAScript 6 and Functions libraries.
Tutorial
A.) First, we need to create a Function
that our Facebook app can call in response to any incoming chat messages. PubNub Endpoints allows us to create a Function
that is a webhook.
Select or create a new App
from your Admin Dashboard. Then, select or create a new Keyset
. Create or select a new Module
. From there, create a new Function
and make sure the event type is On Request
:
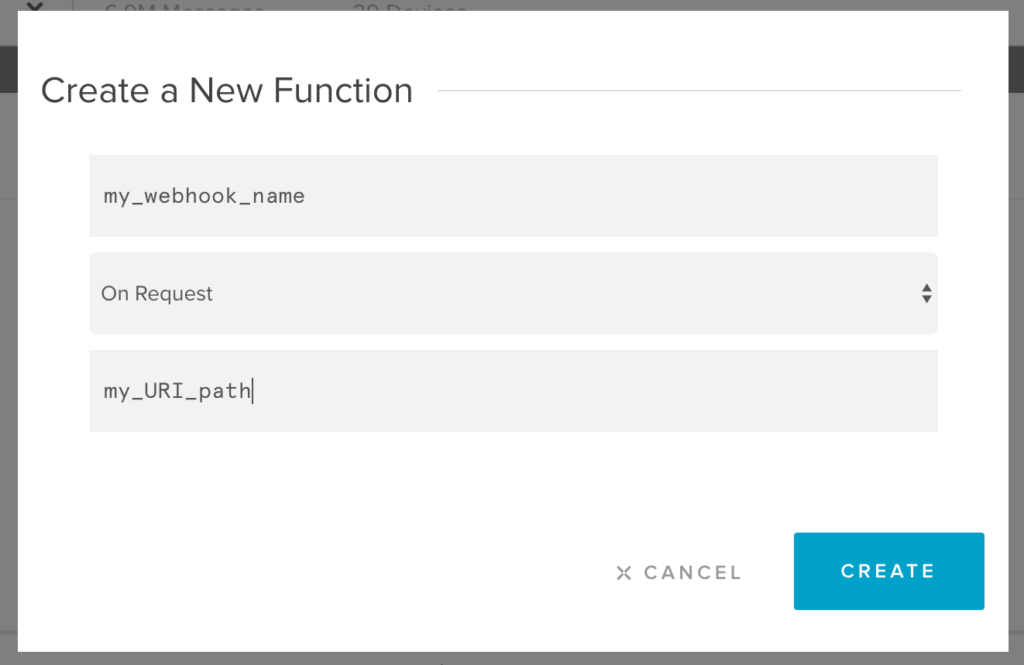
Enter a URI path of your choice and select “Create.” From the Functions editor window, copy your webhook URL by clicking “Copy URL” in the window on the upper right:
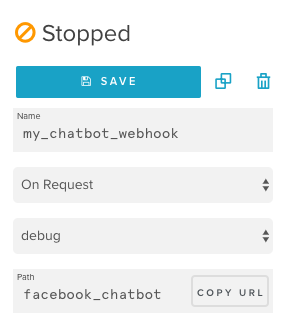
Copy the URL of your URI path.
B.) Go to the Facebook tutorial and follow the first step “1. Create a Facebook App and Page”. On the second step “2. Setup Webhook”, for the Callback URL paste the Functions webhook URL you copied in step A. The Verify Token is any string of your choosing. Make sure to select messages
and messaging_postbacks
under Subscription Fields.
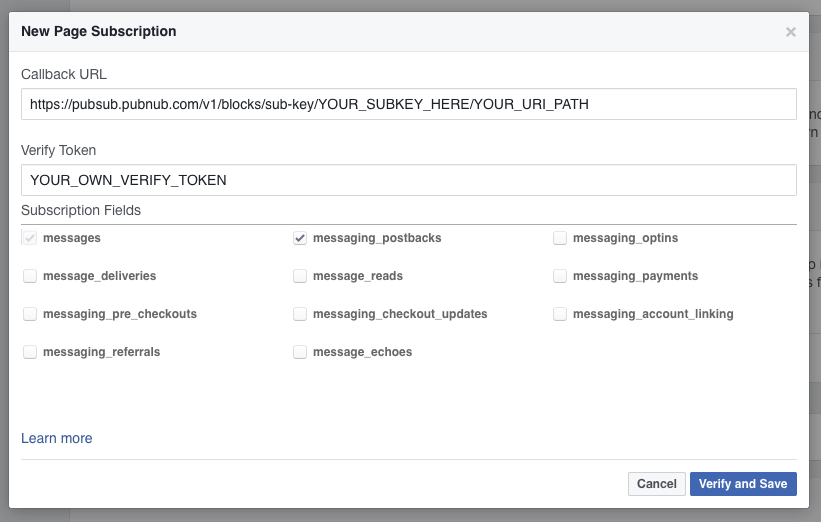
Your window should look similar to this, except with your own Callback URL and Verify Token.
Facebook issues a GET request to your webhook containing the Verify Token as well as a challenge. We need to add code to our function that checks for the verify token and responds with the challenge contained in the verification request:
Your function should look like this so far.In your function, make sure you put your Verify Token where it says let VERIFY_TOKEN = ‘my_verify_token’
, then save your Function and start it. Then in Facebook, hit “verify and save.”
C.) Follow steps “3. Get a Page Access Token” and “4. Subscribe the App to the Page” in the Facebook guide. Update the PAGE_ACCESS_TOKEN
variable in your Function code.
D.) Now we need to code our Function to receive messages from Messenger and respond to them. Facebook makes a POST request anytime a user messages our Page. Add an else condition to the if statement from step B:
For receiving messages, our function will look for the event.message
field and call the receivedMessage
function.
E.) In receivedMessage
, we will echo back to the user the same message that was received:
Make sure all your functions are contained in the overarching export default (request, response) => {}
.
sendTextMessage
formats the data in the request:
callSendAPI
calls Facebook’s Send API:
Save your Function and restart it, then go to your Facebook Page and send a message to it. You should see the message echoed back to you along with output to the Functions console.
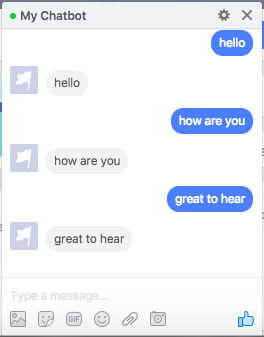
Message your Page to interact with your chatbot.
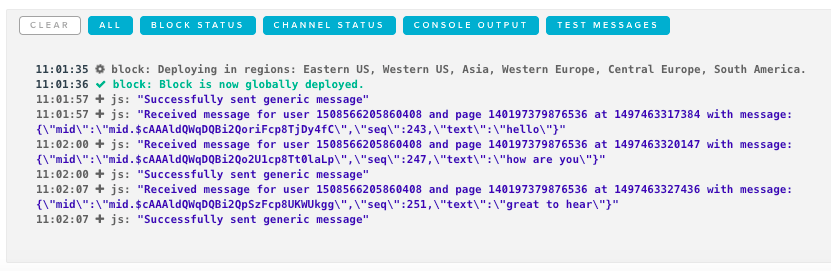
View log output in the Functions console window.
If you want other users to message your chatbot, then you must either add them as Admins to your application, or publish your Page for Facebook review. If accepted, your Page will be public for all users to view and message.
F.) receivedMessage
also sends back other kinds of messages for certain keywords. If you send the message ‘generic’, it will call sendGenericMessage
which sends back a Structured Message with a generic template. Uncomment sendGenericMessage(senderID)
from receivedMessage
in step E.) and add the following function to your event handler:
Facebook’s complete sample app on Github has more examples of sending other types of messages, such as images, gifs, videos, files, etc.
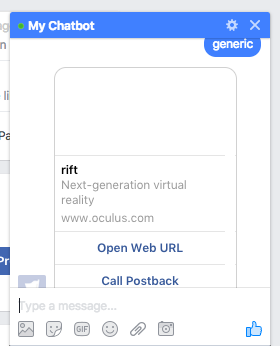
Notice the structured message and the button “Call Postback” which triggers a postback call to your function.
G.) In the last step, the Structured Message that is sent has postbacks. Postbacks are backend calls to your webhook when buttons are clicked. These calls contain the payload
that is set for the button. Buttons on Structured Messages support opening URLs and postbacks.
Comment out receivedPostback(msg)
from the else if statement in step D.) and add the following code to your Function:
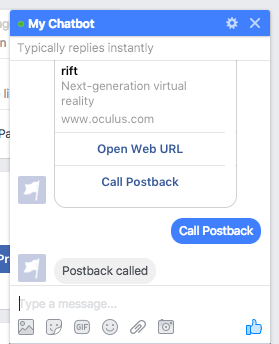
After clicking “Call Postback” a postback is registered.

Functions console output for when a postback is called.
You can view the Function code for the entire tutorial here.
Backed by a Real-time Network
Functions and REST endpoints created with Functions automatically scale with your usage, always run “hot” (no start-up time needed), and are deployed in milliseconds to 15 points of presence (PoPs) worldwide. Each webhook request is then served by the closest point of presence. Functions also has a Key/Value store for use which is replicated to all PoPs as well.
Because your endpoint is deployed on the PubNub network, you have the ability to immediately broadcast to millions of users or devices via publish/subscribe from within your Function. For example, you can configure Github webhooks to trigger your Function anytime an issue for your repo is created or updated. Then from within your Function, you can publish the Github issue information to a channel that your application is subscribed to. Anyone using your application will receive the published message in 250ms or less.
Ideas for Implementation
This tutorial is meant to get you started, providing a skeleton for you to build upon. Here are some ideas for further learning:
- A Messenger/Slack bot that notifies you of icy weather and road conditions in your zipcode. E.g. A Function to receive webhook calls from a 3rd party service (e.g. Weather Underground) and alert users accordingly.
- A Messenger/Slack bot that allows the user to report suspicious activity in their neighborhoods and alert the authorities. E.g. A Function that listens for chat messages reporting suspicious activity and routes them to security personnel that are subscribed to updates on a PubNub channel.
For further learning, check out the Functions and Endpoints documentation. Create a free account to stay in touch with us! We’ll keep you posted as we release more webinars and tutorials in the near future.