How to build a chat app using HTML, CSS, Vue, & JS
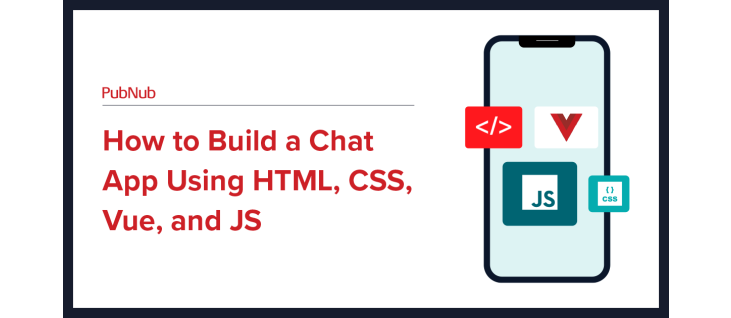
Building a chat application is an effective way to create digital environments where people and devices around the world can easily collaborate, socialize, create new experiences, and exchange information in real time. You’ll need a platform to develop your chat app to provide users with an easy way to connect with one another, regardless of the device they are using. Vue.js is an open-source web framework that is designed to be flexible, lightweight, fast, and able to target not only web apps, but also desktop, mobile, and WebGL applications. With Vue.js, you are able to quickly create responsive user interfaces with its component-based model architecture and use the familiar front-end development languages HTML, CSS, and JavaScript.
According to surveys performed by StackOverflow, including the 2022 survey, Vue.js has consistently ranked in the top ten (including some years being in the top five) most popular web frameworks and technologies used by professional developers, competing with other frameworks such as Angular, React, Laravel, and Node.js (which Vue.js uses in developing applications). Vue.js is designed to be pliable and efficient in creating applications for web developers familiar with HTML, CSS, and JavaScript. Continue reading to learn more about each of these languages and frameworks and how to build a Vue chat app using them.
Why use HTML, CSS, Vue, and JS for your chat app
Using HTML to build a chat app
HTML (HyperText Markup Language) is the language that defines and structures web pages. Since its inception in the early 90s, every underlying web page is built using HTML in some form. HTML is a plaintext document that is rendered in your browser using the DOM (Document Object Model) API that interacts with any HTML file. The DOM is loaded in the browser and is responsible for representing the document in a node tree, where each node is represented by comments and elements. Elements are the individual pieces of a webpage and act as the building blocks for HTML. An element can be as simple as a line of text, to as complex as creating tables or even custom elements.
HTML is essential to develop any application using a web framework like Vue.js. Although you can target multiple platforms with Vue, HTML is still used to create, structure, and set up the user interface in your chat application. You use HTML to set up the different screens in your app, such as the authentication and chat windows. HTML is also used to structure the message input section (chatbox), clickable windows to open users’ friend lists, and a section to show the previous messages.
Using CSS to build a chat app
CSS (Cascading Style Sheets) is the language used to describe elements in web pages. CSS determines how elements should be rendered in web pages, allowing developers to change the properties of elements to fit their application’s unique style. Every single HTML element has CSS properties and can be changed inline (using the style attribute), internally in the same HTML file (in a separate tag), or externally in a separate CSS file (by targeting specific element tags, IDs, and classes, including div ids, and div classes). Although every element may have different properties depending on its type, CSS is used for styling properties such as:
Text: Font style, header styling, size, color, boldness, underlining, etc.
Layout: Setting the position, width, height, color, and size of elements.
Building Blocks: Setting background images and colors, position groups of elements together (the box model).
Interactions: Enabling scroll, mouse cursor, and other user interactions with elements.
CSS would be used in the exact same way as you would on the web to build your chat application. While you build and structure your chat application using HTML, you use CSS to design the look, feel, and compatibility of your app across different platforms. It is used to determine the font style of your application, the color theme (or themes if you allow users to choose), how the windows look and are aligned in relation to other windows, and what elements are interactable on the page. CSS is essential in your application to ensure that your users have not only a good experience, but to ensure that it is recognized amongst the hundreds of other chat apps.
Using Vue to build a chat app
Although you could develop a chat application in vanilla HTML, CSS, and JS, Vue handles a lot of the heavy lifting for your UI when designing user applications such as chat. Vue is based on the component-based programming model, which is similar to object-oriented languages that emphasize the separation of code fragments as templates to be reusable, easier to read, and efficiently test specific areas of code. Vue is more than just a simple JavaScript framework - it can optimize your code for you during compilation and ensures your components do not overrender, wasting computing resources. Vue is a progressive framework, meaning that you can start with a core library and then add the pieces you need as you are developing.
The two most important features of Vue are declarative rendering and reactivity. Declarative rendering is where Vue extends standard HTML that allows users to declaratively describe HTML output based on JavaScript state. This effectively means that developers can declare what they want the resulting HTML output to look like, rather than the process itself. The second important feature, reactivity, is where JavaScript state changes are tracked automatically and update the DOM as changes happen. This means that updates occur in real time, which works well with PubNub’s own real-time communication platform.
Vue.js allows you to create a UI for your chat application that targets multiple platforms. You can build your application in components, which accommodates extensive reusability throughout your application, is easier to maintain, and allows you to replace older components with newer ones as need be, without affecting the data layer. Since Vue is a progressive framework, you can add new functionality to your application as you are developing, so you aren’t forced to use a bloated framework from the beginning. You only need to bring your front-end development knowledge to begin creating the UI in your chat application.
Using JS to build a chat app
JS (JavaScript) is an interpreted programming language that powers the front-end logic of web pages and other browser-based environments. JS is used by developers to drive the logic for users interacting with pages (event handlers), retrieving and sending data, data processing, and even creating and manipulating the HTML and CSS rendered on the page. JS code can be implemented in web pages by adding the code to HTML files inline, internally in a separate script tag, or externally in JS files associated with the project.
For chat applications, JS is used to handle the front-end logic of the application. JS is used when users send messages by clicking on a button, and listen for any updates when receiving new messages from other users to update chat windows. JS is also used to process login information, retrieve previous messages, determine if other users are online, and save user settings. The JavaScript SDK is one of PubNub’s most popular SDKs, powering hundreds of applications. This SDK is how users interact with PubNub’s real-time data APIs, allowing developers to power features such as the communication of chat messages, event listeners to update your application with any changes, and online detection of when users join chat rooms.
How to build a chat app using HTML, CSS, Vue, & JS
To begin building your chat application using HTML, CSS, Vue, and JS, you’ll need to first install any necessary prerequisites. Open your command line interface, such as Bash or Terminal, as you’ll need to run some commands.
Install Node.js and npm
You’ll need to begin by downloading Node.js, the JavaScript runtime environment that is used to build applications. It’s recommended to download the latest, stable version for your operating system.
Included with Node.js is npm (Node Package Manager), which is the package manager for Node.js that allows JavaScript developers to share packaged modules of code.
To test that you have Node.js and npm correctly installed, you can type node --version
and npm --version
in the command line.
Initialize Vue.js
Using the command line, install Vue.js using the following command:
This installs the Vue build tool Vite and you’ll be presented with prompts for several optional features. Press y to install the Vue package, and give your project a name. You can choose no by hitting enter for each option, such as for adding TypeScript. Install any other dependencies required.
Once the project is created, start the dev server by entering the following commands in the command line to navigate to and run your Vue project:
1
This will run your application locally, typically in a browser with a URL such as http://localhost:8080.
Choose Your IDE
The recommended IDE to develop Vue applications is to use Visual Studio Code and install the Vue Language Features (Volar) extension to be able to use features such as syntax highlighting, IntelliSense, and formatting for Vue.js applications.
You can also use other officially supported IDEs.
Develop Your Chat Application
You are now ready to begin developing your chat application. As you are designing your application, you’ll want to make sure you are properly taking advantage of Vue’s unique feature set, including the Vue components that allow developers to split the UI into independent and reusable pieces. It is recommended that you follow Vue’s official guide in creating an application and understanding Vue’s features and functionality.
As you continue to build your application, you’ll need to keep in mind the different types of interactions and features your users require. Users will want to be able to join different servers or organizations that are uniquely created for their hobbies or professional use. They will want to be able to collaborate on different channels or topics, as well as be able to have group chats or even 1:1 conversations with friends and coworkers in private chat rooms. Users should be able to access a member list that shows if their friends or channel members are online. When typing messages, users should be able to see when other users are typing and responding and allow for message reactions and emoji support to keep conversations lively.
Powering these features is by no means an easy task. You have to set up an infrastructure to maintain the communication platform that allows for messages to deliver to users, detect an online presence system, load messages when users come online, and maintain these features securely for your users, on top of building the UI for your chat app. While it is possible to create this infrastructure yourself, this will take time, resources, and upkeep that can be spent elsewhere. This is where PubNub can help.
PubNub is a developer API platform that enables applications to receive real-time updates at a massive, global scale. PubNub serves as the foundation for over 2000 customers in diverse industries. PubNub also partners with other companies to provide unique solutions, such as PubNub and Vonage providing telehealth solutions with voice chat, video chat, or even SMS. Developers can depend on PubNub’s scalability and reliability to power chat features for their applications. PubNub is efficient, reliable, and fast enough to power these features in real-time chat applications, without affecting the user experience.
PubNub has the following features built-in to its API and can meet the specific needs of your application.
Publish: Send updates whenever user input is updated, such as text updates, emoji reactions, sent files, and other complex metadata.
Subscribe: Receive updates to refresh users' screens.
Presence: Update and detect the online status of users.
Message Persistence: Display any missed information to offline users once they log in or track project and document revisions.
Push Notifications: Notify mobile users who are offline about any missed messages, project updates, or application updates.
Objects: Store information about your user in one place without the need of setting up or calling your database.
Access Manager: Restrict access for private conversations, channel rooms, documents, and projects for specific users.
Functions: Translate messages, censor inappropriate messages, announce the arrival of new users, and notify other users of mentions.
Getting Started with PubNub for your chat app
To begin using PubNub to power your Vue.js real-time chat app, you’ll need to first create a PubNub account and download PubNub’s JavaScript SDK. The SDK integrates seamlessly into your application and allows you to connect to PubNub’s real-time communication platform.
Sign in or create an account to create an app on the Admin Portal and get the keys to use in your application. Learn how to do so by following this how-to on creating keys.
Download the JavaScriptSDK by following the instructions in the documentation.
Follow the SDK’s getting started documentation to configure your keys and begin implementing the features to drive your chat functionality.
You can also learn more about how you can enhance your chat application using PubNub with the following resources.
See how other customers depend on PubNub to power their chat applications.
Follow a tour to learn the basics of PubNub.
Interact with our demos, including the Chat in 10 Lines of Code demo, which shows a chat app can be created in less than ten lines of JavaScript code with PubNub.
Learn how to build chat and other industry applications with PubNub.
Follow our step-by-step tutorial to set up and build applications with PubNub, including the Getting Started Tutorial in building a chat app with PubNub’s JavaScriptSDK.
Feel free to reach out to the Developer Relations Team at devrel@pubnub.com for any questions or concerns.