Stream Location Coordinates Data Broadcast with JavaScript
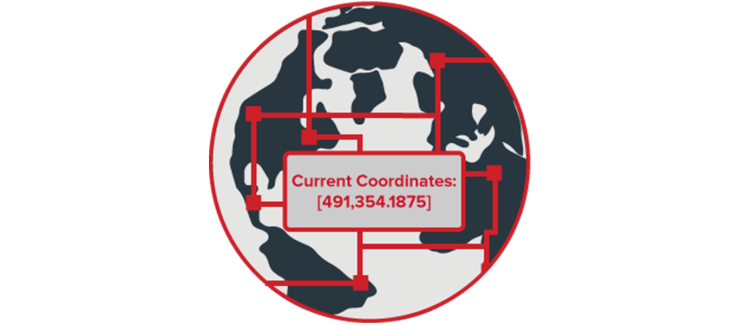
The globe is a physical embodiment of the orthographic project, a tool used in cartography. But what if we want to go digital with this? What if we want to create a virtual simulation of a rotating globe?
In this two part blog series, we’re going to show you how to build an animated D3 map projection with JavaScript (this part) and AngularJS (part two), and stream location coordinates data based on that animated globe.
However, animated JavaScript globes already exist.
We want to take it a step further, and connect the globe projection to the Internet. This a user on the broadcast side to spin the globe, and that movement is reflected in real time on the subscriber side, and coordinates are streamed.
This blog post will show you how to use some of D3.js’s visualization tools and build the broadcaster of coordinates location data. In our second part, we’ll show you how to receive the data and animate the visualization on the subscriber side.
Live working Demo
Want to see it in action? Open the broadcaster and a couple subscribers. As you turn the globe on the broadcaster side, watch as the location is reflected in real time on the subscriber side!
What is a Broadcast Design Pattern?
A broadcast pub/sub messaging design pattern is publishing data from one publisher (broadcaster) to multiple subscribers (listeners) in real time. Updates are sent from the broadcaster, and are received on the subscriber simultaneously. For example, we can build sports statistics streaming apps, or change colors on a webpage in real time.
The design pattern in its simplest form below:
A broadcast design pattern publishes from one broadcaster to multiple subscribers in real time.
So, onto the tutorial!
Creating an Application
The visual medium for this type of tool could be D3.js’s orthographic projection. Check out the interactive orthographic example, provided by Mike Bostock. This example gives us the groundwork to transmit coordinates in real time. Create a file like d3_orthographic_send.html or use the copy provided.
Next you need some data. This is the world-110m.json. Go to this link. Copy everything. Paste it into an empty text file and name it world-110m.json. Direct the d3.json path to your file path. In the example the .html file and the .json are in the same folder.
Once this has been set-up, if you run the file you will be able to spin the globe.
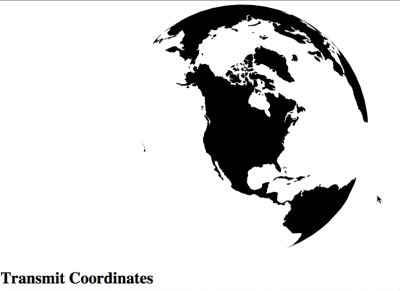
Transmit Coordinates
Next you need to add PubNub and the ability to send the coordinates. Add this line to the script tags at the top to get PubNub’s javascript library working.
At the bottom of our in-line script html you need to initialize PubNub and prepare the publish function.
To get your unique pub/sub keys, you’ll first need to sign up for a PubNub account. Once you sign up, you can get your unique PubNub keys in the PubNub Developer Dashboard. Our free Sandbox tier should give you all the bandwidth you need to build and test your messaging app with the web messaging API.
Notice that you’re publishing messages to the orthoDemo channel. If you created a listener that subscribed to orthoDemo, the listener would be able to hear the messages.
Next you need to go back and have the app call the publish function for the coordinates.
D3 uses a listener for mousemove events over the globe. You take the coordinates, which are provided in a simple array [coord1, coord2]. You then transmit them using the PubNub javascript library and the “pub” method.
Right click the globe, inspect element, and open the console tab. If you begin transmitting you will see a list of coordinates being transmitted. If you were transmitting before you opened this, you will see a list of Array[2] objects.
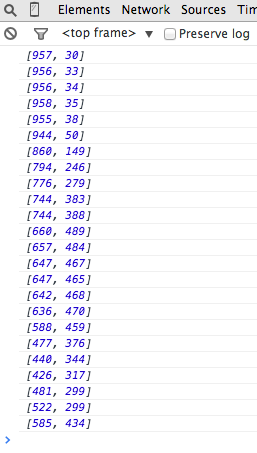
You know it’s working because there are no errors. The console.log, which is printing the coordinates, is in the callback function of the pub method and this means the pub method is being called.
Next Step: Building the Subscriber to Receive the Data
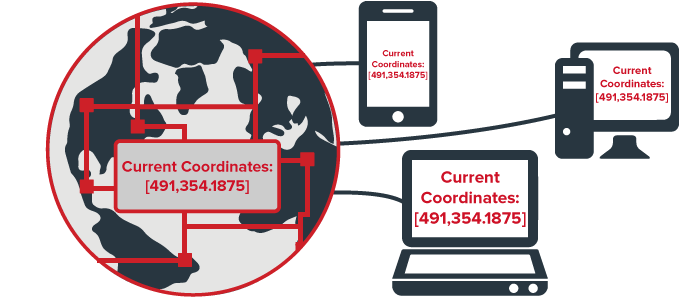
Next we need to receive these coordinates and do something with them. The next entry will discuss the needs and method for creating a receiver. The idea is the receiver takes the coordinates and rotates the globe on its own. For this part of the project it will be necessary to use AngularJS and PubNub’s Angular library. This is in place of the Javascript library used in this example.