Create Microservices and Go Serverless: Functions
The application design model is ever-changing. No longer is creating monolithic applications the standard. We now consider creating microservices in tandem with serverless to be the optimal way of developing, since it offers a better long-term strategy for maintenance and scaling.
Use Cases of Functions for Microservices
eCommerce Platform
A simplified example we can look at is eBay. This isn’t to say that their current infrastructure is built like this, but we can try to imagine how it would look like from a monolithic perspective. eBay in its current setting, to an outsider, looks a bit like this:
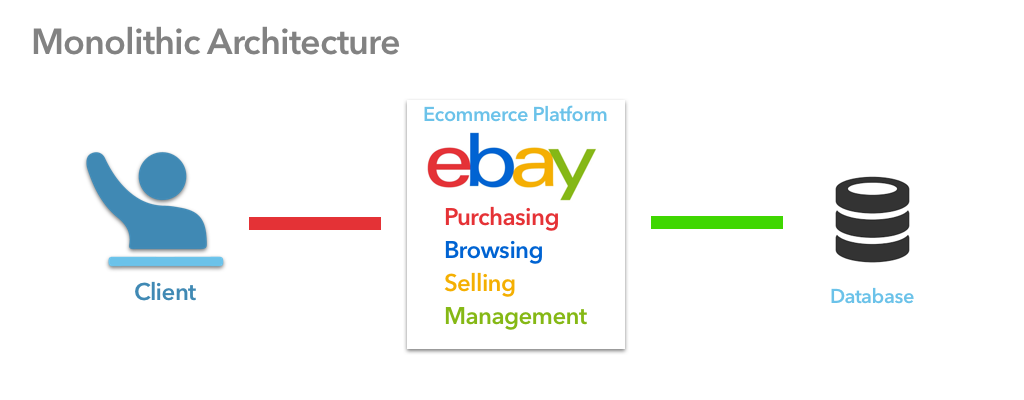
The problem with this sort of infrastructure is that it makes it difficult for applications to scale well. When a company introduces a microservice-based architecture we see that it becomes easier to scale. Keep in mind though, it gets more difficult to manage. We’ll look at how serverless plays a factor in reducing the management hurdles later on.
Functions for a Social Media Platform
The next situation we can look at is a social media platform. Facebook is a suitable example since it provides many services. For example, there is messaging, marketplace, live feed, group and the list goes on. Generally, when a platform launches, it’s quicker to develop in a monolithic style.
However, we can see that as each service gets bigger, they begin to segment into their own services since it’s easier to scale based on the need of each service. In the case of Facebook, the messaging functionality became the messenger app, the groups feature became Facebook groups, and the trend continues as more features grow in scale.
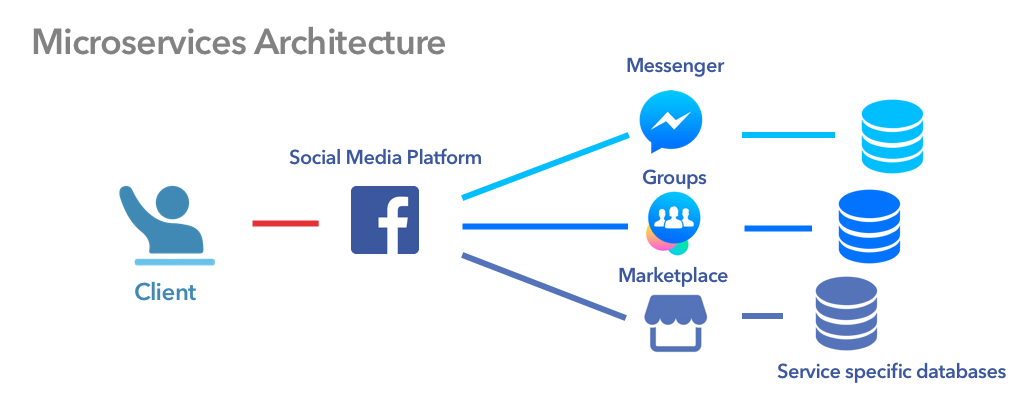
Video Streaming Platform Microservice architecture
We’ve gone through examples that cover both monolithic architecture and one based on microservices, but what would an application look like that was based on serverless microservices?
We can look at Netflix for that. Netflix uses serverless for many functions of their application, ranging from monitoring their data to encoding their media files.
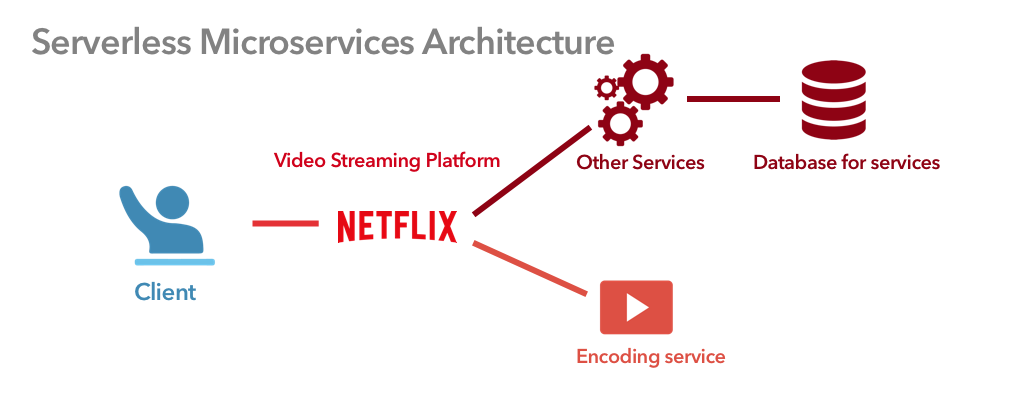
We saw that some of the challenges with microservices is that, even though it may be easier to scale, the management of each specific service becomes more challenging. In the scenario of serverless microservices, this isn’t the case.
Netflix is able to deploy a service onto a serverless platform, similar to Functions, and be able to leave the scaling, routing, and management of the server up to the provider. The benefit of this is that it allows developers to focus on creating a great product rather than juggling the efforts of maintaining a whole infrastructure.
Converting an Existing Service to a Serverless Microservice with PubNub
Start by creating an account at PubNub. From there, let's go ahead and create a new app, then create a key set.
When we’re inside that keyset we can go ahead and click on the Functions icon on the left-hand side and navigate to our modules. In the modules section, we can create a new module which will act as our microservice. In that service, we can make a new event-based block that will deal with the data it’s being sent. In this case, we’ll create a “Before Publish or Fire” function. This will allow us to mutate the data in-transit before it reaches our client.
For more, in-depth details on Functions, I recommend our Crash Course in Functions.
Working with the Data
Out-of-the-box, our Function should look a bit like this:
export default (request) => { const kvstore = require('kvstore'); const xhr = require('xhr'); console.log('request',request); // Log the request envelope passed return request.ok(); // Return a promise when you're done }
You can test the Function by publishing to it from the bottom left in the left-hand side of the dashboard.
In here, we can add our service logic, and whenever a publish occurs to this function we’ll get the altered response back.
Let's create a simple function that takes the message we send and alters it. We can do that by mutating the request.message
object in transit and sending through with the mutation.
export default (request) => { const kvstore = require('kvstore'); const xhr = require('xhr'); request.message.id += 35; console.log('request',request); // Log the request envelope passed return request.ok(); // Return a promise when you're done }
Testing with a Live Application
I’ve set up a website where you can put your publish and subscribe keys and see how your function mutates the data you send in real time. When testing, make sure that your function is operating on the data_channel
channel. This site is completely secure, so feel free to enter your publish/subscribe keys to see how your Function works in actions.