How to Build a JavaScript Simple Chat App with No Backend
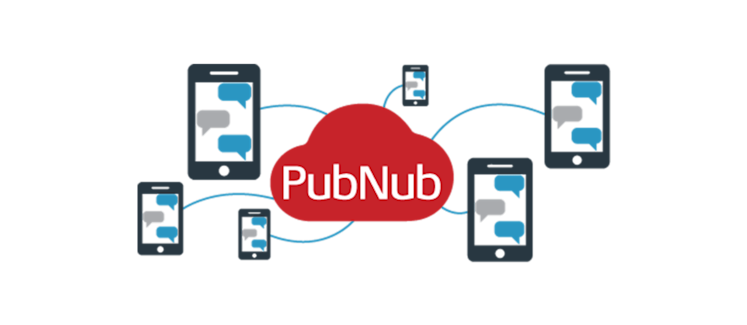
Good News! We’ve launched an all new Chat Resource Center.
We recommend checking out our new Chat Resource Center, which includes overviews, tutorials, and design patterns for building and deploying mobile and web chat.
Trying to plan out and begin building a multi user application can be a pretty tall order but in this part we are going to throw caution to the wind and jump right in. In this post, we’re going to cover:
- Setting up jQuery Mobile and PubNub with a web page
- Creating a basic, simple chat room layout
- Getting communication to and from a single chat room
*This post will assume that you have a basic knowledge of JavaScript and HTML*
This is Part One of our three part tutorial series on how to build a fully-featured JavaScript group chat application with jQuery Mobile and PubNub. Here are the other posts:
- In Action: JavaScript Chat App Demo
- Group Chat App Series Overview
- Part Two: Managing multiple rooms, showing users a history of previous messages, and showing what users are in the room.
- Part Three: Appifying our project through PhoneGap, tweaking the responsive UI, and future improvements.
Installing the Libraries
You’ll first need to sign up for a PubNub account. Once you sign up, you can get your unique PubNub keys in the PubNub Developer Portal.
And now we’re ready to go!
The first thing you have to do is set up a single HTML page with jQuery Mobile and PubNub installed. I highly recommend using the CDN versions for both libraries. This will make download times faster and also scale to millions of users without having to deal with any servers (no need to use any back-end). I also created two files, screen.css and messenger.js, and included them in my page. You can see the <head> section here:
Creating the Simple Chat Page
Now we just need something pretty for the user to look at. Luckily with jQuery Mobile, this is fairly easy to do. The package already comes with several themes built in and allows you to create a page using basic components which is great for building a mobile user interface quickly. Our chat room page will have a list of messages, a send button, and a text box to type in. The code to set this up looks like this:
This page, although with some small styling issues, should suffice for the purposes of this part of the tutorial. In another part of this series, I will talk more about how to get the layout to play nicely and be a little more responsive. Also ignored in this part is a “chat rooms” button that will take the user back to the list of saved chat rooms.
One of the best parts of this user interface is that it is completely cross platform. It works on major desktop browsers and most mobile phones. At PubNub, we look for this kind of out-of-the-box cross platform functionality not only in our personal framework, but also the third party ones we utilize.
Making Magic Happen
Time for the final step: Making the magic happen. This is done by hooking up our PubNub API to our chat interface using JavaScript. Our users will then be able to send messages to a channel and listen for messages from other users. Here’s the code to set this up.
You’ll first need to sign up for a PubNub account. Once you sign up, you can get your unique PubNub keys in the PubNub Developer Portal. Once you have, clone the GitHub repository, and enter your unique PubNub keys on the PubNub initialization, for example:
If you have used the PubNub API before, some of this should look pretty familiar. To communicate with PubNub servers, you need to give the user access using a publish and/or a subscribe key. Be sure to swap in your publish/subscribe keys when testing this out so you can track the data back in our PubNub administration panel.
After that, we use jQuery to grab references to some of our interface elements on the screen. Personally I don’t believe this is a scalable approach to JavaScript development, but for pedagogical reasons this was easier.
The handle message function is what gets called when PubNub gets a real-time message from another user in the channel. It creates a list item element, inserts the message and username, and refreshes the list view which adds all of jQuery Mobile’s fancy list interface and touch capabilities. We can even throw in a scrolling animation for flavor.
Now we get to the message sending. All we do here is read the message the user wrote and make sure they actually typed something, then call publish with PubNub. This is all you have to do and our message is shooting across the internet at light speed (depending your ISP).
Finally we wrap it up by telling PubNub to call handleMessage when we get a message from the chat channel . I also threw in a handler so the user can hit enter instead of clicking on send message. As an application developer, I cannot stress how important it is to throw in shortcuts for your users. Every user has their own way of working (think ‘terminal guru’ vs. ‘grandma’) and it is important to be usable for your entire audience.
That is pretty much it for part one. We setup a simple chat program using PubNub and jQuery Mobile which allows all our users to chat together in one room. If you believe that one room isn’t enough, then be sure to check out the upcoming Part 2 of this series where we will expand on the messenger for multiple chat rooms and more.
Get Started
Sign up for free and use PubNub to power real-time JavaScript chat