Issue Your Own Cryptocurrency with ERC-20 Ethereum Standard
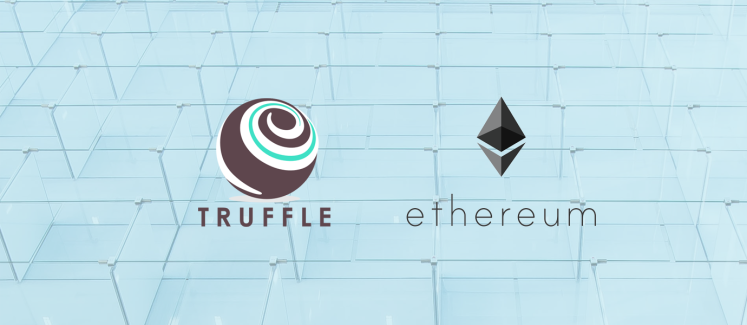
The way we do money is changing. What can you do to keep up? Blockchain and cryptocurrency have been around for years, but only in the past several months has the conversation spread far beyond arcane internet forums and tech company break rooms.
Maybe it’s time for you to make your own cryptocurrency, to make your own medium of exchange for goods and services. That may sound complicated! But it’s simpler than you would expect – thanks to the great strides the decentralization community has made and continues to innovate with.
This is Part One of our three-part series on How To Launch Your Own Production-ready Cryptocurrency. The other parts are:
- Part Two: Write your own integration tests for a Solidity smart contract using JavaScript. A tutorial for deploying Smart contracts and creating a new Ethereum wallet.
- Part Three: How to deploy a cryptocurrency with crowd sale capability to the Ethereum network. Web3.js development and end-to-end testing is demonstrated with the Truffle Suite.
Bank of the Future
A cryptocurrency replaces today’s bank. Banks keep track of the amount of money you have in an account. Instead of trusting a single institution to keep track of this for you, you can trust a massive computer network made up of anyone and everyone to keep track, publicly. The collective computers in this network confirm every single transaction of currency that ever happened and ever will happen. This public consensus is the assurance that people rely on when using cryptocurrency for payment.
Your own cryptocurrency can be the token that you accept for business – kind of like tokens in an arcade. This currency can be brought into existence today. The first step is to choose a big, decentralized computer network that is constantly confirming the legitimacy of new additions to its blockchain. Let’s go with Ethereum.
Smart Contract Programming
Ethereum is a decentralized computing platform for executing smart contracts. These are programs that run code in a transparent environment, without the possibility of third-party tampering. A user’s private key is used to create a fundamentally unique signature for every request that executes a smart contract.
The Ethereum community has a sizable following of open source software engineers that are relentlessly developing amazing tools for the greater good of humanity. To make your own cryptocurrency on the Ethereum network, you need these four tools:
- Solidity – An Ethereum smart contract programming language.
- Truffle Framework – An Ethereum development kit.
- Web3.js – A JavaScript package for interacting with the Ethereum network with an internet browser or Node.js.
- Ether (ETH) – The currency of the Ethereum network.
Optional tools:
- MetaMask – a Chrome extension for crypto payments.
Cryptocurrency is merely one of the limitless use-cases for a blockchain. The brilliant community rallying around Solidity makes it attractive to invest effort in building decentralized apps with Ethereum. First, let’s build your own cryptocurrency to start learning blockchain development.
Developing the ERC-20 Token
The Ethereum community has established some standards regarding the functionality of smart contracts, including tokens. The cryptocurrency token in this tutorial is based on the ERC-20 Token Standard.
If you don’t have node.js, install it now (The tests in part 2 of this guide will not work with versions earlier than node.js 8). Then open a terminal window and do:
npm install -g truffle mkdir MyToken && cd MyToken truffle init npm init -y npm install -E zeppelin-solidity
This installs the Truffle CLI, creates a project directory for your token, and installs an open source Solidity library called OpenZeppelin.
Next, we can begin writing our Solidity contract. Take a few minutes to familiarize yourself with Solidity as well as the DANGERS noted in the Token Standard. In your project directory, create a file in the auto-generated contracts/
folder and name it Token.sol. Add the following code that follows the ERC-20 Token Standard specification.
pragma solidity ^0.4.18; import 'zeppelin-solidity/contracts/math/SafeMath.sol'; /** * Token * * @title A fixed supply ERC-20 token. * https://github.com/ethereum/EIPs/blob/master/EIPS/eip-20.md */ contract Token { using SafeMath for uint; event Transfer( address indexed _from, address indexed _to, uint256 _value ); event Approval( address indexed _owner, address indexed _spender, uint256 _value ); string public symbol; string public name; uint8 public decimals; uint public totalSupply; mapping(address => uint) balances; mapping(address => mapping(address => uint)) allowed; /** * Constructs the Token contract and gives all of the supply to the address * that deployed it. The fixed supply is 1 billion tokens with up to 18 * decimal places. */ function Token() public { symbol = 'TOK'; name = 'Token'; decimals = 18; totalSupply = 1000000000 * 10**uint(decimals); balances[msg.sender] = totalSupply; Transfer(address(0), msg.sender, totalSupply); } /** * @dev Fallback function */ function() public payable { revert(); } /** * Gets the token balance of any wallet. * @param _owner Wallet address of the returned token balance. * @return The balance of tokens in the wallet. */ function balanceOf(address _owner) public constant returns (uint balance) { return balances[_owner]; } /** * Transfers tokens from the sender's wallet to the specified `_to` wallet. * @param _to Address of the transfer's recipient. * @param _value Number of tokens to transfer. * @return True if the transfer succeeded. */ function transfer(address _to, uint _value) public returns (bool success) { balances[msg.sender] = balances[msg.sender].sub(_value); balances[_to] = balances[_to].add(_value); Transfer(msg.sender, _to, _value); return true; } /** * Transfer tokens from any wallet to the `_to` wallet. This only works if * the `_from` wallet has already allocated tokens for the caller wallet * using `approve`. The from wallet must have sufficient balance to * transfer. The caller must have sufficient allowance to transfer. * @param _from Wallet address that tokens are withdrawn from. * @param _to Wallet address that tokens are deposited to. * @param _value Number of tokens transacted. * @return True if the transfer succeeded. */ function transferFrom(address _from, address _to, uint _value) public returns (bool success) { balances[_from] = balances[_from].sub(_value); allowed[_from][msg.sender] = allowed[_from][msg.sender].sub(_value); balances[_to] = balances[_to].add(_value); Transfer(_from, _to, _value); return true; } /** * Sender allows another wallet to `transferFrom` tokens from their wallet. * @param _spender Address of `transferFrom` recipient. * @param _value Number of tokens to `transferFrom`. * @return True if the approval succeeded. */ function approve(address _spender, uint _value) public returns (bool success) { allowed[msg.sender][_spender] = _value; Approval(msg.sender, _spender, _value); return true; } /** * Gets the number of tokens that an `_owner` has approved for a _spender * to `transferFrom`. * @param _owner Wallet address that tokens can be withdrawn from. * @param _spender Wallet address that tokens can be deposited to. * @return The number of tokens allowed to be transferred. */ function allowance(address _owner, address _spender) public constant returns (uint remaining) { return allowed[_owner][_spender]; } }
This token is not mineable and there is a fixed supply. By default, there are 1 billion tokens, and each token can be divided into fractions up to 18 decimal places. The smallest fraction of a token is referred to as Wei in Ethereum or Satoshi in Bitcoin. You can change these numbers, the token name, and the token symbol to whatever you want (see the above constructor function).
Once this code is deployed to the main Ethereum network, anyone who wants to send or receive your token will execute the code in this contract. The balances
map is the data structure that holds all of the information regarding who owns tokens. The address is the wallet owner’s public key, and the unsigned 256 bit integer is the number of token Wei in the wallet.
Read vs. Write
Read and Write operations for the blockchain have two easy rules: reads are free, writes are not. Since we are using the Ethereum network, ETH needs to be spent by the caller when doing blockchain additions. Additions are made by functions that change state, like transferring tokens from one wallet to another. This can be contrasted with read functions, such as viewing the balance of a wallet. Read functions add no new data to the blockchain and they are always free to execute.
Test Your Code
It is very important to thoroughly test the code in your contract before you deploy it. Truffle makes testing easy and fun. Take a look at Part II: Testing and Deploying an Ethereum Token to learn how to write tests, make your Ethereum wallet, and deploy to the main network.