GoInstant-to-PubNub Conceptual Developer Translation Guide
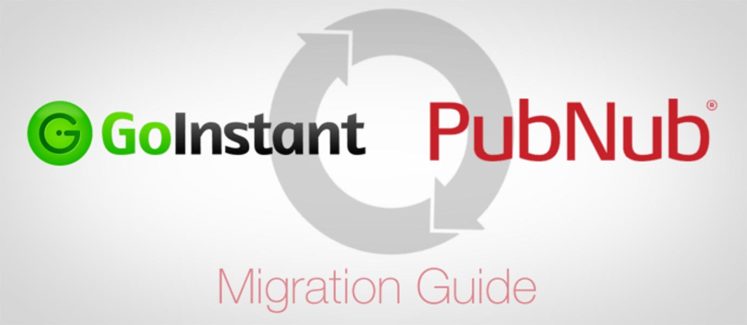
GoInstant-to-PubNub Code Migration Overview
We’re sorry to hear about the discontinuation of GoInstant. PubNub offers comparable features to GoInstant and we’d love to have you migrate to our network. Along with a complete set of migration tools and docs, we are also offering a free month to any GoInstant developer who wishes to migrate to PubNub.
Read below for a general overview of how GoInstant features translate to the PubNub Data Stream Network. The examples below come right from GoInstant’s Getting Started Guide and have been translated to the PubNub SDK.
Note: For GoInstant customers who need PubNub Data Sync, this feature requires both sign up for the GoInstant Migration, and a separate sign-up to access the Private Beta.
Getting Started with Your GoInstant-to-PubNub Migration
Initialization
GoInstant
Connecting to GoInstant gives you access to store data for your application.
<script src="https://cdn.goinstant.net/v1/platform.min.js"></script> <script> var url = 'https://goinstant.net/ACCOUNT/APP'; var connect = goinstant.connect(url); </script>
PubNub
Get a new Pubnub instance with publish and subscribe key. Check out our getting started guide.
<script src="https://cdn.pubnub.com/pubnub.min.js"></script> <script> var pubnub = PUBNUB.init({ publish_key: 'demo', subscribe_key: 'demo' }); </script>
Pub/Sub
GoInstant
When you don’t need to store data, but need to stream super quick messages between users or devices, use Channels. This is available with the Room #channel interface.
var myChannel = room.channel('notifications'); channel.message({ time: Date.now(), message: 'A new user has connected' });
PubNub
The publish() function is used to send a message to all subscribers of a channel. A successfully published message is replicated across the PubNub Data Stream Network and sent simultaneously to all subscribed clients on a channel.
Check out our data streams tutorial.
pubnub.publish({ channel: 'notifications', message: { time: Date.now(), message: 'A new user has connected' } });
Security
GoInstant
Simple security rules for controlling application and data access. In this example all users can read the data inside the person key but only admin users can write.
{ "$room": { "person": { "#read": {"users": ["*"]}, "#write": {"users": [], "groups": ["admin"]} } } }
PubNub
Access Manager provides fine-grain Publish and Subscribe permissions down to person, device, or channel. For more on permissions and authentication, check out our Access Manager tutorial.
// Grant all users on 'privateChat' read access. pubnub.grant({ channel: 'privateChat', read: true, ttl: 60 }); // Grant any user with ```auth_key``` read and write access. pubnub.grant({ channel: 'privateChat', auth_key: 'abxyz12-auth-key-987tuv', read: true, write: true, ttl: 60 });
Storage and Sync
GoInstant
person.get(function(err, value) { // value contains {name: 'John Smith', title: 'CEO'} }); person.on('set', function(value) { // value contains the updated contents of our key });
PubNub
PubNub offers a similar API called Data Sync which is currently in private beta. We’re giving preferred access to developers migrating from GoInstant. Sign up here.
- Automatically sync application data in real time across a range of devices
- Store and share objects throughout your application’s lifecycle
- Support for JavaScript, iOS, Android, Python, Java, and many other environments
- Global synchronization with under 1/4-second latency
- Read/Write access controls permissions on objects across users and devices
- Data encryption via SSL and AES for secure synchonization
API Reference for Migration
Connection
GoInstant
Connect to the GoInstant servers. This will trigger any handlers listening to the connect event.
var url = 'https://goinstant.net/YOURACCOUNT/YOURAPP'; var connection = new goinstant.Connection(url); connection.connect(function(err) { if (err) { console.log('Error connecting to GoInstant:', err); return; } // you're connected! });
PubNub
PubNub connections are made when the client subscribes to a channel.
// Initialize with Publish & Subscribe Keys var pubnub = PUBNUB.init({ publish_key: 'demo', subscribe_key: 'demo' }); // Subscribe with callbacks pubnub.subscribe({ channel : 'my_channel', message : function( message, env, channel ){ // RECEIVED A MESSAGE. console.log(message) }, connect: function(){console.log("Connected")}, disconnect: function(){console.log("Disconnected")}, reconnect: function(){console.log("Reconnected")}, error: function(){console.log("Network Error")}, });
Disconnect
GoInstant
Disconnects from the GoInstant servers. This will trigger any handlers listening to the disconnect event.
connection.disconnect(function(err) { if (err) { console.log("Error disconnecting:", err); return; } // you are disconnected! });
PubNub
When subscribed to a single channel, this function causes the client to issue a leave from the channel and close any open socket to the PubNub Network.
// Unsubscribe from 'my_channel'
pubnub.unsubscribe({
channel : 'my_channel',
});
isGuest
GoInstant
Returns true, false, or null based on if the currently logged-in user is a Guest or not. If the connection is not currently established, null is returned, which in a boolean context is “falsy”.
var url = 'https://goinstant.net/YOURACCOUNT/YOURAPP'; goinstant.connect(url, function(err, conn) {}); if (err) { console.log("Error connecting:", err); // Failed to connect to GoInstant // NOTE: conn.isGuest() will return `null` if called here return; } if (conn.isGuest()) { showLoginButtons(conn); } else { joinRooms(conn); } });
PubNub
The state API is used to get or set key/value pairs specific to a subscriber uuid. A client’s uuid is set during init.
State information is supplied as a JSON object of key/value pairs.
// Initialize with Custom UUID var pubnub = PUBNUB.init({ publish_key: 'demo', subscribe_key: 'demo', uuid: 'my_uuid' }); // Set state to current uuid pubnub.state({ channel : "my_channel", state : { "isGuest": true }, callback : function(m){console.log(m)}, error : function(m){console.log(m)} ); // Get state by uuid. pubnub.state({ channel : "my_channel", uuid : "my_uuid", callback : function(m){console.log(m)}, error : function(m){console.log(m)} );
LoginURL
GoInstant
Returns a URL to the GoInstant Authentication API for the Account & App used to create this connection.
var href = connection.loginUrl('facebook');
PubNub
- Make the Facebook, Twitter, or other OAuth call to identify the user
- Use the information returned as the
auth_key
oruuid
duringPUBNUB.init()
// Facebook Login for Web // https://developers.facebook.com/docs/facebook-login/login-flow-for-web/ FB.api('/me', function(data) { pubnub = PUBNUB.init({ publish_key: 'demo', subscribe_key: 'demo', uuid: data.name }); pubnub.subscribe({ ... }); });
LogoutUrl
GoInstant
Returns a URL to the GoInstant Authentication API that allows a user to “sign out” of the Account & App used to create this connection.
window.location = connection.logoutUrl();
PubNub
Just like our login example, we use FB.logout
then unsubscribe to the channel.
FB.logout(function(response) { pubnub.unsubscribe({ ... }); });
Off
GoInstant
Remove a previously established listener for a Connection event.
connection.off(eventName, callback(errorObject))
PubNub
Because PubNub’s events are registered as callbacks in the PUBNUB.subscribe()
function we do not offer a way to unregister events.
On
GoInstant
Create a listener for a Connection event, which listens for events that are fired locally, and so will never be fired when other users connect/disconnect/error.
connection.on(eventName, listener(errorObject))
PubNub
You can supply callbacks within PUBNUB.subscribe()
for similar events.
pubnub.subscribe({ channel: 'my_channel', message: function( message, env, channel ){ // RECEIVED A MESSAGE. }, connect: function(){console.log("Connected")}, disconnect: function(){console.log("Disconnected")}, reconnect: function(){console.log("Reconnected")}, error: function(){console.log("Network Error")}, });
Room
GoInstant
Returns a Room object.
var connection = new goinstant.Connection(url); connection.connect(token, function (err) { var room = connection.room('YOURROOM'); room.join(function(err) { if (err) { console.log("Error joining room:", err); return; } console.log("Joined room!"); }); });
PubNub
PubNub does not have a concept of rooms, only “channels.” You can get information about channel permissions using audit and information about the users in a channel using here_now. PubNub does not supply a method to find all channels within an application. However, you can find all the channels a uuid is connected to with where_now.