Android Geolocation with the Google Maps API (1/4)
🔔 Before we begin this tutorial, signup for a PubNub account to get your API keys. We've got a generous sandbox tier that'll cost you nothing until it's time to scale! 🔔
Real-time mapping and geolocation tracking is a core feature of web and mobile apps across every industry. The idea is simple – detect and stream location data to a live-updating map to smoothly watch location updates as they change in the real world. With that, Android geolocation tracking is an essential technology today.
Tutorial Overview
This is a 4-part tutorial on building real-time maps for Android using the Google Maps API and PubNub. We'll begin with basics, to get your Android environment set up, then add real-time geolocation functionality in Parts 2-4.
- Part One: Google Maps API and Android Setup (you're here!)
- Part Two: Live Map Markers with Google Maps API
- Part Three: Live Location Tracking with Google Maps API
- Part Four: Flight Paths with Google Maps API
In the end, you'll have a basic Android location tracking app with map markers, device location-tracking, and flight paths, powered by the Google Maps API and PubNub.
This is what our app will look like:
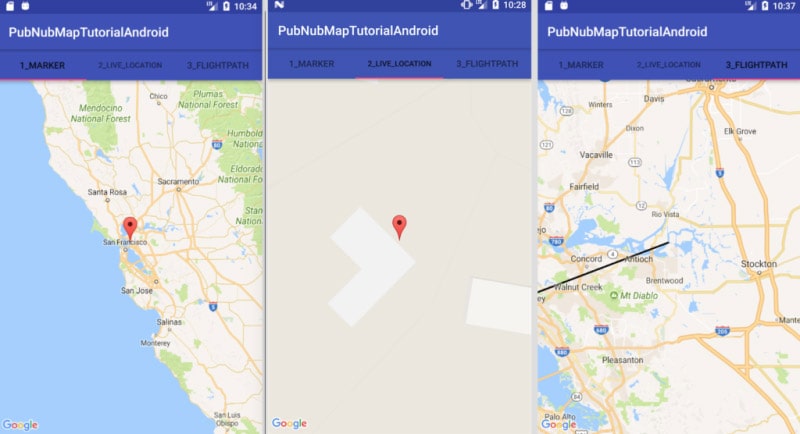
Setup
Tutorial Assets
For this tutorial, we presume you are working with an Android application running Version 23 (Marshmallow) or higher, as this tutorial is intended primarily for mobile devices.
The code for the example used in this tutorial series is available in our GitHub repository. It’s visible via the web UI as well as by using Git to clone the folder to your local machine.
![]() |
Source Code Complete source code is available in our GitHub repository. |
PubNub Developer Keys
To get started, you'll first need your PubNub publish and subscribe keys. If you don't have an account, you can sign up here, and your pub/sub keys are available in the Admin Dashboard. The keys look like UUIDs and start with “pub-c-” and “sub-c-” prefixes respectively.
Once you have your pub/sub keys, create a new PubNub application to represent your Android application (and any other systems that communicate using the same channels). The PubNub application will include publish and subscribe keys that applications can use for those specified features.
Creating your Google Maps API Project and Credentials
To integrate with Google Maps, you’ll need to create a Google Maps API key. To do so, check out this getting started guide.
This creates a unique API key that can be locked down to whatever Android apps you like. You can manage your credentials in the Google API console here.
PubNub Overview
In our Android application, PubNub provides the real-time communication capability that lets our Android application receive incoming events as the position of a remote object (latitude and longitude) changes.
The core PubNub feature we'll use is Real-time Messaging. Our application uses a PubNub channel to send and receive position changed events.
In this sample example, the same application both sends and receives the position events, so consider it is both the publisher and subscriber. But in your application, the publisher (sender) system(s) may be different from the subscriber (receiving) system(s).
Google Maps API Overview
The Google Maps API provides an Android Map widget that displays a map based on your configuration. The map size, map center latitude and longitude, zoom level, map style and other options may be configured to your initial preferences and updated on the fly.
It also provides an initialization callback option that calls a function you specify when the map is loading. You can use features like Map Markers and Polylines which allow you to place friendly markers and flight paths on the map at the location(s) you specify. All the points are user-specified and may be updated in real time as updates arrive.
Google Maps provides great mapping functionality, and combined with PubNub for the real-time location coordinates updates, the two work incredibly well together.
Working with the Code
First, clone the code onto your local machine using Git or the import project feature of Android Studio. Once the project is imported, you’ll be able to modify the files using the IDE like this:
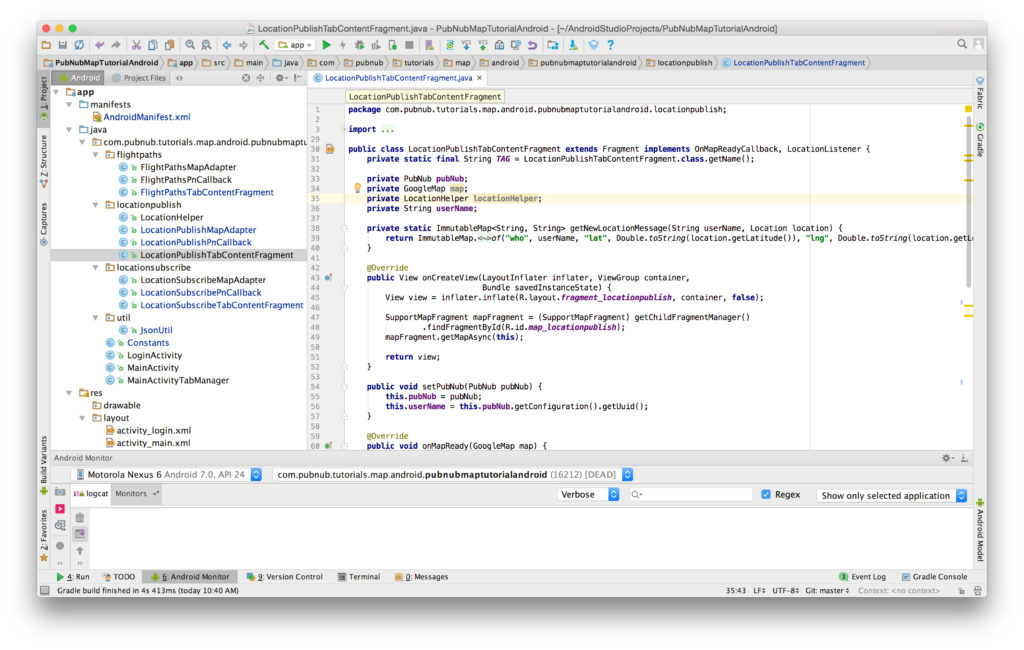
Once you’re in the IDE, you’ll be able to perform the minor code changes to get the app running quickly.
Application Setup and Configuration
This code structure should be familiar if you’ve worked with Android applications in the past. We start with a plain Android application that has two activities:
- LoginActivity, which simply collects a username
- MainActivity, that contains a tabbed view for all of our real-time location features.
We include the PubNub library for real-time communications in the build.gradle file.
compile group: 'com.pubnub', name: 'pubnub-gson', version: '4.12.0'
You’ll also want to include the Google Maps API via Play Services. At the time of writing, the relevant version is 11.0.4.
compile 'com.google.android.gms:play-services:11.0.4'
There are a few key permission settings you’ll need to set up in your AndroidManifest.xml.
<uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/> <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
While you’re in there, you’ll also need to update the Google Maps API key you set up earlier:
<meta-data android:name="com.google.android.geo.API_KEY" android:value="YOUR_MAPS_KEY"/>
We’ve taken the approach of encapsulating common settings into a Constants.java file – for example, the PubNub publish and subscribe keys mentioned earlier in this tutorial.
public class Constants { public static final String PUBNUB_PUBLISH_KEY = "YOUR_PUB_KEY"; public static final String PUBNUB_SUBSCRIBE_KEY = "YOUR_SUB_KEY"; ... }
Running the Code
To run the code, you just need to push the green “play” button in the IDE. That will bring up the Android device chooser so you can choose where to run the app. We typically run our apps using emulation first since it’s quicker.
Once you get the hang of that, it’s easy to connect an Android device via USB for live debugging. This is especially handy for testing live location tracking, which isn't as practical in the emulator.
Next Steps
With that, we now have our app set up. In Part Two, we'll implement live map markers, which identify where a device is located on a map.