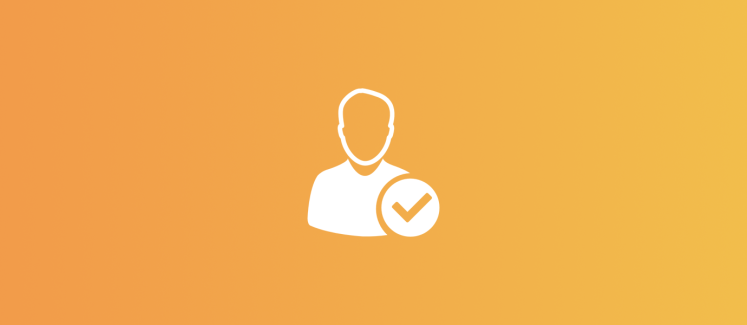
Functions allow a developer to deploy a service that is infinitely scalable in just a few minutes. All you need is a PubNub account, a little bit of Node.js knowledge along with request and response code ability, and you’re ready to begin writing code.
Token Validation
Web applications that use authentication need to have a pattern implemented to make sure that every request a user makes is valid and authentic. A common method of verifying identity is keeping a token in your back-end database, and issuing it only when a user has properly provided their secret credentials.
Every time the authenticated user makes a subsequent request to your application, they must provide this token, which only their device and your database know. We can use Functions as our back-end application and database, thanks to Node.js and the Vault module.
Functions
Functions execute on an in-transit PubNub message, allowing the developer to do some magic in the middle. The On Request event handler turns Functions into your own HTTP server that handles a basic task. You can use the architectural term: microservice.
In this instance, we are storing a secret token, one that we wish to hide in the cloud. Let’s say we need to create a service that validates a client’s token, to make sure they are using the right one. This very simple demo shows how to build and deploy this service in under 5 minutes.
Check out the Functions quickstart tutorial to learn how to submit your code with the deploy button in the PubNub Admin Dashboard.
export default (request, response) => { const vault = require('vault'); let paramsObject = request.params; return vault.get('myToken').then((token) => { if (paramsObject.token === token) { response.status = 200; return response.send(); } else { response.status = 400; return response.send(); } }).catch((err) => { console.error(err); response.status = 500; return response.send(); }); };
Above we have the event handler code to verify a user’s token on request. Before we deploy the code, we should set the secret token inside of Functions dev environment. These are keys and values that are kept safe and secure, and can only be accessed from the event handler code using Vault.
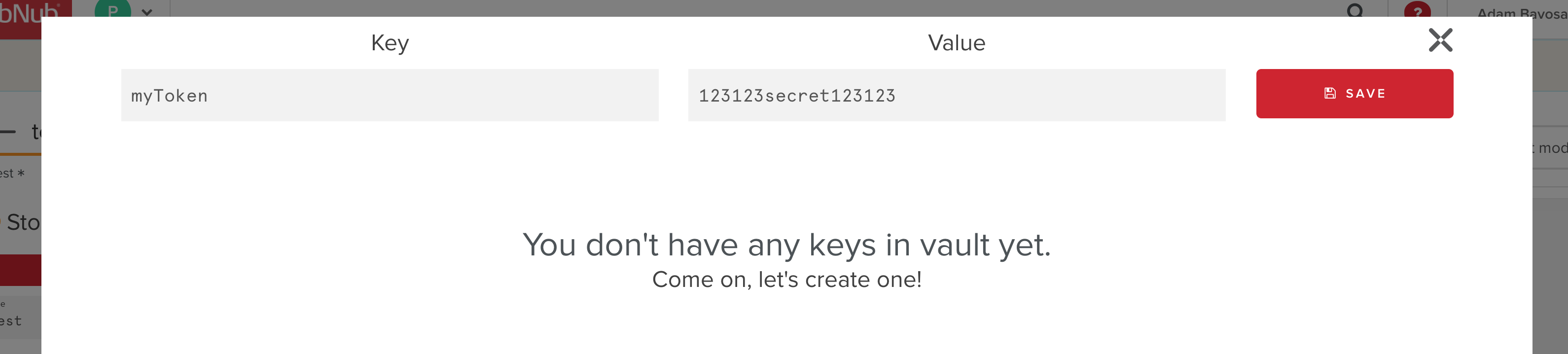
Click on the My Secrets button on the left-hand side of the window and input your key and value for your token.
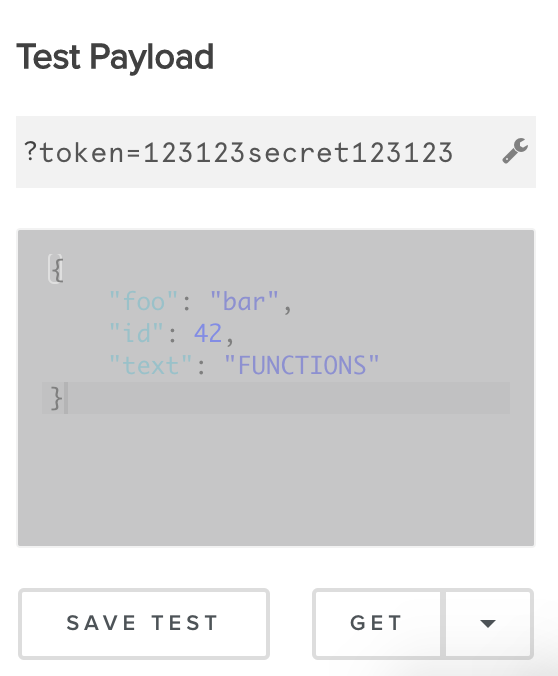
Next, we can use the Test Payload with the proper URI to check that our service sends the correct response codes. Copy the URL for the server using the COPY URL Button on the left side of the functions dev environment.
Hit the play button on the right to deploy this service globally in a few seconds.
We can use the following HTML page with the simple UI to represent our robust and dignified web application. Be sure to paste the On Request URL from the Functions Dev Environment into your JavaScript (COPY URL button on the left).
<!DOCTYPE html> <html> <head> <title>Token Validator</title> <link rel="stylesheet" type="text/css" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css"> </head> <body> <input id="text" type="text"> <input id="submit" type="submit"> <p id="serverResponse"></p> </body> <script src="https://cdn.pubnub.com/sdk/javascript/pubnub.4.20.2.js"></script> <script type="text/javascript"> var text = document.getElementById('text'); var submit = document.getElementById('submit'); var serverResponse = document.getElementById('serverResponse'); var onRequestUri = "__PubNub_Functions_URI__"; function reqListener () { serverResponse.innerHTML = this.status; } submit.addEventListener("click", function() { var req = new XMLHttpRequest(); req.addEventListener("load", reqListener); req.open("GET", onRequestUri + "?token=" + text.value); req.send(); }); </script> </html>
Now we can confirm that our serverless app works. Input a correct or incorrect token into the textbox and hit submit. The response status code will be displayed below the textbox.
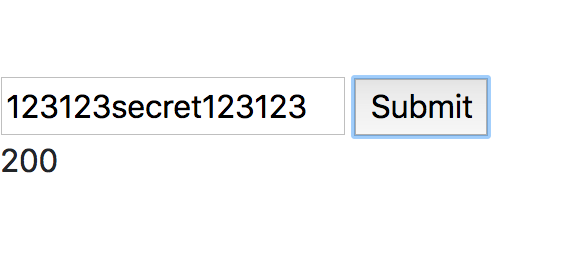
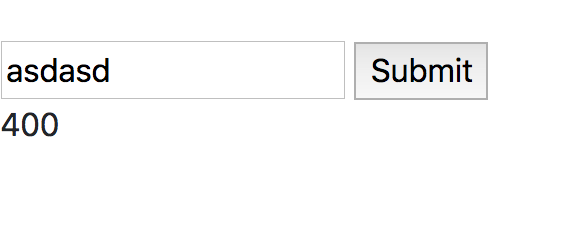
This example does not provide any complex algorithms for verifying tokens however this can be done using the crypto module in Functions.